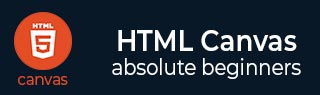
- HTML Canvas Tutorial
- HTML Canvas - Home
- HTML Canvas - Introduction
- Environmental Setup
- HTML Canvas - First Application
- HTML Canvas - Drawing 2D Shapes
- HTML Canvas - Path Elements
- 2D Shapes Using Path Elements
- HTML Canvas - Colors
- HTML Canvas - Adding Styles
- HTML Canvas - Adding Text
- HTML Canvas - Adding Images
- HTML Canvas - Canvas Clock
- HTML Canvas - Transformations
- Composting and Clipping
- HTML Canvas - Basic Animations
- Advanced Animations
- HTML Canvas API Functions
- HTML Canvas - Element
- HTML Canvas - Rectangles
- HTML Canvas - Lines
- HTML Canvas - Paths
- HTML Canvas - Text
- HTML Canvas - Colors and Styles
- HTML Canvas - Images
- HTML Canvas - Shadows and Transformations
- HTML Canvas Useful Resources
- HTML Canvas - Quick Guide
- HTML Canvas - Useful Resources
- HTML Canvas - Discussion
HTML Canvas - miterLimit Property
The HTML Canvas miterLimit property of Canvas 2D API can be used set the ratio of miter style applied using lineJoin property.
This property should be applied after starting the path and before drawing the line and is from the CanvasRenderingContext2D interface.
Possible input values
It accepts non-zero integer number values which specifies the miter limit ratio drawn inside the canvas element. The default value is taken as '10.0' by default.
Example
The following example draws simple lines onto the Canvas element after applying the HTML Canvas miterLimit property.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="400" height="200" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.beginPath() context.moveTo(30, 10); context.lineTo(100, 160); context.lineTo(170, 10); context.lineJoin = 'miter'; context.miterLimit = 2; context.lineWidth = 20; context.stroke(); context.closePath(); context.beginPath(); context.moveTo(200, 10); context.lineTo(270, 160); context.lineTo(340, 10); context.miterLimit = 25; context.lineJoin = 'miter'; context.lineWidth = 20; context.stroke(); context.closePath(); </script> </body> </html>
Output
The output returned by the above code on the webpage as −
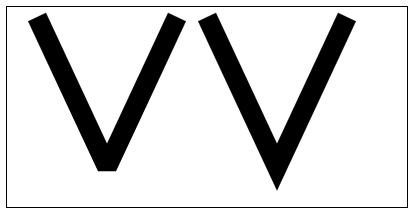
Example
The following program implements miterLimit property on the shape rectangle drawn inside the Canvas element using lines.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="250" height="200" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.beginPath(); context.miterLimit = 20; context.lineJoin = 'miter'; context.lineWidth = 10; context.moveTo(30, 30); context.lineTo(30, 130); context.lineTo(180, 130); context.lineTo(180, 30); context.lineTo(25, 30); context.stroke(); context.closePath(); </script> </body> </html>
Output
The output returned by the above code on the webpage as −
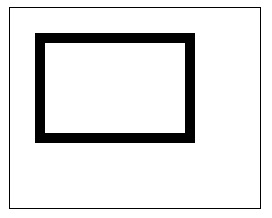
To Continue Learning Please Login