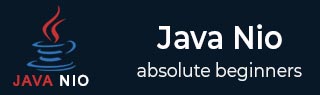
- Java NIO Tutorial
- Java NIO - Home
- Java NIO - Overview
- Java NIO - Environment Setup
- Java NIO vs JAVA IO
- Java NIO - Channels
- Java NIO - File Channel
- Java NIO - DataGram Channel
- Java NIO - Socket Channel
- Java NIO - Server Socket Channel
- Java NIO - Scatter
- Java NIO - Gather
- Java NIO - Buffer
- Java NIO - Selector
- Java NIO - Pipe
- Java NIO - Path
- Java NIO - File
- Java NIO - AsynchronousFileChannel
- Java NIO - CharSet
- Java NIO - FileLock
- Java NIO Useful Resources
- Java NIO - Quick Guide
- Java NIO - Useful Resources
- Java NIO - Discussion
Java NIO - Datagram Channel
Java NIO Datagram is used as channel which can send and receive UDP packets over a connection less protocol.By default datagram channel is blocking while it can be use in non blocking mode.In order to make it non-blocking we can use the configureBlocking(false) method.DataGram channel can be open by calling its one of the static method named as open() which can also take IP address as parameter so that it can be used for multi casting.
Datagram channel alike of FileChannel do not connected by default in order to make it connected we have to explicitly call its connect() method.However datagram channel need not be connected in order for the send and receive methods to be used while it must be connected in order to use the read and write methods, since those methods do not accept or return socket addresses.
We can check the connection status of datagram channel by calling its isConnected() method.Once connected, a datagram channel remains connected until it is disconnected or closed.Datagram channels are thread safe and supports multi-threading and concurrency simultaneously.
Important methods of datagram channel
bind(SocketAddress local) − This method is used to bind the datagram channel's socket to the local address which is provided as the parameter to this method.
connect(SocketAddress remote) − This method is used to connect the socket to the remote address.
disconnect() − This method is used to disconnect the socket to the remote address.
getRemoteAddress() − This method return the address of remote location to which the channel's socket is connected.
isConnected() − As already mentioned this method returns the status of connection of datagram channel i.e whether it is connected or not.
open() and open(ProtocolFamily family) − Open method is used open a datagram channel for single address while parametrized open method open channel for multiple addresses represented as protocol family.
read(ByteBuffer dst) − This method is used to read data from the given buffer through datagram channel.
receive(ByteBuffer dst) − This method is used to receive datagram via this channel.
send(ByteBuffer src, SocketAddress target) − This method is used to send datagram via this channel.
Example
The following example shows the how to send data from Java NIO DataGramChannel.
Server: DatagramChannelServer.java
import java.io.IOException; import java.net.InetSocketAddress; import java.net.SocketAddress; import java.nio.ByteBuffer; import java.nio.channels.DatagramChannel; public class DatagramChannelServer { public static void main(String[] args) throws IOException { DatagramChannel server = DatagramChannel.open(); InetSocketAddress iAdd = new InetSocketAddress("localhost", 8989); server.bind(iAdd); System.out.println("Server Started: " + iAdd); ByteBuffer buffer = ByteBuffer.allocate(1024); //receive buffer from client. SocketAddress remoteAdd = server.receive(buffer); //change mode of buffer buffer.flip(); int limits = buffer.limit(); byte bytes[] = new byte[limits]; buffer.get(bytes, 0, limits); String msg = new String(bytes); System.out.println("Client at " + remoteAdd + " sent: " + msg); server.send(buffer,remoteAdd); server.close(); } }
Output
Server Started: localhost/127.0.0.1:8989
Client: DatagramChannelClient.java
import java.io.IOException; import java.net.InetSocketAddress; import java.net.SocketAddress; import java.nio.ByteBuffer; import java.nio.channels.DatagramChannel; public class DatagramChannelClient { public static void main(String[] args) throws IOException { DatagramChannel client = null; client = DatagramChannel.open(); client.bind(null); String msg = "Hello World!"; ByteBuffer buffer = ByteBuffer.wrap(msg.getBytes()); InetSocketAddress serverAddress = new InetSocketAddress("localhost", 8989); client.send(buffer, serverAddress); buffer.clear(); client.receive(buffer); buffer.flip(); client.close(); } }
Output
Running the client will print the following output on server.
Server Started: localhost/127.0.0.1:8989 Client at /127.0.0.1:64857 sent: Hello World!