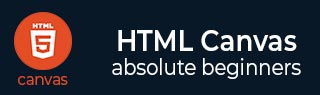
- HTML Canvas Tutorial
- HTML Canvas - Home
- HTML Canvas - Introduction
- Environmental Setup
- HTML Canvas - First Application
- HTML Canvas - Drawing 2D Shapes
- HTML Canvas - Path Elements
- 2D Shapes Using Path Elements
- HTML Canvas - Colors
- HTML Canvas - Adding Styles
- HTML Canvas - Adding Text
- HTML Canvas - Adding Images
- HTML Canvas - Canvas Clock
- HTML Canvas - Transformations
- Composting and Clipping
- HTML Canvas - Basic Animations
- Advanced Animations
- HTML Canvas API Functions
- HTML Canvas - Element
- HTML Canvas - Rectangles
- HTML Canvas - Lines
- HTML Canvas - Paths
- HTML Canvas - Text
- HTML Canvas - Colors and Styles
- HTML Canvas - Images
- HTML Canvas - Shadows and Transformations
- HTML Canvas Useful Resources
- HTML Canvas - Quick Guide
- HTML Canvas - Useful Resources
- HTML Canvas - Discussion
HTML Canvas - setLineDash() Method
The HTML Canvas setLineDash() method is used to set the line dash pattern when there is a need to stroke the lines in Canvas element.
It takes an array specifying the lengths and gaps values and applies the style. It is available in the CanvasRenderingContext2D interface.
Syntax
Following is the syntax of HTML Canvas setLineDash() method −
CanvasRenderingContext2D.setLineDash(values);
Parameters
Following is the parameter used by this method
S.No | Parameter & Description |
---|---|
1 | values
An array of numbers representing the distances to maintain the space between each line dash and the length of the line dash pattern. |
Return values
A line dash pattern is returned on the canvas element only when it is stroked or filled by using their respective methods.
Example
The following example draws a dashed line by using the HTML Canvas setLineDash() method for the CanvasRenderingContext2D object.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="200" height="150" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.setLineDash([4, 16]); context.beginPath(); context.strokeStyle = 'blue'; context.moveTo(25, 25); context.lineTo(125, 25); context.lineTo(125, 125); context.stroke(); </script> </body> </html>
Output
The output returned by the above code on the webpage as −
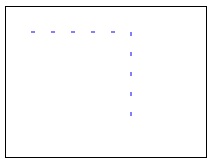
Example
The following example draws a square onto the Canvas and applies setLineDash() method to its borders to get the line dash pattern.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="200" height="150" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.beginPath(); context.lineWidth = 10; context.strokeStyle = 'black'; context.moveTo(25, 25); context.lineTo(25, 125); context.lineTo(125, 125); context.stroke(); context.closePath(); context.setLineDash([4, 16]); context.beginPath(); context.lineWidth = 1; context.strokeStyle = 'blue'; context.moveTo(25, 25); context.lineTo(125, 25); context.lineTo(125, 125); context.stroke(); context.closePath(); </script> </body> </html>
Output
The output returned by the above code on the webpage as −
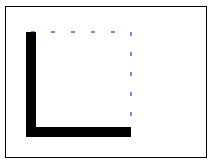
To Continue Learning Please Login