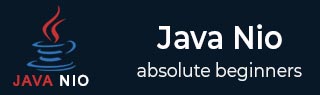
- Java NIO Tutorial
- Java NIO - Home
- Java NIO - Overview
- Java NIO - Environment Setup
- Java NIO vs JAVA IO
- Java NIO - Channels
- Java NIO - File Channel
- Java NIO - DataGram Channel
- Java NIO - Socket Channel
- Java NIO - Server Socket Channel
- Java NIO - Scatter
- Java NIO - Gather
- Java NIO - Buffer
- Java NIO - Selector
- Java NIO - Pipe
- Java NIO - Path
- Java NIO - File
- Java NIO - AsynchronousFileChannel
- Java NIO - CharSet
- Java NIO - FileLock
- Java NIO Useful Resources
- Java NIO - Quick Guide
- Java NIO - Useful Resources
- Java NIO - Discussion
Java NIO - File
Java NIO package provide one more utility API named as Files which is basically used for manipulating files and directories using its static methods which mostly works on Path object.
As mentioned in Path tutorial that Path interface is introduced in Java NIO package during Java 7 version in file package.So this tutorial is for same File package.
This class consists exclusively of static methods that operate on files, directories, or other types of files.In most cases, the methods defined here will delegate to the associated file system provider to perform the file operations.
There are many methods defined in the Files class which could also be read from Java docs.In this tutorial we tried to cover some of the important methods among all of the methods of Java NIO Files class.
Important methods of Files class.
Following are the important methods defined in Java NIO Files class.
createFile(Path filePath, FileAttribute attrs) − Files class provides this method to create file using specified Path.
Example
package com.java.nio; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; public class CreateFile { public static void main(String[] args) { //initialize Path object Path path = Paths.get("D:file.txt"); //create file try { Path createdFilePath = Files.createFile(path); System.out.println("Created a file at : "+createdFilePath); } catch (IOException e) { e.printStackTrace(); } } }
Output
Created a file at : D:\data\file.txt
copy(InputStream in, Path target, CopyOption… options) − This method is used to copies all bytes from specified input stream to specified target file and returns number of bytes read or written as long value.LinkOption for this parameter with the following values −
COPY_ATTRIBUTES − copy attributes to the new file, e.g. last-modified-time attribute.
REPLACE_EXISTING − replace an existing file if it exists.
NOFOLLOW_LINKS − If a file is a symbolic link, then the link itself, not the target of the link, is copied.
Example
package com.java.nio; import java.io.IOException; import java.nio.charset.Charset; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.nio.file.StandardCopyOption; import java.util.List; public class WriteFile { public static void main(String[] args) { Path sourceFile = Paths.get("D:file.txt"); Path targetFile = Paths.get("D:fileCopy.txt"); try { Files.copy(sourceFile, targetFile, StandardCopyOption.REPLACE_EXISTING); } catch (IOException ex) { System.err.format("I/O Error when copying file"); } Path wiki_path = Paths.get("D:fileCopy.txt"); Charset charset = Charset.forName("ISO-8859-1"); try { List<String> lines = Files.readAllLines(wiki_path, charset); for (String line : lines) { System.out.println(line); } } catch (IOException e) { System.out.println(e); } } }
Output
To be or not to be?
createDirectories(Path dir, FileAttribute<?>...attrs) − This method is used to create directories using given path by creating all nonexistent parent directories.
delete(Path path) − This method is used to deletes the file from specified path.It throws NoSuchFileException if the file is not exists at specified path or if the file is directory and it may not empty and cannot be deleted.
exists(Path path) − This method is used to check if file exists at specified path and if the file exists it will return true or else it returns false.
readAllBytes(Path path) − This method is used to reads all the bytes from the file at given path and returns the byte array containing the bytes read from the file.
Example
package com.java.nio; import java.io.IOException; import java.nio.charset.Charset; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.util.List; public class ReadFile { public static void main(String[] args) { Path wiki_path = Paths.get("D:file.txt"); Charset charset = Charset.forName("ISO-8859-1"); try { List<String> lines = Files.readAllLines(wiki_path, charset); for (String line : lines) { System.out.println(line); } } catch (IOException e) { System.out.println(e); } } }
Output
Welcome to file.
size(Path path) − This method is used to get the size of the file at specified path in bytes.
write(Path path, byte[] bytes, OpenOption… options) − This method is used to writes bytes to a file at specified path.
Example
package com.java.nio; import java.io.IOException; import java.nio.charset.Charset; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.util.List; public class WriteFile { public static void main(String[] args) { Path path = Paths.get("D:file.txt"); String question = "To be or not to be?"; Charset charset = Charset.forName("ISO-8859-1"); try { Files.write(path, question.getBytes()); List<String> lines = Files.readAllLines(path, charset); for (String line : lines) { System.out.println(line); } } catch (IOException e) { System.out.println(e); } } }
Output
To be or not to be?