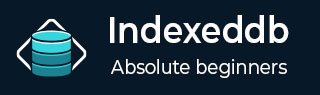
- IndexedDB Tutorial
- IndexedDB - Home
- IndexedDB - Introduction
- IndexedDB - Installation
- IndexedDB - Connection
- IndexedDB - Object Stores
- IndexedDB - Creating Data
- IndexedDB - Reading Data
- IndexedDB - Updating Data
- IndexedDB - Deleting Data
- Using getAll() Functions
- IndexedDB - Indexes
- IndexedDB - Ranges
- IndexedDB - Transactions
- IndexedDB - Error Handling
- IndexedDB - Searching
- IndexedDB - Cursors
- IndexedDB - Promise Wrapper
- IndexedDB - Ecmascript Binding
- IndexedDB Useful Resources
- IndexedDB - Quick Guide
- IndexedDB - Useful Resources
- IndexedDB - Discussion
IndexedDB - Reading Data
We enter data into the database, and we need to call the data to view the changes and also for various other purposes.
We must call the get() method on the object store to read this data. The get method takes the primary key of the object you want to retrieve from the store.
Syntax
var request = objectstore.get(data);
Here, we are requesting the objectstore to get the data using get() function.
Example
Following example is an implementation of requesting the objectstore to get the data −
<!DOCTYPE html> <html lang="en"> <head> <title>Document</title> </head> <body> <script> const request = indexedDB.open("botdatabase",1); request.onupgradeneeded = function(){ const db = request.result; const store = db.createObjectStore("bots",{ keyPath: "id"}); } request.onsuccess = function(){ document.write("database opened successfully"); const db = request.result; const transaction=db.transaction("bots","readwrite"); const store = transaction.objectStore("bots"); store.add({id: 1, name: "jason",branch: "IT"}); store.add({id: 2, name: "praneeth",branch: "CSE"}); store.add({id: 3, name: "palli",branch: "EEE"}); store.add({id: 4, name: "abdul",branch: "IT"}); const idquery = store.get(4); idquery.onsuccess = function(){ document.write("idquery",idquery.result); } transaction.oncomplete = function(){ db.close; } } </script> </body> </html>
Output
database opened successfully idquery {id: 4, name: 'abdul', branch: 'IT'}
Advertisements
To Continue Learning Please Login