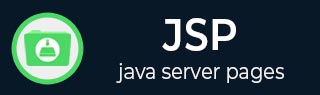
- Basic JSP Tutorial
- JSP - Home
- JSP - Overview
- JSP - Environment Setup
- JSP - Architecture
- JSP - Lifecycle
- JSP - Syntax
- JSP - Directives
- JSP - Actions
- JSP - Implicit Objects
- JSP - Client Request
- JSP - Server Response
- JSP - Http Status Codes
- JSP - Form Processing
- JSP - Writing Filters
- JSP - Cookies Handling
- JSP - Session Tracking
- JSP - File Uploading
- JSP - Handling Date
- JSP - Page Redirect
- JSP - Hits Counter
- JSP - Auto Refresh
- JSP - Sending Email
- Advanced JSP Tutorials
- JSP - Standard Tag Library
- JSP - Database Access
- JSP - XML Data
- JSP - Java Beans
- JSP - Custom Tags
- JSP - Expression Language
- JSP - Exception Handling
- JSP - Debugging
- JSP - Security
- JSP - Internationalization
- JSP Useful Resources
- JSP - Questions and Answers
- JSP - Quick Guide
- JSP - Useful Resources
- JSP - Discussion
JSP - Auto Refresh
In this chapter, we will discuss Auto Refresh in JSP. Consider a webpage which is displaying live game score or stock market status or currency exchange ration. For all such type of pages, you would need to refresh your Webpage regularly using refresh or reload button with your browser.
JSP makes this job easy by providing you a mechanism where you can make a webpage in such a way that it would refresh automatically after a given interval.
The simplest way of refreshing a Webpage is by using the setIntHeader() method of the response object. Following is the signature of this method −
public void setIntHeader(String header, int headerValue)
This method sends back the header "Refresh" to the browser along with an integer value which indicates time interval in seconds.
Auto Page Refresh Example
In the following example, we will use the setIntHeader() method to set Refresh header. This will help simulate a digital clock −
<%@ page import = "java.io.*,java.util.*" %> <html> <head> <title>Auto Refresh Header Example</title> </head> <body> <center> <h2>Auto Refresh Header Example</h2> <% // Set refresh, autoload time as 5 seconds response.setIntHeader("Refresh", 5); // Get current time Calendar calendar = new GregorianCalendar(); String am_pm; int hour = calendar.get(Calendar.HOUR); int minute = calendar.get(Calendar.MINUTE); int second = calendar.get(Calendar.SECOND); if(calendar.get(Calendar.AM_PM) == 0) am_pm = "AM"; else am_pm = "PM"; String CT = hour+":"+ minute +":"+ second +" "+ am_pm; out.println("Crrent Time: " + CT + "\n"); %> </center> </body> </html>
Now put the above code in main.jsp and try to access it. This will display the current system time after every 5 seconds as follows. Just run the JSP and wait to see the result −
Auto Refresh Header Example
Current Time is: 9:44:50 PM