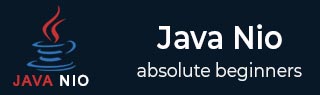
- Java NIO Tutorial
- Java NIO - Home
- Java NIO - Overview
- Java NIO - Environment Setup
- Java NIO vs JAVA IO
- Java NIO - Channels
- Java NIO - File Channel
- Java NIO - DataGram Channel
- Java NIO - Socket Channel
- Java NIO - Server Socket Channel
- Java NIO - Scatter
- Java NIO - Gather
- Java NIO - Buffer
- Java NIO - Selector
- Java NIO - Pipe
- Java NIO - Path
- Java NIO - File
- Java NIO - AsynchronousFileChannel
- Java NIO - CharSet
- Java NIO - FileLock
- Java NIO Useful Resources
- Java NIO - Quick Guide
- Java NIO - Useful Resources
- Java NIO - Discussion
Java NIO - Scatter
As we know that Java NIO is a more optimized API for data IO operations as compared to the conventional IO API of Java.One more additional support which Java NIO provides is to read/write data from/to multiple buffers to channel.This multiple read and write support is termed as Scatter and Gather in which data is scattered to multiple buffers from single channel in case of read data while data is gathered from multiple buffers to single channel in case of write data.
In order to achieve this multiple read and write from channel there is ScatteringByteChannel and GatheringByteChannel API which Java NIO provides for read and write the data as illustrate in below example.
ScatteringByteChannel
Read from multiple channels − In this we made to reads data from a single channel into multiple buffers.For this multiple buffers are allocated and are added to a buffer type array.Then this array is passed as parameter to the ScatteringByteChannel read() method which then writes data from the channel in the sequence the buffers occur in the array.Once a buffer is full, the channel moves on to fill the next buffer.
The following example shows how scattering of data is performed in Java NIO
C:/Test/temp.txt
Hello World!
import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; import java.nio.ByteBuffer; import java.nio.channels.ScatteringByteChannel; public class ScatterExample { private static String FILENAME = "C:/Test/temp.txt"; public static void main(String[] args) { ByteBuffer bLen1 = ByteBuffer.allocate(1024); ByteBuffer bLen2 = ByteBuffer.allocate(1024); FileInputStream in; try { in = new FileInputStream(FILENAME); ScatteringByteChannel scatter = in.getChannel(); scatter.read(new ByteBuffer[] {bLen1, bLen2}); bLen1.position(0); bLen2.position(0); int len1 = bLen1.asIntBuffer().get(); int len2 = bLen2.asIntBuffer().get(); System.out.println("Scattering : Len1 = " + len1); System.out.println("Scattering : Len2 = " + len2); } catch (FileNotFoundException exObj) { exObj.printStackTrace(); } catch (IOException ioObj) { ioObj.printStackTrace(); } } }
Output
Scattering : Len1 = 1214606444 Scattering : Len2 = 0
In last it can be concluded that scatter/gather approach in Java NIO is introduced as an optimized and multitasked when used properly.It allows you to delegate to the operating system the grunt work of separating out the data you read into multiple buckets, or assembling disparate chunks of data into a whole.No doubt this saves time and uses operating system more efficiently by avoiding buffer copies, and reduces the amount of code need to write and debug.