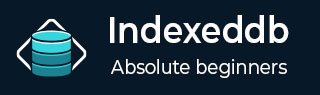
- IndexedDB Tutorial
- IndexedDB - Home
- IndexedDB - Introduction
- IndexedDB - Installation
- IndexedDB - Connection
- IndexedDB - Object Stores
- IndexedDB - Creating Data
- IndexedDB - Reading Data
- IndexedDB - Updating Data
- IndexedDB - Deleting Data
- Using getAll() Functions
- IndexedDB - Indexes
- IndexedDB - Ranges
- IndexedDB - Transactions
- IndexedDB - Error Handling
- IndexedDB - Searching
- IndexedDB - Cursors
- IndexedDB - Promise Wrapper
- IndexedDB - Ecmascript Binding
- IndexedDB Useful Resources
- IndexedDB - Quick Guide
- IndexedDB - Useful Resources
- IndexedDB - Discussion
IndexedDB - Ranges
When we don’t want to get all the data at once we use ranges. When we want to get data in a specific range only we use ranges. We define the range using the IDBKeyRange object. This object has 4 methods which are −
- upperBound()
- lowerBound()
- bound()
- only()
Syntax
IDBKeyRange.lowerBound(indexKey); IDBKeyRange.upperBound(indexKey); IDBKeyRange.bound(lowerIndexKey, upperIndexKey);
Following is the list of various range codes −
S.No. | Range Codes & Description |
---|---|
1 | All keys ≥ a DBKeyRange.lowerBound(a) |
2 | All keys > a IDBKeyRange.lowerBound(a, true) |
3 | All keys ≤ b IDBKeyRange.upperBound(b) |
4 | All keys < b IDBKeyRange.upperBound(b, true) |
5 | All keys ≥ a && ≤ b IDBKeyRange.bound(a, b) |
6 | All keys > a &&< b IDBKeyRange.bound(a, b, true, true) |
7 | All keys > a && ≤ b IDBKeyRange.bound(a, b, true, false) |
8 | All keys ≥ a && < b IDBKeyRange.bound(a, b, false, true) |
9 | The key = c IDBKeyRange.only(c) |
We generally use the indexes for using range and in the syntax, the index key represents the indexes keypath value.
Examples
Various examples to retrieve the range codes using get() and getAll() methods is as follows −
class.get(‘student’) class.getAll(IDBKeyRange.bound(‘science’,’math’) class.getAll(IDBKeyRange.upperbound(‘science’,true) class.getAll() class.getAllKeys(IDBKeyRange.lowerbound(‘student’,true))
HTML Example
Consider the HTML example below to obtain the range codes −
<!DOCTYPE html> <html lang="en"> <head> <title>Document</title> </head> <body> <script> const request = indexedDB.open("botdatabase",1); request.onupgradeneeded = function(){ const db = request.result; const store = db.createObjectStore("bots",{ keyPath: "id"}); store.createIndex("branch_db",["branch"],{unique: false}); } request.onsuccess = function(){ document.write("database opened successfully"); const db = request.result; const transaction=db.transaction("bots","readwrite"); const store = transaction.objectStore("bots"); const branchIndex = store.index("branch_db"); store.add({id: 1, name: "jason",branch: "IT"}); store.add({id: 2, name: "praneeth",branch: "CSE"}); store.add({id: 3, name: "palli",branch: "EEE"}); store.add({id: 4, name: "abdul",branch: "IT"}); store.put({id: 4, name: "deevana",branch: "CSE"}); const upperquery =store.getAll(IDBKeyRange.upperBound('2', true)); upperquery.onsuccess = function(){ document.write("upperquery",upperquery.result); } transaction.oncomplete = function(){ db.close; } } </script> </body> </html>
Output
database opened successfully upperquery (4) [{...}, {...}, {...}, {...}] arg1: (4) [{...}, {...}, {...}, {...}] 0: {id: 1, name: 'jason', branch: 'IT'} 1: {id: 2, name: 'praneeth', branch: 'CSE'} 2: {id: 3, name: 'palli', branch: 'EEE'} 3: {id: 4, name: 'deevana', branch: 'CSE'} length: 4 [[Prototype]]: Array(0) [[Prototype]]: Object