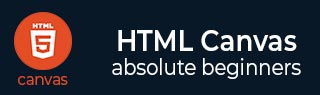
- HTML Canvas Tutorial
- HTML Canvas - Home
- HTML Canvas - Introduction
- Environmental Setup
- HTML Canvas - First Application
- HTML Canvas - Drawing 2D Shapes
- HTML Canvas - Path Elements
- 2D Shapes Using Path Elements
- HTML Canvas - Colors
- HTML Canvas - Adding Styles
- HTML Canvas - Adding Text
- HTML Canvas - Adding Images
- HTML Canvas - Canvas Clock
- HTML Canvas - Transformations
- Composting and Clipping
- HTML Canvas - Basic Animations
- Advanced Animations
- HTML Canvas API Functions
- HTML Canvas - Element
- HTML Canvas - Rectangles
- HTML Canvas - Lines
- HTML Canvas - Paths
- HTML Canvas - Text
- HTML Canvas - Colors and Styles
- HTML Canvas - Images
- HTML Canvas - Shadows and Transformations
- HTML Canvas Useful Resources
- HTML Canvas - Quick Guide
- HTML Canvas - Useful Resources
- HTML Canvas - Discussion
HTML Canvas - fill() Method
The HTML Canvas fill() method of Canvas 2D API is used to fill the current path or the given path inside the Canvas element.
It fills the path using black color by default which can be changed using the color passed using the fillStyle property.
Syntax
Following is the syntax of HTML Canvas fill() method −
CanvasRenderingContext2D.fill(path, fillrule)
Parameters
Following is the list of parameters of this method −
S.No | Parameter & Description |
---|---|
1 | path
Path to be used to apply for the fill() method. |
2 | fillrule
The algorithm to apply to check the point is in or out of the clipping region. The possible values for this method are &minud;
|
Return Value
The given path is filled using the color passed by fillStyle property or 'black' by default.
Example 1
The following example draws a rhombus onto the Canvas element and fills it using HTML Canvas fill() method.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="220" height="200" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.beginPath(); context.moveTo(100, 50); context.lineTo(50, 100); context.lineTo(100, 150); context.lineTo(150, 100); context.closePath(); context.fill(); </script> </body> </html>
Output
The output returned by the above code on the webpage as −
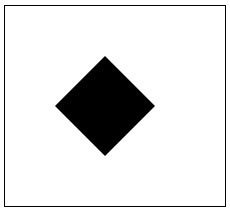
Example 2
The following example draws shapes on the Canvas element and fills them accordingly using fillrule algorithms.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="340" height="340" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.rect(20, 20, 300, 300); context.rect(50, 50, 200, 200); context.rect(80, 80, 100, 100); context.lineWidth = 5; context.stroke(); context.fillStyle = 'blue'; context.fill('evenodd'); context.closePath(); </script> </body> </html>
Output
The output returned by the above code on the webpage as −
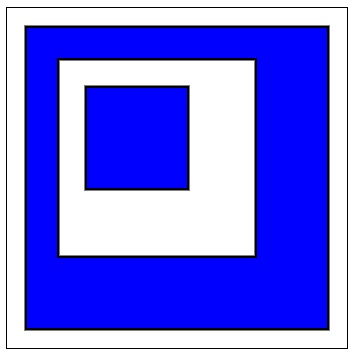
To Continue Learning Please Login