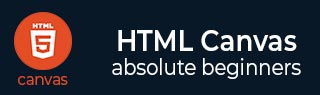
- HTML Canvas Tutorial
- HTML Canvas - Home
- HTML Canvas - Introduction
- Environmental Setup
- HTML Canvas - First Application
- HTML Canvas - Drawing 2D Shapes
- HTML Canvas - Path Elements
- 2D Shapes Using Path Elements
- HTML Canvas - Colors
- HTML Canvas - Adding Styles
- HTML Canvas - Adding Text
- HTML Canvas - Adding Images
- HTML Canvas - Canvas Clock
- HTML Canvas - Transformations
- Composting and Clipping
- HTML Canvas - Basic Animations
- Advanced Animations
- HTML Canvas API Functions
- HTML Canvas - Element
- HTML Canvas - Rectangles
- HTML Canvas - Lines
- HTML Canvas - Paths
- HTML Canvas - Text
- HTML Canvas - Colors and Styles
- HTML Canvas - Images
- HTML Canvas - Shadows and Transformations
- HTML Canvas Useful Resources
- HTML Canvas - Quick Guide
- HTML Canvas - Useful Resources
- HTML Canvas - Discussion
HTML Canvas - fillStyle Property
The HTML Canvas fillStyle property of Canvas 2D API is from the interface CanvasRenderingContext2D takes the context object shape and fills it with the passed color value.
It mainly specifies the color, gradient, or pattern to use inside any shape. The color is 'black' by default.
Possible input values
The property accepts any one of the following values −
Any format of CSS color value.
A gradient object for adding inside the shape.
A pattern object for creating a repeated pattern inside the shape.
Example
The following program draws a rectangle inside the Canvas element and fills using the HTML Canvas fillStyle property.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="300" height="250" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.fillStyle = 'blue'; context.rect(35, 35, 200, 150); context.fill(); </script> </body> </html>
Output
The output returned by the above code on the webpage as −
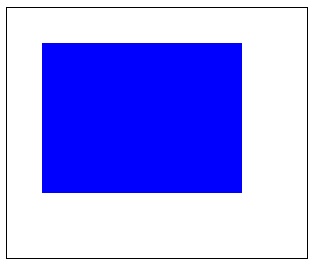
Example
The following program draws a rectangle inside the Canvas element and fills a pattern created using the fillStyle property.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="300" height="250" style="border: 1px solid black; background-color: blue;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d') var image = new Image(); image.src = 'https://www.tutorialspoint.com/themes/home/tp-diamond-logo-white.png'; image.onload = function() { var pattern = context.createPattern(image, 'repeat'); context.fillStyle = pattern; context.fillRect(0, 0, canvas.width, canvas.height); } </script> </body> </html>
Output
The output returned by the above code on the webpage as −
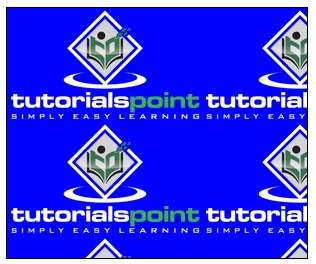
Example
The following program draws a rectangle inside the Canvas element and fills a gradient object using the fillStyle property.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="300" height="250" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById("canvas"); var context = canvas.getContext('2d'); var lineargrad = context.createLinearGradient(0, 0, 200, 100); context.fillStyle = lineargrad; lineargrad.addColorStop(0, 'cyan'); lineargrad.addColorStop(0.5, 'white'); lineargrad.addColorStop(1, 'purple'); context.fillRect(10, 10, 250, 200); </script> </body> </html>
Output
The output returned by the above code on the webpage as −
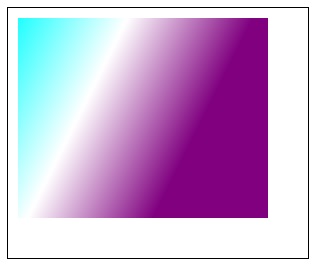
To Continue Learning Please Login