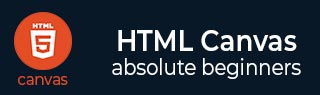
- HTML Canvas Tutorial
- HTML Canvas - Home
- HTML Canvas - Introduction
- Environmental Setup
- HTML Canvas - First Application
- HTML Canvas - Drawing 2D Shapes
- HTML Canvas - Path Elements
- 2D Shapes Using Path Elements
- HTML Canvas - Colors
- HTML Canvas - Adding Styles
- HTML Canvas - Adding Text
- HTML Canvas - Adding Images
- HTML Canvas - Canvas Clock
- HTML Canvas - Transformations
- Composting and Clipping
- HTML Canvas - Basic Animations
- Advanced Animations
- HTML Canvas API Functions
- HTML Canvas - Element
- HTML Canvas - Rectangles
- HTML Canvas - Lines
- HTML Canvas - Paths
- HTML Canvas - Text
- HTML Canvas - Colors and Styles
- HTML Canvas - Images
- HTML Canvas - Shadows and Transformations
- HTML Canvas Useful Resources
- HTML Canvas - Quick Guide
- HTML Canvas - Useful Resources
- HTML Canvas - Discussion
HTML Canvas - imageSmoothingEnabled Property
The HTML Canvas imageSmoothingEnabled property of Canvas API applies image smoothing based on the values passed.
This property is commonly used in pixel optimizations for developing games, it retains the sharpness of image when the property is set to false.
Possible input values
It takes Boolean values 'true' or 'false' and apply the smoothing to the referred image object. The default value is 'true'.
Example
The following example takes an image and applies smoothing to the image with value being 'true' by using HTML Canvas imageSmoothingEnabled property.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="450" height="150" style="border: 1px solid black;background-color: black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); const image = new Image() image.src = 'https://www.tutorialspoint.com/scripts/img/logo-footer.png'; context.drawImage(image, 20, 20, 150, 100); context.imageSmoothingEnabled = true; context.drawImage(image, 250, 20, 150, 100); </script> </body> </html>
Output
The output returned by the image on the webpage as −
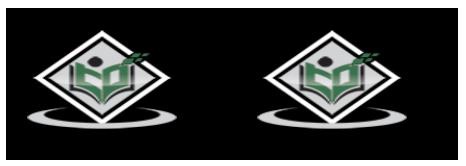
Example
In this example, we take an image and apply the smoothing property as 'false' to the context image object and render it on the Canvas element using imageSmoothingEnabled property.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="670" height="200" style="border: 1px solid black;background-color: black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); const image = new Image(); image.src = 'https://www.tutorialspoint.com/scripts/img/logo-footer.png'; context.drawImage(image, 20, 20, 300, 150); context.imageSmoothingEnabled = false; context.drawImage(image, 350, 20, 300, 150); </script> </body> </html>
Output
The output returned by the image on the webpage as −
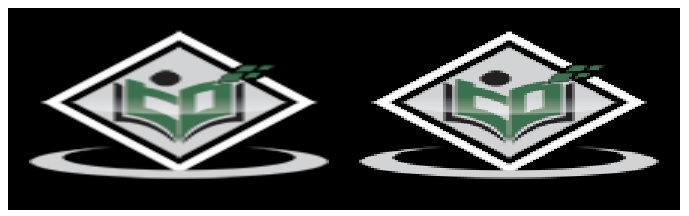
To Continue Learning Please Login