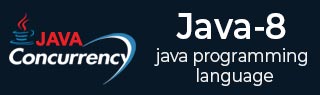
- Java Concurrency Tutorial
- Concurrency - Home
- Concurrency - Overview
- Concurrency - Environment Setup
- Concurrency - Major Operations
- Interthread Communication
- Concurrency - Synchronization
- Concurrency - Deadlock
- Utility Class Examples
- Concurrency - ThreadLocal
- Concurrency - ThreadLocalRandom
- Lock Examples
- Concurrency - Lock
- Concurrency - ReadWriteLock
- Concurrency - Condition
- Atomic Variable Examples
- Concurrency - AtomicInteger
- Concurrency - AtomicLong
- Concurrency - AtomicBoolean
- Concurrency - AtomicReference
- Concurrency - AtomicIntegerArray
- Concurrency - AtomicLongArray
- Concurrency - AtomicReferenceArray
- Executor Examples
- Concurrency - Executor
- Concurrency - ExecutorService
- ScheduledExecutorService
- Thread Pool Examples
- Concurrency - newFixedThreadPool
- Concurrency - newCachedThreadPool
- newScheduledThreadPool
- newSingleThreadExecutor
- Concurrency - ThreadPoolExecutor
- ScheduledThreadPoolExecutor
- Advanced Examples
- Concurrency - Futures and Callables
- Concurrency - Fork-Join framework
- Concurrent Collections
- Concurrency - BlockingQueue
- Concurrency - ConcurrentMap
- ConcurrentNavigableMap
- Concurrency Useful Resources
- Concurrency - Quick Guide
- Concurrency - Useful Resources
- Concurrency - Discussion
Java Concurrency - ThreadLocal Class
The ThreadLocal class is used to create thread local variables which can only be read and written by the same thread. For example, if two threads are accessing code having reference to same threadLocal variable then each thread will not see any modification to threadLocal variable done by other thread.
ThreadLocal Methods
Following is the list of important methods available in the ThreadLocal class.
Sr.No. | Method & Description |
---|---|
1 | public T get() Returns the value in the current thread's copy of this thread-local variable. |
2 | protected T initialValue() Returns the current thread's "initial value" for this thread-local variable. |
3 | public void remove() Removes the current thread's value for this thread-local variable. |
4 | public void set(T value) Sets the current thread's copy of this thread-local variable to the specified value. |
Example
The following TestThread program demonstrates some of these methods of the ThreadLocal class. Here we've used two counter variable, one is normal variable and another one is ThreadLocal.
class RunnableDemo implements Runnable { int counter; ThreadLocal<Integer> threadLocalCounter = new ThreadLocal<Integer>(); public void run() { counter++; if(threadLocalCounter.get() != null) { threadLocalCounter.set(threadLocalCounter.get().intValue() + 1); } else { threadLocalCounter.set(0); } System.out.println("Counter: " + counter); System.out.println("threadLocalCounter: " + threadLocalCounter.get()); } } public class TestThread { public static void main(String args[]) { RunnableDemo commonInstance = new RunnableDemo(); Thread t1 = new Thread(commonInstance); Thread t2 = new Thread(commonInstance); Thread t3 = new Thread(commonInstance); Thread t4 = new Thread(commonInstance); t1.start(); t2.start(); t3.start(); t4.start(); // wait for threads to end try { t1.join(); t2.join(); t3.join(); t4.join(); } catch (Exception e) { System.out.println("Interrupted"); } } }
This will produce the following result.
Output
Counter: 1 threadLocalCounter: 0 Counter: 2 threadLocalCounter: 0 Counter: 3 threadLocalCounter: 0 Counter: 4 threadLocalCounter: 0
You can see the value of counter is increased by each thread, but threadLocalCounter remains 0 for each thread.