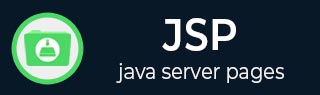
- Basic JSP Tutorial
- JSP - Home
- JSP - Overview
- JSP - Environment Setup
- JSP - Architecture
- JSP - Lifecycle
- JSP - Syntax
- JSP - Directives
- JSP - Actions
- JSP - Implicit Objects
- JSP - Client Request
- JSP - Server Response
- JSP - Http Status Codes
- JSP - Form Processing
- JSP - Writing Filters
- JSP - Cookies Handling
- JSP - Session Tracking
- JSP - File Uploading
- JSP - Handling Date
- JSP - Page Redirect
- JSP - Hits Counter
- JSP - Auto Refresh
- JSP - Sending Email
- Advanced JSP Tutorials
- JSP - Standard Tag Library
- JSP - Database Access
- JSP - XML Data
- JSP - Java Beans
- JSP - Custom Tags
- JSP - Expression Language
- JSP - Exception Handling
- JSP - Debugging
- JSP - Security
- JSP - Internationalization
- JSP Useful Resources
- JSP - Questions and Answers
- JSP - Quick Guide
- JSP - Useful Resources
- JSP - Discussion
JSP - Filters
In this chapter, we will discuss Filters in JSP. Servlet and JSP Filters are Java classes that can be used in Servlet and JSP Programming for the following purposes −
To intercept requests from a client before they access a resource at back end.
To manipulate responses from server before they are sent back to the client.
There are various types of filters suggested by the specifications −
- Authentication Filters
- Data compression Filters
- Encryption Filters
- Filters that trigger resource access events
- Image Conversion Filters
- Logging and Auditing Filters
- MIME-TYPE Chain Filters
- Tokenizing Filters
- XSL/T Filters That Transform XML Content
Filters are deployed in the deployment descriptor file web.xml and then map to either servlet or JSP names or URL patterns in your application's deployment descriptor. The deployment descriptor file web.xml can be found in <Tomcat-installation-directory>\conf directory.
When the JSP container starts up your web application, it creates an instance of each filter that you have declared in the deployment descriptor. The filters execute in the order that they are declared in the deployment descriptor.
Servlet Filter Methods
A filter is simply a Java class that implements the javax.servlet.Filter interface. The javax.servlet.Filter interface defines three methods −
S.No. | Method & Description |
---|---|
1 | public void doFilter (ServletRequest, ServletResponse, FilterChain) This method is called by the container each time a request/response pair is passed through the chain due to a client request for a resource at the end of the chain. |
2 | public void init(FilterConfig filterConfig) This method is called by the web container to indicate to a filter that it is being placed into service. |
3 | public void destroy() This method is called by the web container to indicate to a filter that it is being taken out of service. |
JSP Filter Example
Following example shows how to print the client’s IP address and the current date time, each time it would access any JSP file. This example will give you a basic understanding of the JSP Filter, but you can write more sophisticated filter applications using the same concept −
// Import required java libraries import java.io.*; import javax.servlet.*; import javax.servlet.http.*; import java.util.*; // Implements Filter class public class LogFilter implements Filter { public void init(FilterConfig config) throws ServletException { // Get init parameter String testParam = config.getInitParameter("test-param"); //Print the init parameter System.out.println("Test Param: " + testParam); } public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws java.io.IOException, ServletException { // Get the IP address of client machine. String ipAddress = request.getRemoteAddr(); // Log the IP address and current timestamp. System.out.println("IP "+ ipAddress + ", Time "+ new Date().toString()); // Pass request back down the filter chain chain.doFilter(request,response); } public void destroy( ) { /* Called before the Filter instance is removed from service by the web container*/ } }
Compile LogFilter.java in the usual way and put your LogFilter.class file in <Tomcat-installation-directory>/webapps/ROOT/WEB-INF/classes.
JSP Filter Mapping in Web.xml
Filters are defined and then mapped to a URL or JSP file name, in much the same way as Servlet is defined and then mapped to a URL pattern in web.xml file. Create the following entry for filter tag in the deployment descriptor file web.xml
<filter> <filter-name>LogFilter</filter-name> <filter-class>LogFilter</filter-class> <init-param> <param-name>test-param</param-name> <param-value>Initialization Paramter</param-value> </init-param> </filter> <filter-mapping> <filter-name>LogFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping>
The above filter will apply to all the servlets and JSP because we specified /* in our configuration. You can specify a particular servlet or the JSP path if you want to apply filter on few servlets or JSP only.
Now try to call any servlet or JSP and you will see generated log in you web server log. You can use Log4J logger to log above log in a separate file.
Using Multiple Filters
Your web application may define several different filters with a specific purpose. Consider, you define two filters AuthenFilter and LogFilter. Rest of the process will remain as explained above except you need to create a different mapping as mentioned below −
<filter> <filter-name>LogFilter</filter-name> <filter-class>LogFilter</filter-class> <init-param> <param-name>test-param</param-name> <param-value>Initialization Paramter</param-value> </init-param> </filter> <filter> <filter-name>AuthenFilter</filter-name> <filter-class>AuthenFilter</filter-class> <init-param> <param-name>test-param</param-name> <param-value>Initialization Paramter</param-value> </init-param> </filter> <filter-mapping> <filter-name>LogFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <filter-mapping> <filter-name>AuthenFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping>
Filters Application Order
The order of filter-mapping elements in web.xml determines the order in which the web container applies the filter to the servlet or JSP. To reverse the order of the filter, you just need to reverse the filter-mapping elements in the web.xml file.
For example, the above example will apply the LogFilter first and then it will apply AuthenFilter to any servlet or JSP; the following example will reverse the order −
<filter-mapping> <filter-name>AuthenFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <filter-mapping> <filter-name>LogFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping>