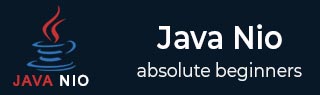
- Java NIO Tutorial
- Java NIO - Home
- Java NIO - Overview
- Java NIO - Environment Setup
- Java NIO vs JAVA IO
- Java NIO - Channels
- Java NIO - File Channel
- Java NIO - DataGram Channel
- Java NIO - Socket Channel
- Java NIO - Server Socket Channel
- Java NIO - Scatter
- Java NIO - Gather
- Java NIO - Buffer
- Java NIO - Selector
- Java NIO - Pipe
- Java NIO - Path
- Java NIO - File
- Java NIO - AsynchronousFileChannel
- Java NIO - CharSet
- Java NIO - FileLock
- Java NIO Useful Resources
- Java NIO - Quick Guide
- Java NIO - Useful Resources
- Java NIO - Discussion
Java NIO - Channels
Description
As name suggests channel is used as mean of data flow from one end to other.Here in java NIO channel act same between buffer and an entity at other end in other words channel are use to read data to buffer and also write data from buffer.
Unlike from streams which are used in conventional Java IO channels are two way i.e can read as well as write.Java NIO channel supports asynchronous flow of data both in blocking and non blocking mode.
Implementations of Channel
Java NIO channel is implemented primarily in following classes −
FileChannel − In order to read data from file we uses file channel. Object of file channel can be created only by calling the getChannel() method on file object as we can't create file object directly.
DatagramChannel − The datagram channel can read and write the data over the network via UDP (User Datagram Protocol).Object of DataGramchannel can be created using factory methods.
SocketChannel − The SocketChannel channel can read and write the data over the network via TCP (Transmission Control Protocol). It also uses the factory methods for creating the new object.
ServerSocketChannel − The ServerSocketChannel read and write the data over TCP connections, same as a web server. For every incoming connection a SocketChannel is created.
Example
Following example reads from a text file from C:/Test/temp.txt and prints the content to the console.
temp.txt
Hello World!
ChannelDemo.java
import java.io.IOException; import java.io.RandomAccessFile; import java.nio.ByteBuffer; import java.nio.channels.FileChannel; public class ChannelDemo { public static void main(String args[]) throws IOException { RandomAccessFile file = new RandomAccessFile("C:/Test/temp.txt", "r"); FileChannel fileChannel = file.getChannel(); ByteBuffer byteBuffer = ByteBuffer.allocate(512); while (fileChannel.read(byteBuffer) > 0) { // flip the buffer to prepare for get operation byteBuffer.flip(); while (byteBuffer.hasRemaining()) { System.out.print((char) byteBuffer.get()); } } file.close(); } }
Output
Hello World!