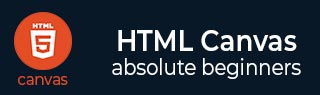
- HTML Canvas Tutorial
- HTML Canvas - Home
- HTML Canvas - Introduction
- Environmental Setup
- HTML Canvas - First Application
- HTML Canvas - Drawing 2D Shapes
- HTML Canvas - Path Elements
- 2D Shapes Using Path Elements
- HTML Canvas - Colors
- HTML Canvas - Adding Styles
- HTML Canvas - Adding Text
- HTML Canvas - Adding Images
- HTML Canvas - Canvas Clock
- HTML Canvas - Transformations
- Composting and Clipping
- HTML Canvas - Basic Animations
- Advanced Animations
- HTML Canvas API Functions
- HTML Canvas - Element
- HTML Canvas - Rectangles
- HTML Canvas - Lines
- HTML Canvas - Paths
- HTML Canvas - Text
- HTML Canvas - Colors and Styles
- HTML Canvas - Images
- HTML Canvas - Shadows and Transformations
- HTML Canvas Useful Resources
- HTML Canvas - Quick Guide
- HTML Canvas - Useful Resources
- HTML Canvas - Discussion
HTML Canvas - isPointInPath() Method
The HTML Canvas isPointInPath() method of the Canvas 2D API checks whether the specified point is contained in the current path and reports it by using Boolean output.
Syntax
Following is the syntax of HTML Canvas isPointInPath() method −
CanvasRenderingContext2D.isPointInPath(x, y, path, rule);
Parameters
Following is the list of parameters of this method −
S.No | Parameter & Description |
---|---|
1 | x
The x co-ordinate of the point to check. |
2 | y
The y co-ordinate of the point to check. |
3 | path
Path which is to be referred to check if the provided point is contained in it or not. If no path is given, current path is used. |
4 | rule
The algorithm to be used to determine whether the point is in path or not. This parameter takes two input values.
|
Return value
When a CanvasRenderingContext2D interface context object access isPointInPath() method, it returns a Boolean value whether the point is in path or not.
Example 1
The following example draws a circle in the Canvas element and checks whether the provided point is in the path or not using HTML Canvas isPointInPath() method.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="200" height="200" style="border: 1px solid black;"></canvas> <p>Check the given point is in the path : <code id="check">false</code> </p> <script> function Context() { var canvas = document.getElementById("canvas"); var context = canvas.getContext("2d"); var check = document.getElementById("check"); context.beginPath(); context.arc(100, 100, 75, 1 * Math.PI, 5 * Math.PI); context.fill(); context.closePath(); check.innerText = context.isPointInPath(150, 150); } </script> </body> </html>
Output
The following code returns output on the webpage as −

Example 2
The following example draws a stroked triangle in the Canvas element and checks whether the provided point is in the path or not.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="200" height="200" style="border: 1px solid black;"></canvas> <p>Check the given point is in the shape : <code id="check">false</code> </p> <script> function Context() { var canvas = document.getElementById("canvas"); var context = canvas.getContext("2d"); var check = document.getElementById("check"); context.strokeStyle = 'blue'; context.beginPath(); context.moveTo(100, 50); context.lineTo(25, 150); context.lineTo(175, 150); context.lineTo(100, 50); context.stroke(); context.closePath(); check.innerText = context.isPointInPath(15, 15); } </script> </body> </html>
Output
The following code returns output on the webpage as −
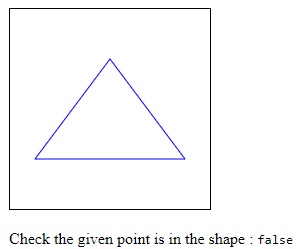
To Continue Learning Please Login