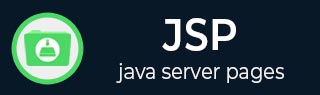
- Basic JSP Tutorial
- JSP - Home
- JSP - Overview
- JSP - Environment Setup
- JSP - Architecture
- JSP - Lifecycle
- JSP - Syntax
- JSP - Directives
- JSP - Actions
- JSP - Implicit Objects
- JSP - Client Request
- JSP - Server Response
- JSP - Http Status Codes
- JSP - Form Processing
- JSP - Writing Filters
- JSP - Cookies Handling
- JSP - Session Tracking
- JSP - File Uploading
- JSP - Handling Date
- JSP - Page Redirect
- JSP - Hits Counter
- JSP - Auto Refresh
- JSP - Sending Email
- Advanced JSP Tutorials
- JSP - Standard Tag Library
- JSP - Database Access
- JSP - XML Data
- JSP - Java Beans
- JSP - Custom Tags
- JSP - Expression Language
- JSP - Exception Handling
- JSP - Debugging
- JSP - Security
- JSP - Internationalization
- JSP Useful Resources
- JSP - Questions and Answers
- JSP - Quick Guide
- JSP - Useful Resources
- JSP - Discussion
JSP - Database Access
In this chapter, we will discuss how to access database with JSP. We assume you have good understanding on how JDBC application works. Before starting with database access through a JSP, make sure you have proper JDBC environment setup along with a database.
For more detail on how to access database using JDBC and its environment setup you can go through our JDBC Tutorial.
To start with basic concept, let us create a table and create a few records in that table as follows −
Create Table
To create the Employees table in the EMP database, use the following steps −
Step 1
Open a Command Prompt and change to the installation directory as follows −
C:\> C:\>cd Program Files\MySQL\bin C:\Program Files\MySQL\bin>
Step 2
Login to the database as follows −
C:\Program Files\MySQL\bin>mysql -u root -p Enter password: ******** mysql>
Step 3
Create the Employee table in the TEST database as follows − −
mysql> use TEST; mysql> create table Employees ( id int not null, age int not null, first varchar (255), last varchar (255) ); Query OK, 0 rows affected (0.08 sec) mysql>
Create Data Records
Let us now create a few records in the Employee table as follows − −
mysql> INSERT INTO Employees VALUES (100, 18, 'Zara', 'Ali'); Query OK, 1 row affected (0.05 sec) mysql> INSERT INTO Employees VALUES (101, 25, 'Mahnaz', 'Fatma'); Query OK, 1 row affected (0.00 sec) mysql> INSERT INTO Employees VALUES (102, 30, 'Zaid', 'Khan'); Query OK, 1 row affected (0.00 sec) mysql> INSERT INTO Employees VALUES (103, 28, 'Sumit', 'Mittal'); Query OK, 1 row affected (0.00 sec) mysql>
SELECT Operation
Following example shows how we can execute the SQL SELECT statement using JTSL in JSP programming −
<%@ page import = "java.io.*,java.util.*,java.sql.*"%> <%@ page import = "javax.servlet.http.*,javax.servlet.*" %> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix = "c"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/sql" prefix = "sql"%> <html> <head> <title>SELECT Operation</title> </head> <body> <sql:setDataSource var = "snapshot" driver = "com.mysql.jdbc.Driver" url = "jdbc:mysql://localhost/TEST" user = "root" password = "pass123"/> <sql:query dataSource = "${snapshot}" var = "result"> SELECT * from Employees; </sql:query> <table border = "1" width = "100%"> <tr> <th>Emp ID</th> <th>First Name</th> <th>Last Name</th> <th>Age</th> </tr> <c:forEach var = "row" items = "${result.rows}"> <tr> <td><c:out value = "${row.id}"/></td> <td><c:out value = "${row.first}"/></td> <td><c:out value = "${row.last}"/></td> <td><c:out value = "${row.age}"/></td> </tr> </c:forEach> </table> </body> </html>
Access the above JSP, the following result will be displayed −
Emp ID First Name Last Name Age 100 Zara Ali 18 101 Mahnaz Fatma 25 102 Zaid Khan 30 103 Sumit Mittal 28
INSERT Operation
Following example shows how we can execute the SQL INSERT statement using JTSL in JSP programming −
<%@ page import = "java.io.*,java.util.*,java.sql.*"%> <%@ page import = "javax.servlet.http.*,javax.servlet.*" %> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix = "c"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/sql" prefix = "sql"%> <html> <head> <title>JINSERT Operation</title> </head> <body> <sql:setDataSource var = "snapshot" driver = "com.mysql.jdbc.Driver" url = "jdbc:mysql://localhost/TEST" user = "root" password = "pass123"/> <sql:update dataSource = "${snapshot}" var = "result"> INSERT INTO Employees VALUES (104, 2, 'Nuha', 'Ali'); </sql:update> <sql:query dataSource = "${snapshot}" var = "result"> SELECT * from Employees; </sql:query> <table border = "1" width = "100%"> <tr> <th>Emp ID</th> <th>First Name</th> <th>Last Name</th> <th>Age</th> </tr> <c:forEach var = "row" items = "${result.rows}"> <tr> <td><c:out value = "${row.id}"/></td> <td><c:out value = "${row.first}"/></td> <td><c:out value = "${row.last}"/></td> <td><c:out value = "${row.age}"/></td> </tr> </c:forEach> </table> </body> </html>
Access the above JSP, the following result will be displayed −
Emp ID First Name Last Name Age 100 Zara Ali 18 101 Mahnaz Fatma 25 102 Zaid Khan 30 103 Sumit Mittal 28 104 Nuha Ali 2
DELETE Operation
Following example shows how we can execute the SQL DELETE statement using JTSL in JSP programming −
<%@ page import = "java.io.*,java.util.*,java.sql.*"%> <%@ page import = "javax.servlet.http.*,javax.servlet.*" %> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix = "c"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/sql" prefix = "sql"%> <html> <head> <title>DELETE Operation</title> </head> <body> <sql:setDataSource var = "snapshot" driver = "com.mysql.jdbc.Driver" url = "jdbc:mysql://localhost/TEST" user = "root" password = "pass123"/> <c:set var = "empId" value = "103"/> <sql:update dataSource = "${snapshot}" var = "count"> DELETE FROM Employees WHERE Id = ? <sql:param value = "${empId}" /> </sql:update> <sql:query dataSource = "${snapshot}" var = "result"> SELECT * from Employees; </sql:query> <table border = "1" width = "100%"> <tr> <th>Emp ID</th> <th>First Name</th> <th>Last Name</th> <th>Age</th> </tr> <c:forEach var = "row" items = "${result.rows}"> <tr> <td><c:out value = "${row.id}"/></td> <td><c:out value = "${row.first}"/></td> <td><c:out value = "${row.last}"/></td> <td><c:out value = "${row.age}"/></td> </tr> </c:forEach> </table> </body> </html>
Access the above JSP, the following result will be displayed −
Emp ID First Name Last Name Age 100 Zara Ali 18 101 Mahnaz Fatma 25 102 Zaid Khan 30
UPDATE Operation
Following example shows how we can execute the SQL UPDATE statement using JTSL in JSP programming −
<%@ page import = "java.io.*,java.util.*,java.sql.*"%> <%@ page import = "javax.servlet.http.*,javax.servlet.*" %> <%@ taglib uri = "http://java.sun.com/jsp/jstl/core" prefix = "c"%> <%@ taglib uri = "http://java.sun.com/jsp/jstl/sql" prefix = "sql"%> <html> <head> <title>DELETE Operation</title> </head> <body> <sql:setDataSource var = "snapshot" driver = "com.mysql.jdbc.Driver" url = "jdbc:mysql://localhost/TEST" user = "root" password = "pass123"/> <c:set var = "empId" value = "102"/> <sql:update dataSource = "${snapshot}" var = "count"> UPDATE Employees SET WHERE last = 'Ali' <sql:param value = "${empId}" /> </sql:update> <sql:query dataSource = "${snapshot}" var = "result"> SELECT * from Employees; </sql:query> <table border = "1" width = "100%"> <tr> <th>Emp ID</th> <th>First Name</th> <th>Last Name</th> <th>Age</th> </tr> <c:forEach var = "row" items = "${result.rows}"> <tr> <td><c:out value = "${row.id}"/></td> <td><c:out value = "${row.first}"/></td> <td><c:out value = "${row.last}"/></td> <td><c:out value = "${row.age}"/></td> </tr> </c:forEach> </table> </body> </html>
Access the above JSP, the following result will be displayed −
Emp ID First Name Last Name Age 100 Zara Ali 18 101 Mahnaz Fatma 25 102 Zaid Ali 30