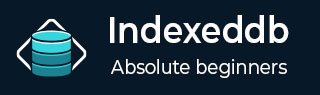
- IndexedDB Tutorial
- IndexedDB - Home
- IndexedDB - Introduction
- IndexedDB - Installation
- IndexedDB - Connection
- IndexedDB - Object Stores
- IndexedDB - Creating Data
- IndexedDB - Reading Data
- IndexedDB - Updating Data
- IndexedDB - Deleting Data
- Using getAll() Functions
- IndexedDB - Indexes
- IndexedDB - Ranges
- IndexedDB - Transactions
- IndexedDB - Error Handling
- IndexedDB - Searching
- IndexedDB - Cursors
- IndexedDB - Promise Wrapper
- IndexedDB - Ecmascript Binding
- IndexedDB Useful Resources
- IndexedDB - Quick Guide
- IndexedDB - Useful Resources
- IndexedDB - Discussion
IndexedDB - Introduction
A database management system provides a mechanism for the storage and retrieval of data. There are various kinds of databases available mostly used ones among them are −
- Hierarchical databases
- Network databases
- Object-oriented databases
- Relational databases
- NoSQL databases
NoSQL databases
A NoSQL database (sometimes called Not Only SQL) is a database that provides a mechanism to store and retrieve data other than the tabular relations used in relational databases. These databases are schema-free, support easy replication, have simple API, are eventually consistent, and can handle huge amounts of data (big data).
There are different types of NoSQL databases also like −
- Document databases.
Key-value stores.
Column-oriented databases.
Graph databases.
What is IndexedDB
Indexed Database is a type of NoSQL database or Non−relational structured query language. It is a transactional database system, like an SQL−based RDBMS. However, unlike SQL−based RDBMSs, which use fixed-column tables, IndexedDB is a JavaScript−based object-oriented database.
It is used when we need to store significant amounts of data on the server side and faster than local storage. Since it stores the data in the browser it can be used online and offline too. Using this, you can create a web application (with rich query abilities) that can run whether an internet connection is available or not.
Key Characteristics of IndexedDB
Following are the key characters of the IndexedDB database −
IndexedDB is a NoSQL database that stores key−value pairs. It can store almost any kind of value by keys or multiple key types.
As mentioned, IndexedDB follows a transactional database model − Transaction is a wrapper class around the operation or group of operations so that data integrity is maintained. You don't want data to be altered or missed so if the transaction is failed a callback is rolled out.
IndexedDB does not use Structured Query Language − Since IndexedDB uses a NoSQL database it doesn’t use SQL, instead, it uses queries on Indexes to produce data via cursors or the getAll() method to iterate around different sets.
IndexedDB uses a lot of requests − Requests are objects that receive the success or failure of DOM events (DOM - HTML DOM events allow JavaScript to register different event handlers on elements in an HTML document). DOM events are success or error which has a target property that indicates the flow of the request.
Success events can’t be canceled but, error events can be canceled. There are a lot of requests in IndexedDB like on success, onerror and addEventListener(), removeEventListener() on them. For getting to know the status of the request we also have ready state, result, and error code properties.
IndexedDB needs to follow the same origin − Origin is the URL of the document in which the script is being written, each origin has some databases under it and each database has its name to be identified by the origin. The security boundary imposed on IndexedDB prevents applications from accessing data with a different origin.
For example, if we take a URL and take different subdirectories of it, it can retrieve data but if we change location to port 8080 and try to retrieve data from the usual URL and the changed port, we cannot retrieve the data.
Terminology
Following are various important terms in indexedDB that you should know before proceeding further −
Database − In IndexedDB database is the highest level which contains the object stores that contain the data.
Object Stores − Object stores are the data storage entities of IndexedDB. Think of it as tables in RDBMS where we store data based on the type of data we want to store (ex: id, name, roll no, etc).
Transaction − For any database operation we do the following process.
- Get database object
- Open transaction on the database
- Open object store on the transaction and then, operate on object store.
So basically, a transaction is a wrapper function that is connected to every database and it ensures data integrity such that if the transaction is canceled or any error happens it will call back to where the transaction has not yet been begun.
Index − Think of object store as a table and we use indexes to retrieve data of a single property from them. Ex: Name, age, etc.
Cursor − In a database, if we need to traverse multiple records from object stores we use cursors.
Support for IndexedDB
IndexedDB is a database in a browser so, we need to check if it is supported by the current/existing browser. To do so, paste the following code in an text editor save it as test.html and run it your browser.
const indexedDB = window.indexedDB || window.mozIndexedDB || window.webkitIndexedDB || window.msIndexedDB || window.shimIndexedDB; if (!indexedDB) { document.write("IndexedDB could not be found in this browser."); } const request = indexedDB.open("MyDatabase", 1);
If IndexedDB is supported by your browser this program gets executed successfully, an a database will be created.
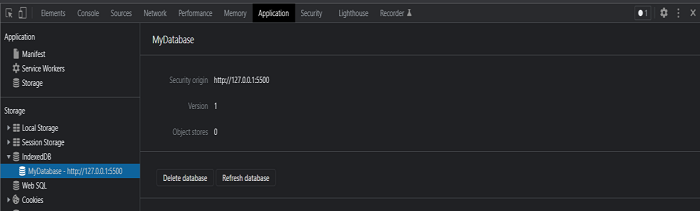