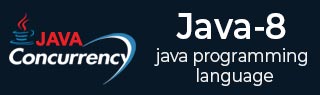
- Java Concurrency Tutorial
- Concurrency - Home
- Concurrency - Overview
- Concurrency - Environment Setup
- Concurrency - Major Operations
- Interthread Communication
- Concurrency - Synchronization
- Concurrency - Deadlock
- Utility Class Examples
- Concurrency - ThreadLocal
- Concurrency - ThreadLocalRandom
- Lock Examples
- Concurrency - Lock
- Concurrency - ReadWriteLock
- Concurrency - Condition
- Atomic Variable Examples
- Concurrency - AtomicInteger
- Concurrency - AtomicLong
- Concurrency - AtomicBoolean
- Concurrency - AtomicReference
- Concurrency - AtomicIntegerArray
- Concurrency - AtomicLongArray
- Concurrency - AtomicReferenceArray
- Executor Examples
- Concurrency - Executor
- Concurrency - ExecutorService
- ScheduledExecutorService
- Thread Pool Examples
- Concurrency - newFixedThreadPool
- Concurrency - newCachedThreadPool
- newScheduledThreadPool
- newSingleThreadExecutor
- Concurrency - ThreadPoolExecutor
- ScheduledThreadPoolExecutor
- Advanced Examples
- Concurrency - Futures and Callables
- Concurrency - Fork-Join framework
- Concurrent Collections
- Concurrency - BlockingQueue
- Concurrency - ConcurrentMap
- ConcurrentNavigableMap
- Concurrency Useful Resources
- Concurrency - Quick Guide
- Concurrency - Useful Resources
- Concurrency - Discussion
Interthread Communication
If you are aware of interprocess communication then it will be easy for you to understand interthread communication. Interthread communication is important when you develop an application where two or more threads exchange some information.
There are three simple methods and a little trick which makes thread communication possible. All the three methods are listed below −
Sr.No. | Method & Description |
---|---|
1 | public void wait() Causes the current thread to wait until another thread invokes the notify(). |
2 | public void notify() Wakes up a single thread that is waiting on this object's monitor. |
3 | public void notifyAll() Wakes up all the threads that called wait( ) on the same object. |
These methods have been implemented as final methods in Object, so they are available in all the classes. All three methods can be called only from within a synchronized context.
Example
This examples shows how two threads can communicate using wait() and notify() method. You can create a complex system using the same concept.
class Chat { boolean flag = false; public synchronized void Question(String msg) { if (flag) { try { wait(); } catch (InterruptedException e) { e.printStackTrace(); } } System.out.println(msg); flag = true; notify(); } public synchronized void Answer(String msg) { if (!flag) { try { wait(); } catch (InterruptedException e) { e.printStackTrace(); } } System.out.println(msg); flag = false; notify(); } } class T1 implements Runnable { Chat m; String[] s1 = { "Hi", "How are you ?", "I am also doing fine!" }; public T1(Chat m1) { this.m = m1; new Thread(this, "Question").start(); } public void run() { for (int i = 0; i < s1.length; i++) { m.Question(s1[i]); } } } class T2 implements Runnable { Chat m; String[] s2 = { "Hi", "I am good, what about you?", "Great!" }; public T2(Chat m2) { this.m = m2; new Thread(this, "Answer").start(); } public void run() { for (int i = 0; i < s2.length; i++) { m.Answer(s2[i]); } } } public class TestThread { public static void main(String[] args) { Chat m = new Chat(); new T1(m); new T2(m); } }
When the above program is complied and executed, it produces the following result −
Output
Hi Hi How are you ? I am good, what about you? I am also doing fine! Great!
Above example has been taken and then modified from [https://stackoverflow.com/questions/2170520/inter-thread-communication-in-java]
To Continue Learning Please Login