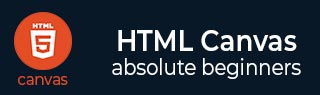
- HTML Canvas Tutorial
- HTML Canvas - Home
- HTML Canvas - Introduction
- Environmental Setup
- HTML Canvas - First Application
- HTML Canvas - Drawing 2D Shapes
- HTML Canvas - Path Elements
- 2D Shapes Using Path Elements
- HTML Canvas - Colors
- HTML Canvas - Adding Styles
- HTML Canvas - Adding Text
- HTML Canvas - Adding Images
- HTML Canvas - Canvas Clock
- HTML Canvas - Transformations
- Composting and Clipping
- HTML Canvas - Basic Animations
- Advanced Animations
- HTML Canvas API Functions
- HTML Canvas - Element
- HTML Canvas - Rectangles
- HTML Canvas - Lines
- HTML Canvas - Paths
- HTML Canvas - Text
- HTML Canvas - Colors and Styles
- HTML Canvas - Images
- HTML Canvas - Shadows and Transformations
- HTML Canvas Useful Resources
- HTML Canvas - Quick Guide
- HTML Canvas - Useful Resources
- HTML Canvas - Discussion
HTML Canvas - Canvas Property
The HTML Canvas property of the Canvas API is a reference to the Canvas context object. It helps us to define the canvas tag and use it to develop graphics using the canvas object. This is achieved by using JavaScript code.
Possible input values
It takes the canvas tag and the provided code snippet and constructs a new Canvas element object with the specified dimensions and the styles given.
Using this context can be provided to the Canvas element and graphics can be rendered using the available methods and properties.
Example
The following example demonstrates how the HTML Canvas property can be used to generate a new context object which can be further used to render shapes.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" height="200" width="200" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); console.log(context.canvas); </script> </body> </html>
Output
The output returned by the above code in the webpage console as −
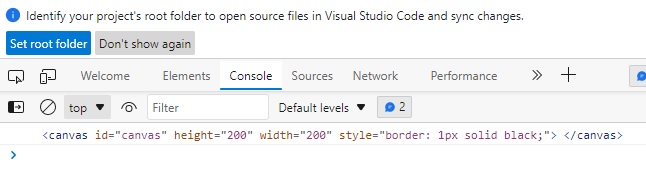
The output seen on the webpage as −
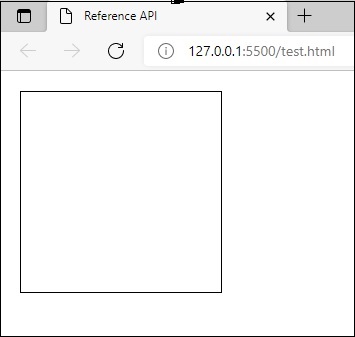
Example
The following example demonstrates how canvas property can be used to generate a new context object and the border is styled by increasing size and adding color.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } #canvas { border: 10px solid green; } </style> </head> <body> <canvas id="canvas" height="200" width="200"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); </script> </body> </html>
Output
The output returned by the above code in the webpage as −
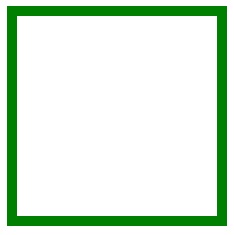
Example
The following example demonstrates how canvas property can be used to generate a new context object and the background is filled.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } #canvas { background-color: aqua; } </style> </head> <body> <canvas id="canvas"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); </script> </body> </html>
Output
The output returned by the above code in the webpage as −
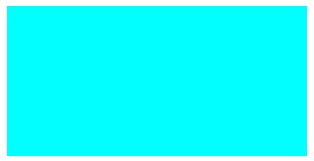
Example
The following example demonstrates how canvas property can be used to generate a new context object and the border is styled using CSS styles.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } #canvas { border: 50px groove grey; } </style> </head> <body> <canvas id="canvas"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); </script> </body> </html>
Output
The output returned by the above code in the webpage as −

To Continue Learning Please Login