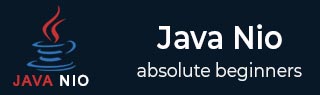
- Java NIO Tutorial
- Java NIO - Home
- Java NIO - Overview
- Java NIO - Environment Setup
- Java NIO vs JAVA IO
- Java NIO - Channels
- Java NIO - File Channel
- Java NIO - DataGram Channel
- Java NIO - Socket Channel
- Java NIO - Server Socket Channel
- Java NIO - Scatter
- Java NIO - Gather
- Java NIO - Buffer
- Java NIO - Selector
- Java NIO - Pipe
- Java NIO - Path
- Java NIO - File
- Java NIO - AsynchronousFileChannel
- Java NIO - CharSet
- Java NIO - FileLock
- Java NIO Useful Resources
- Java NIO - Quick Guide
- Java NIO - Useful Resources
- Java NIO - Discussion
Java NIO - Pipe
In Java NIO pipe is a component which is used to write and read data between two threads.Pipe mainly consist of two channels which are responsible for data propagation.
Among two constituent channels one is called as Sink channel which is mainly for writing data and other is Source channel whose main purpose is to read data from Sink channel.
Data synchronization is kept in order during data writing and reading as it must be ensured that data must be read in a same order in which it is written to the Pipe.
It must kept in notice that it is a unidirectional flow of data in Pipe i.e data is written in Sink channel only and could only be read from Source channel.
In Java NIO pipe is defined as a abstract class with mainly three methods out of which two are abstract.
Methods of Pipe class
open() − This method is used get an instance of Pipe or we can say pipe is created by calling out this method.
sink() − This method returns the Pipe's sink channel which is used to write data by calling its write method.
source() − This method returns the Pipe's source channel which is used to read data by calling its read method.
Example
The following example shows the implementation of Java NIO pipe.
import java.io.IOException; import java.nio.ByteBuffer; import java.nio.channels.Pipe; public class PipeDemo { public static void main(String[] args) throws IOException { //An instance of Pipe is created Pipe pipe = Pipe.open(); // gets the pipe's sink channel Pipe.SinkChannel skChannel = pipe.sink(); String testData = "Test Data to Check java NIO Channels Pipe."; ByteBuffer buffer = ByteBuffer.allocate(512); buffer.clear(); buffer.put(testData.getBytes()); buffer.flip(); //write data into sink channel. while(buffer.hasRemaining()) { skChannel.write(buffer); } //gets pipe's source channel Pipe.SourceChannel sourceChannel = pipe.source(); buffer = ByteBuffer.allocate(512); //write data into console while(sourceChannel.read(buffer) > 0){ //limit is set to current position and position is set to zero buffer.flip(); while(buffer.hasRemaining()){ char ch = (char) buffer.get(); System.out.print(ch); } //position is set to zero and limit is set to capacity to clear the buffer. buffer.clear(); } } }
Output
Test Data to Check java NIO Channels Pipe.
Assuming we have a text file c:/test.txt, which has the following content. This file will be used as an input for our example program.