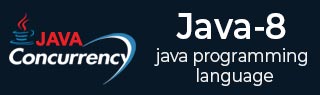
- Java Concurrency Tutorial
- Concurrency - Home
- Concurrency - Overview
- Concurrency - Environment Setup
- Concurrency - Major Operations
- Interthread Communication
- Concurrency - Synchronization
- Concurrency - Deadlock
- Utility Class Examples
- Concurrency - ThreadLocal
- Concurrency - ThreadLocalRandom
- Lock Examples
- Concurrency - Lock
- Concurrency - ReadWriteLock
- Concurrency - Condition
- Atomic Variable Examples
- Concurrency - AtomicInteger
- Concurrency - AtomicLong
- Concurrency - AtomicBoolean
- Concurrency - AtomicReference
- Concurrency - AtomicIntegerArray
- Concurrency - AtomicLongArray
- Concurrency - AtomicReferenceArray
- Executor Examples
- Concurrency - Executor
- Concurrency - ExecutorService
- ScheduledExecutorService
- Thread Pool Examples
- Concurrency - newFixedThreadPool
- Concurrency - newCachedThreadPool
- newScheduledThreadPool
- newSingleThreadExecutor
- Concurrency - ThreadPoolExecutor
- ScheduledThreadPoolExecutor
- Advanced Examples
- Concurrency - Futures and Callables
- Concurrency - Fork-Join framework
- Concurrent Collections
- Concurrency - BlockingQueue
- Concurrency - ConcurrentMap
- ConcurrentNavigableMap
- Concurrency Useful Resources
- Concurrency - Quick Guide
- Concurrency - Useful Resources
- Concurrency - Discussion
newScheduledThreadPool Method
A scheduled thread pool can be obtainted by calling the static newScheduledThreadPool() method of Executors class.
Syntax
ExecutorService executor = Executors.newScheduledThreadPool(1);
Example
The following TestThread program shows usage of newScheduledThreadPool method in thread based environment.
import java.util.concurrent.Executors; import java.util.concurrent.ScheduledExecutorService; import java.util.concurrent.ScheduledFuture; import java.util.concurrent.TimeUnit; public class TestThread { public static void main(final String[] arguments) throws InterruptedException { final ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1); final ScheduledFuture<?> beepHandler = scheduler.scheduleAtFixedRate(new BeepTask(), 2, 2, TimeUnit.SECONDS); scheduler.schedule(new Runnable() { @Override public void run() { beepHandler.cancel(true); scheduler.shutdown(); } }, 10, TimeUnit.SECONDS); } static class BeepTask implements Runnable { public void run() { System.out.println("beep"); } } }
This will produce the following result.
Output
beep beep beep beep
Advertisements
To Continue Learning Please Login