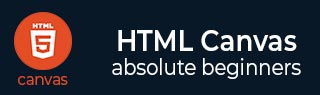
- HTML Canvas Tutorial
- HTML Canvas - Home
- HTML Canvas - Introduction
- Environmental Setup
- HTML Canvas - First Application
- HTML Canvas - Drawing 2D Shapes
- HTML Canvas - Path Elements
- 2D Shapes Using Path Elements
- HTML Canvas - Colors
- HTML Canvas - Adding Styles
- HTML Canvas - Adding Text
- HTML Canvas - Adding Images
- HTML Canvas - Canvas Clock
- HTML Canvas - Transformations
- Composting and Clipping
- HTML Canvas - Basic Animations
- Advanced Animations
- HTML Canvas API Functions
- HTML Canvas - Element
- HTML Canvas - Rectangles
- HTML Canvas - Lines
- HTML Canvas - Paths
- HTML Canvas - Text
- HTML Canvas - Colors and Styles
- HTML Canvas - Images
- HTML Canvas - Shadows and Transformations
- HTML Canvas Useful Resources
- HTML Canvas - Quick Guide
- HTML Canvas - Useful Resources
- HTML Canvas - Discussion
HTML Canvas - resetTransform() Method
The HTML Canvas resetTransform() method of Canvas 2D API completely resets the transformation methods applied to the interface context object of CanvasRenderingContext2D.
Syntax
Following is the syntax of HTML Canvas resetTransform() method −
CanvasRenderingContext2D.resetTransform();
Parameters
It does not take any parameters as it only resets the transformation matrix to the applied context object of CanvasRenderingContext2D interface.
Return Value
The current transformation matrix applied to the Context object is reset to the identity matrix when resetTransform() is called.
Example 1
The following example takes rectangle shape and rotates it by modifying the matrix, it is then reset to the identity matrix using HTML Canvas resetTransform() method.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="200" height="200" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById("canvas"); var context = canvas.getContext("2d"); context.rotate(75 * Math.PI / 180); context.fillStyle = 'blue'; context.fillRect(45, -100, 150, 100); context.resetTransform(); } </script> </body> </html>
Output
The output returned by the following code on the webpage as −
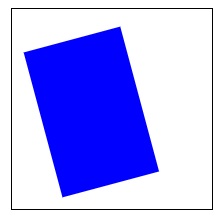
Example 2
The following example takes rectangle shape and rotates it by modifying the matrix, it is then reset to the identity matrix using resetTransform() method.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="400" height="200" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById("canvas"); var context = canvas.getContext("2d"); context.transform(1, 0, 1.7, 1, 0, 0); context.beginPath(); context.fillStyle = 'gray'; context.arc(100, 100, 50, 1 * Math.PI, 5 * Math.PI); context.fill(); context.closePath(); context.resetTransform(); context.beginPath(); context.fillStyle = 'red'; context.arc(100, 100, 50, 1 * Math.PI, 5 * Math.PI); context.fill(); context.closePath(); } </script> </body> </html>
Output
The output returned by the following code on the webpage as −
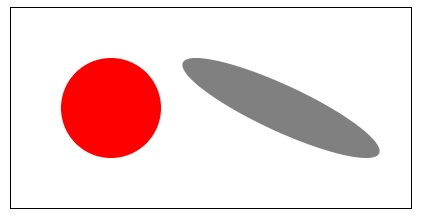
To Continue Learning Please Login