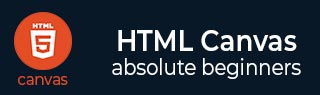
- HTML Canvas Tutorial
- HTML Canvas - Home
- HTML Canvas - Introduction
- Environmental Setup
- HTML Canvas - First Application
- HTML Canvas - Drawing 2D Shapes
- HTML Canvas - Path Elements
- 2D Shapes Using Path Elements
- HTML Canvas - Colors
- HTML Canvas - Adding Styles
- HTML Canvas - Adding Text
- HTML Canvas - Adding Images
- HTML Canvas - Canvas Clock
- HTML Canvas - Transformations
- Composting and Clipping
- HTML Canvas - Basic Animations
- Advanced Animations
- HTML Canvas API Functions
- HTML Canvas - Element
- HTML Canvas - Rectangles
- HTML Canvas - Lines
- HTML Canvas - Paths
- HTML Canvas - Text
- HTML Canvas - Colors and Styles
- HTML Canvas - Images
- HTML Canvas - Shadows and Transformations
- HTML Canvas Useful Resources
- HTML Canvas - Quick Guide
- HTML Canvas - Useful Resources
- HTML Canvas - Discussion
HTML Canvas - Advanced Animations
In the previous chapter, Basic animations help us to understand how to animate the Canvas element. Here, we will be looking at the physical concepts of animation such as velocity, acceleration, etc.
Let us work on a simple acceleration example where we use a small square to expand and collapse. The implementation code is given below.
Example
<!DOCTYPE html> <html lang="en"> <head> <title>Advanced Animations</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="animation();"> <canvas id="canvas" width="555" height="555" style="border: 1px solid black;"></canvas> <script> function animation() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); var reqframe; var square = { posx: 0, posy: 0, width: 50, height: 50, vx: 2, vy: 1, draw: function() { context.fillRect(this.posx, this.posy, this.width, this.height); context.fillStyle = 'red'; context.fill(); } }; function draw() { context.clearRect(0, 0, canvas.width, canvas.height); square.draw(); square.width += square.vx; square.height += square.vy; if (square.height + square.vy > canvas.height || square.height + square.vy < 0) { square.vy = -square.vy; } if (square.width + square.vx > canvas.width || square.width + square.vx < 0) { square.vx = -square.vx; } reqframe = window.requestAnimationFrame(draw); } canvas.addEventListener('mouseover', function(e) { reqframe = window.requestAnimationFrame(draw); }); canvas.addEventListener('mouseout', function(e) { window.cancelAnimationFrame(reqframe); }); } </script> </body> </html>
Output
The output returned by the code is

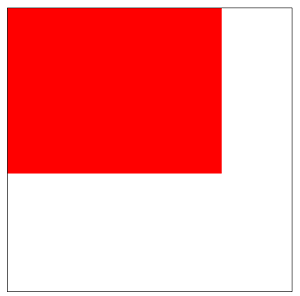
Advertisements
To Continue Learning Please Login