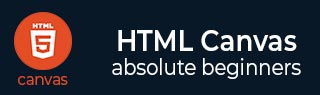
- HTML Canvas Tutorial
- HTML Canvas - Home
- HTML Canvas - Introduction
- Environmental Setup
- HTML Canvas - First Application
- HTML Canvas - Drawing 2D Shapes
- HTML Canvas - Path Elements
- 2D Shapes Using Path Elements
- HTML Canvas - Colors
- HTML Canvas - Adding Styles
- HTML Canvas - Adding Text
- HTML Canvas - Adding Images
- HTML Canvas - Canvas Clock
- HTML Canvas - Transformations
- Composting and Clipping
- HTML Canvas - Basic Animations
- Advanced Animations
- HTML Canvas API Functions
- HTML Canvas - Element
- HTML Canvas - Rectangles
- HTML Canvas - Lines
- HTML Canvas - Paths
- HTML Canvas - Text
- HTML Canvas - Colors and Styles
- HTML Canvas - Images
- HTML Canvas - Shadows and Transformations
- HTML Canvas Useful Resources
- HTML Canvas - Quick Guide
- HTML Canvas - Useful Resources
- HTML Canvas - Discussion
HTML Canvas - Filter Property
The HTML Canvas filter property is used to apply color and contrast effects such as grayscale, sepia, etc to the image drawn onto the canvas element using the context object.
It works exactly as the CSS filter property and takes the same input values. It applies the filter provided with the exact value to the image inside the Canvas element.
Possible input values
The filter property accepts 'none' value which does not return anything. The values accepted by the parameter are given below.
S.No | Value & Description |
---|---|
1 | url()
This value is given when CSS URL is needed to access the filter data and apply to the CanvasRenderingContext2D object. |
2 | blur()
When this value is given as object filter, the object is blurred. Values of input are integers and when zero is given, the object remains unchanged. |
3 | brightness()
This in-built method is used to make the object brighter or darker. If the value given is less than 100%, the object darkens and if the value is above 100%, the object brightens. |
4 | contrast()
This value of filter property is used to adjust the contrast of drawing. It is given in percentage and 0% gives a completely black object on the canvas. |
5 | drop-shadow()
It applies shadow to the object. It takes four values to perform this operation which is the distance of shadow from object, radius, and color of the shadow. |
6 | grayscale()
This value of filter property converts the object into grayscale. It takes percentage values as input. |
7 | hue-rotate()
This value applies hue-rotation onto the drawing. It is given in degrees and 0deg does not alter the drawing. |
8 | invert()
This method inverts the object in the canvas and takes percentage value to perform the operation. |
9 | opacity()
To make the drawing transparent, this method of filter property is called. It also takes percentage value as input. |
10 | saturation()
To saturate the drawing, we apply this filter property to the CanvasRenderingContext2D object, by giving percentage value. |
11 | sepia()
This adds sepia filter to the drawing when called by passing a percentage value indicating the requirement of filter. |
12 | none
No filter is applied and the drawing remains same. |
Example
The following program applies 'brightness' value to the HTML Canvas filter property to the context object created.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="500" height="200" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'brightness(150%)' context.fillStyle = 'cyan'; context.fill(); context.fillRect(50, 20, 150, 100); context.filter = 'brightness(50%)' context.fillStyle = 'cyan'; context.fill(); context.fillRect(250, 20, 150, 100); } </script> </body> </html>
Output
The output returned by the above code on the webpage as −
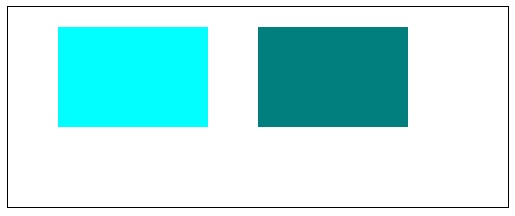
Example
The following program applies blur filter property to the context object created.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="400" height="200" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'blur(3px)'; var image = new Image(); image.onload = function() { context.drawImage(image, 50, 50); }; image.src = 'https://www.tutorialspoint.com/html5/images/logo.png'; } </script> </body> </html>
Output
The output returned by the above code on the webpage as −
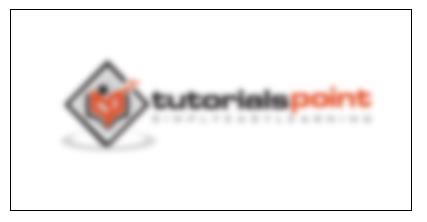
Example
The following program applies contrast filter property to the context object created.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="200" height="200" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'contrast(30%)'; context.beginPath(); context.arc(100, 100, 50, 1 * Math.PI, 5 * Math.PI); context.fill(); context.closePath(); } </script> </body> </html>
Output
The output returned by the above code on the webpage as −
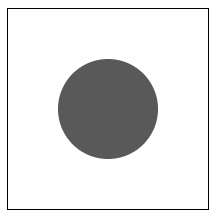
Example
The following program applies drop-shadow filter property to the context object created.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="400" height="200" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'drop-shadow(20px 10px grey)'; var image = new Image(); image.onload = function() { context.drawImage(image, 50, 50); }; image.src = 'https://www.tutorialspoint.com/html5/images/logo.png'; } </script> </body> </html>
Output
The output returned by the above code on the webpage as −
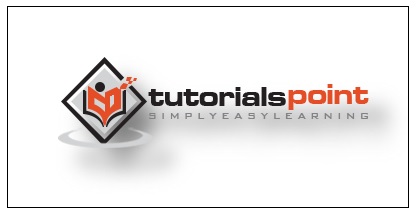
Example
The following program applies grayscale filter property to the context object created.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="400" height="200" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'grayscale(125%)'; var image = new Image(); image.onload = function() { context.drawImage(image, 50, 50); }; image.src = 'https://www.tutorialspoint.com/html5/images/logo.png'; } </script> </body> </html>
Output
The output returned by the above code on the webpage as −
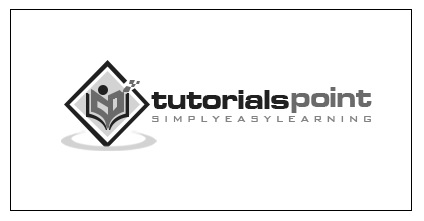
Example
The following program applies hue-rotate filter property to the context object created.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="625" height="425" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'hue-rotate(45deg)'; var image = new Image(); image.onload = function() { context.drawImage(image, 10, 10); }; image.src = '../../images/image3.png'; } </script> </body> </html>
Output
The image used in this program is −
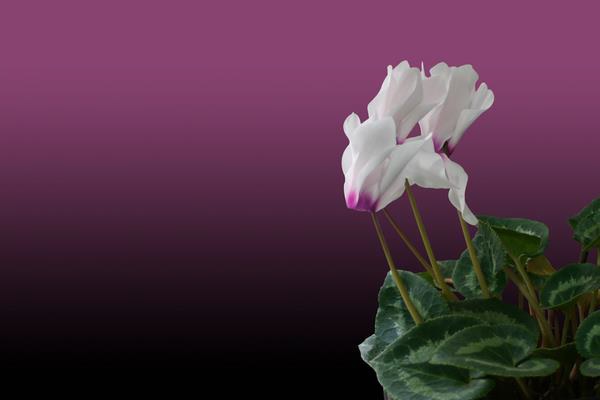
The output returned by the above code on the webpage as −
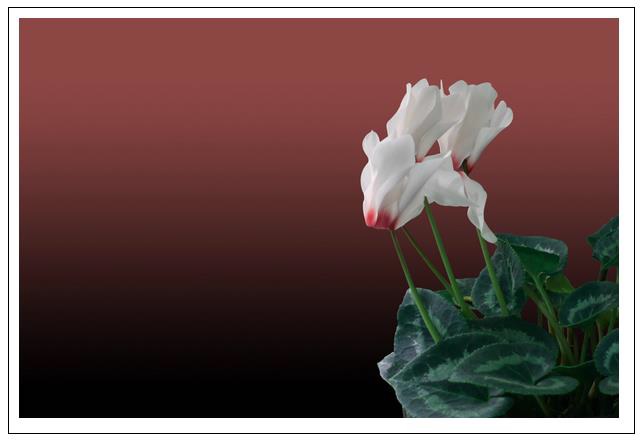
Example
The following program applies invert filter property to the context object created.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="625" height="425" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'invert(100%)'; var image = new Image(); image.onload = function() { context.drawImage(image, 10, 10); }; image.src = '../../images/image7.png'; } </script> </body> </html>
Output
The image used in this program is −
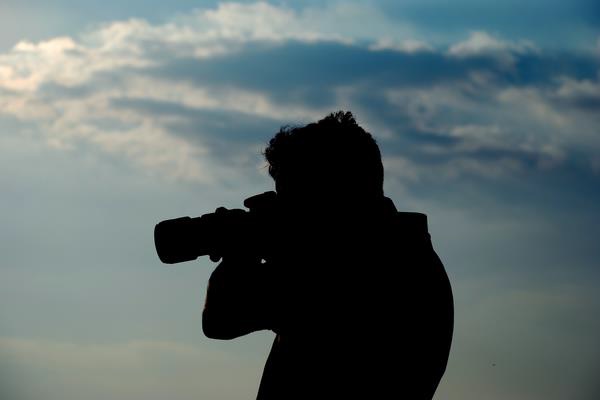
The output returned by the above code on the webpage as −
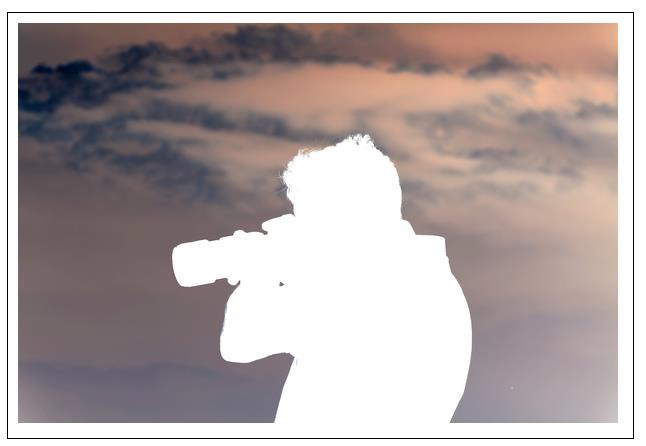
Example
The following program applies opacity filter property to the context object created.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload = "Context();"> <canvas id="canvas" width="625" height="425" style="border: 1px solid black;"></canvas> <script> function Context(){ var canvas=document.getElementById('canvas'); var context=canvas.getContext('2d'); context.filter = 'opacity(75%)'; var image = new Image(); image.onload = function() { context.drawImage(image, 10, 10); }; image.src = '../../images/image33.png'; } </script> </body> </html>
Output
The image used in this program is −
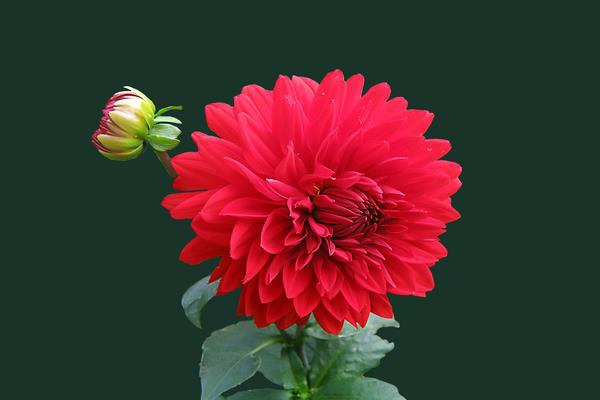
The output returned by the above code on the webpage as −
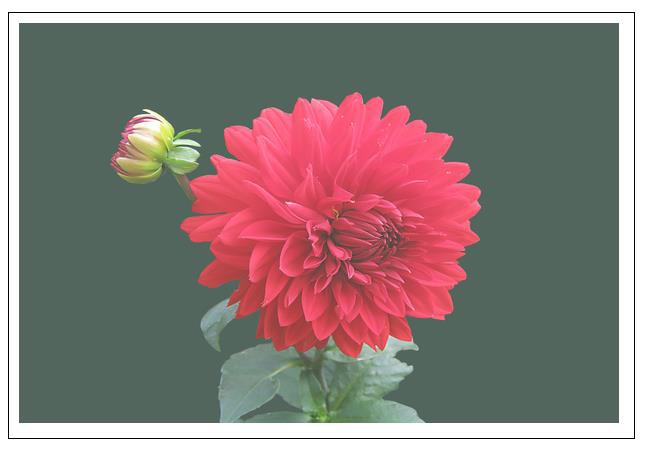
Example
The following program applies saturation filter property to the context object created.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload = "Context();"> <canvas id="canvas" width="625" height="425" style="border: 1px solid black;"></canvas> <script> function Context(){ var canvas=document.getElementById('canvas'); var context=canvas.getContext('2d'); context.filter = 'saturate(250%)'; var image = new Image(); image.onload = function() { context.drawImage(image, 10, 10); }; image.src = '../../images/image66.png'; } </script> </body> </html>
Output
The image used in this program is
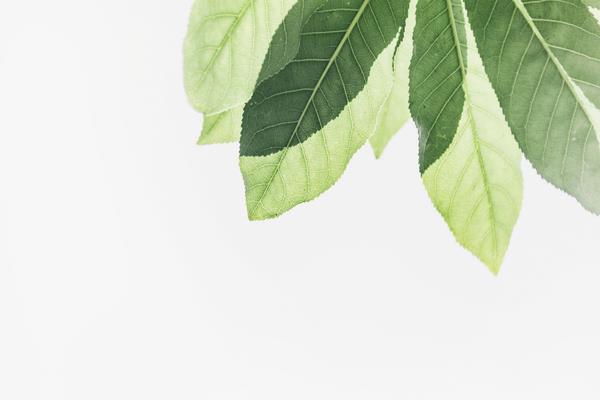
The output returned by the above code on the webpage as −
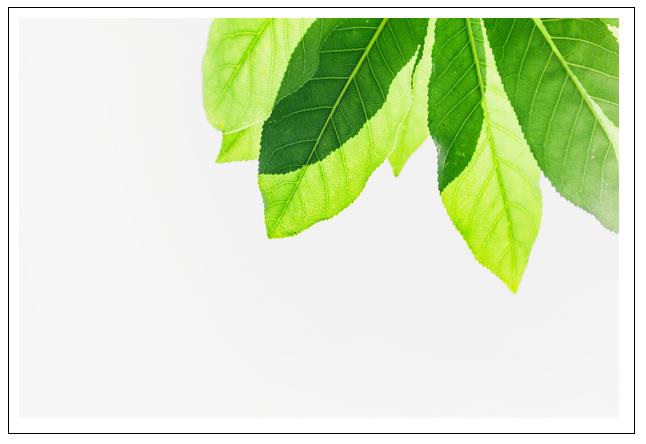
Example
The following program applies sepia filter property to the context object created.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="625" height="425" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'sepia(95%)'; var image = new Image(); image.onload = function() { context.drawImage(image, 10, 10); }; image.src = '../../images/image77.png'; } </script> </body> </html>
Output
The image used in this program is −
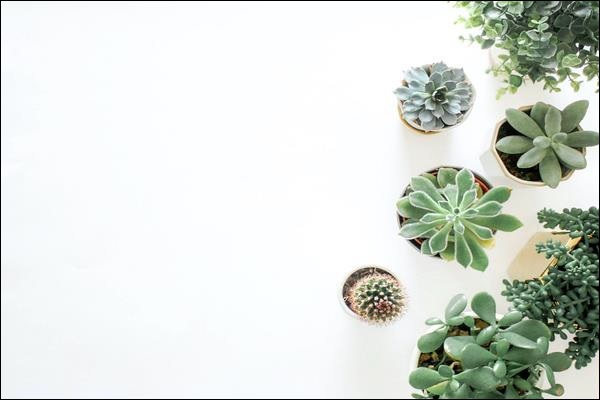
The output returned by the above code on the webpage as −
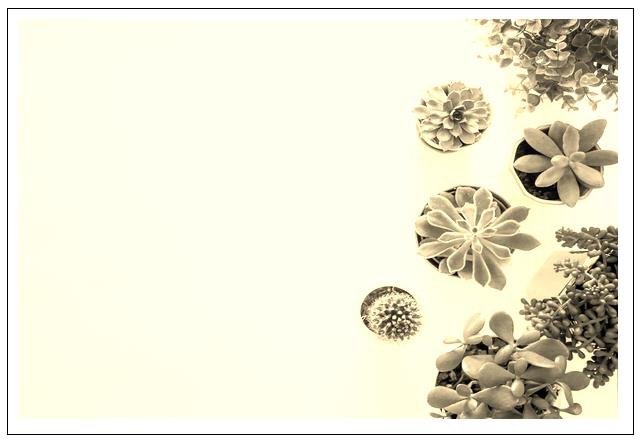
To Continue Learning Please Login