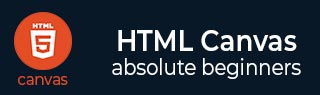
- HTML Canvas Tutorial
- HTML Canvas - Home
- HTML Canvas - Introduction
- Environmental Setup
- HTML Canvas - First Application
- HTML Canvas - Drawing 2D Shapes
- HTML Canvas - Path Elements
- 2D Shapes Using Path Elements
- HTML Canvas - Colors
- HTML Canvas - Adding Styles
- HTML Canvas - Adding Text
- HTML Canvas - Adding Images
- HTML Canvas - Canvas Clock
- HTML Canvas - Transformations
- Composting and Clipping
- HTML Canvas - Basic Animations
- Advanced Animations
- HTML Canvas API Functions
- HTML Canvas - Element
- HTML Canvas - Rectangles
- HTML Canvas - Lines
- HTML Canvas - Paths
- HTML Canvas - Text
- HTML Canvas - Colors and Styles
- HTML Canvas - Images
- HTML Canvas - Shadows and Transformations
- HTML Canvas Useful Resources
- HTML Canvas - Quick Guide
- HTML Canvas - Useful Resources
- HTML Canvas - Discussion
HTML Canvas - globalAlpha Property
The HTML Canvas globalAlpha property can be used to specify the transparency value to be applied to the shapes drawn on the canvas element.
It generally specifies the 'alpha' value so that the shape or image can be made more transparent. This is also used by the 'rgba' color code where "a" is the transparency coefficient.
Possible input values
It takes a decimal number as input in the range '0.0' to '1.0' inclusive where '0.0' indicates full transparency and '1.0' indicates full opacity of the shape.
Example
The following example draws a square onto the Canvas element and applies transparency to it using the HTML Canvas globalAlpha property.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="200" height="100" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.beginPath(); context.rect(50, 10, 75, 75); context.globalAlpha = 0.25; context.fillStyle = 'brown'; context.fill(); context.closePath(); </script> </body> </html>
Output
The output returned by the above code on the webpage as −
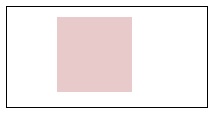
Example
The following example applies transparency to the triangle drawn onto the Canvas element using globalAlpha property.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="200" height="150" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.beginPath(); context.moveTo(100, 50); context.lineTo(50, 100); context.lineTo(150, 100); context.lineTo(100, 50); context.globalAlpha = 0.5; context.fillStyle = 'blue'; context.fill(); context.closePath(); </script> </body> </html>
Output
The output returned by the above code on the webpage as −
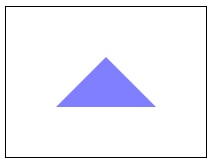
To Continue Learning Please Login