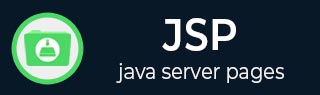
- Basic JSP Tutorial
- JSP - Home
- JSP - Overview
- JSP - Environment Setup
- JSP - Architecture
- JSP - Lifecycle
- JSP - Syntax
- JSP - Directives
- JSP - Actions
- JSP - Implicit Objects
- JSP - Client Request
- JSP - Server Response
- JSP - Http Status Codes
- JSP - Form Processing
- JSP - Writing Filters
- JSP - Cookies Handling
- JSP - Session Tracking
- JSP - File Uploading
- JSP - Handling Date
- JSP - Page Redirect
- JSP - Hits Counter
- JSP - Auto Refresh
- JSP - Sending Email
- Advanced JSP Tutorials
- JSP - Standard Tag Library
- JSP - Database Access
- JSP - XML Data
- JSP - Java Beans
- JSP - Custom Tags
- JSP - Expression Language
- JSP - Exception Handling
- JSP - Debugging
- JSP - Security
- JSP - Internationalization
- JSP Useful Resources
- JSP - Questions and Answers
- JSP - Quick Guide
- JSP - Useful Resources
- JSP - Discussion
JSP - Sending Email
In this chapter, we will discuss how to send emails using JSP. To send an email using a JSP, you should have the JavaMail API and the Java Activation Framework (JAF) installed on your machine.
You can download the latest version of JavaMail (Version 1.2) from the Java's standard website.
You can download the latest version of JavaBeans Activation Framework JAF (Version 1.0.2) from the Java's standard website.
Download and unzip these files, in the newly-created top-level directories. You will find a number of jar files for both the applications. You need to add the mail.jar and the activation.jar files in your CLASSPATH.
Send a Simple Email
Here is an example to send a simple email from your machine. It is assumed that your localhost is connected to the Internet and that it is capable enough to send an email. Make sure all the jar files from the Java Email API package and the JAF package are available in CLASSPATH.
<%@ page import = "java.io.*,java.util.*,javax.mail.*"%> <%@ page import = "javax.mail.internet.*,javax.activation.*"%> <%@ page import = "javax.servlet.http.*,javax.servlet.*" %> <% String result; // Recipient's email ID needs to be mentioned. String to = "abcd@gmail.com"; // Sender's email ID needs to be mentioned String from = "mcmohd@gmail.com"; // Assuming you are sending email from localhost String host = "localhost"; // Get system properties object Properties properties = System.getProperties(); // Setup mail server properties.setProperty("mail.smtp.host", host); // Get the default Session object. Session mailSession = Session.getDefaultInstance(properties); try { // Create a default MimeMessage object. MimeMessage message = new MimeMessage(mailSession); // Set From: header field of the header. message.setFrom(new InternetAddress(from)); // Set To: header field of the header. message.addRecipient(Message.RecipientType.TO, new InternetAddress(to)); // Set Subject: header field message.setSubject("This is the Subject Line!"); // Now set the actual message message.setText("This is actual message"); // Send message Transport.send(message); result = "Sent message successfully...."; } catch (MessagingException mex) { mex.printStackTrace(); result = "Error: unable to send message...."; } %> <html> <head> <title>Send Email using JSP</title> </head> <body> <center> <h1>Send Email using JSP</h1> </center> <p align = "center"> <% out.println("Result: " + result + "\n"); %> </p> </body> </html>
Let us now put the above code in SendEmail.jsp file and call this JSP using the URL http://localhost:8080/SendEmail.jsp. This will help send an email to the given email ID abcd@gmail.com. You will receive the following response −
Send Email using JSP
Result: Sent message successfully....
If you want to send an email to multiple recipients, then use the following methods to specify multiple email IDs −
void addRecipients(Message.RecipientType type, Address[] addresses) throws MessagingException
Here is the description of the parameters −
type − This would be set to TO, CC or BCC. Here CC represents Carbon Copy and BCC represents Black Carbon Copy. Example Message.RecipientType.TO
addresses − This is the array of email ID. You would need to use the InternetAddress() method while specifying email IDs
Send an HTML Email
Here is an example to send an HTML email from your machine. It is assumed that your localhost is connected to the Internet and that it is capable enough to send an email. Make sure all the jar files from the Java Email API package and the JAF package are available in CLASSPATH.
This example is very similar to the previous one, except that here we are using the setContent() method to set content whose second argument is "text/html" to specify that the HTML content is included in the message.
Using this example, you can send as big an HTML content as you require.
<%@ page import = "java.io.*,java.util.*,javax.mail.*"%> <%@ page import = "javax.mail.internet.*,javax.activation.*"%> <%@ page import = "javax.servlet.http.*,javax.servlet.*" %> <% String result; // Recipient's email ID needs to be mentioned. String to = "abcd@gmail.com"; // Sender's email ID needs to be mentioned String from = "mcmohd@gmail.com"; // Assuming you are sending email from localhost String host = "localhost"; // Get system properties object Properties properties = System.getProperties(); // Setup mail server properties.setProperty("mail.smtp.host", host); // Get the default Session object. Session mailSession = Session.getDefaultInstance(properties); try { // Create a default MimeMessage object. MimeMessage message = new MimeMessage(mailSession); // Set From: header field of the header. message.setFrom(new InternetAddress(from)); // Set To: header field of the header. message.addRecipient(Message.RecipientType.TO, new InternetAddress(to)); // Set Subject: header field message.setSubject("This is the Subject Line!"); // Send the actual HTML message, as big as you like message.setContent("<h1>This is actual message</h1>", "text/html" ); // Send message Transport.send(message); result = "Sent message successfully...."; } catch (MessagingException mex) { mex.printStackTrace(); result = "Error: unable to send message...."; } %> <html> <head> <title>Send HTML Email using JSP</title> </head> <body> <center> <h1>Send Email using JSP</h1> </center> <p align = "center"> <% out.println("Result: " + result + "\n"); %> </p> </body> </html>
Let us now use the above JSP to send HTML message on a given email ID.
Send Attachment in Email
Following is an example to send an email with attachment from your machine −
<%@ page import = "java.io.*,java.util.*,javax.mail.*"%> <%@ page import = "javax.mail.internet.*,javax.activation.*"%> <%@ page import = "javax.servlet.http.*,javax.servlet.*" %> <% String result; // Recipient's email ID needs to be mentioned. String to = "abcd@gmail.com"; // Sender's email ID needs to be mentioned String from = "mcmohd@gmail.com"; // Assuming you are sending email from localhost String host = "localhost"; // Get system properties object Properties properties = System.getProperties(); // Setup mail server properties.setProperty("mail.smtp.host", host); // Get the default Session object. Session mailSession = Session.getDefaultInstance(properties); try { // Create a default MimeMessage object. MimeMessage message = new MimeMessage(mailSession); // Set From: header field of the header. message.setFrom(new InternetAddress(from)); // Set To: header field of the header. message.addRecipient(Message.RecipientType.TO, new InternetAddress(to)); // Set Subject: header field message.setSubject("This is the Subject Line!"); // Create the message part BodyPart messageBodyPart = new MimeBodyPart(); // Fill the message messageBodyPart.setText("This is message body"); // Create a multipart message Multipart multipart = new MimeMultipart(); // Set text message part multipart.addBodyPart(messageBodyPart); // Part two is attachment messageBodyPart = new MimeBodyPart(); String filename = "file.txt"; DataSource source = new FileDataSource(filename); messageBodyPart.setDataHandler(new DataHandler(source)); messageBodyPart.setFileName(filename); multipart.addBodyPart(messageBodyPart); // Send the complete message parts message.setContent(multipart ); // Send message Transport.send(message); String title = "Send Email"; result = "Sent message successfully...."; } catch (MessagingException mex) { mex.printStackTrace(); result = "Error: unable to send message...."; } %> <html> <head> <title>Send Attachment Email using JSP</title> </head> <body> <center> <h1>Send Attachment Email using JSP</h1> </center> <p align = "center"> <%out.println("Result: " + result + "\n");%> </p> </body> </html>
Let us now run the above JSP to send a file as an attachment along with a message on a given email ID.
User Authentication Part
If it is required to provide user ID and Password to the email server for authentication purpose, then you can set these properties as follows −
props.setProperty("mail.user", "myuser"); props.setProperty("mail.password", "mypwd");
Rest of the email sending mechanism will remain as explained above.
Using Forms to Send Email
You can use HTML form to accept email parameters and then you can use the request object to get all the information as follows −
String to = request.getParameter("to"); String from = request.getParameter("from"); String subject = request.getParameter("subject"); String messageText = request.getParameter("body");
Once you have all the information, you can use the above mentioned programs to send email.