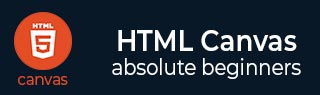
- HTML Canvas Tutorial
- HTML Canvas - Home
- HTML Canvas - Introduction
- Environmental Setup
- HTML Canvas - First Application
- HTML Canvas - Drawing 2D Shapes
- HTML Canvas - Path Elements
- 2D Shapes Using Path Elements
- HTML Canvas - Colors
- HTML Canvas - Adding Styles
- HTML Canvas - Adding Text
- HTML Canvas - Adding Images
- HTML Canvas - Canvas Clock
- HTML Canvas - Transformations
- Composting and Clipping
- HTML Canvas - Basic Animations
- Advanced Animations
- HTML Canvas API Functions
- HTML Canvas - Element
- HTML Canvas - Rectangles
- HTML Canvas - Lines
- HTML Canvas - Paths
- HTML Canvas - Text
- HTML Canvas - Colors and Styles
- HTML Canvas - Images
- HTML Canvas - Shadows and Transformations
- HTML Canvas Useful Resources
- HTML Canvas - Quick Guide
- HTML Canvas - Useful Resources
- HTML Canvas - Discussion
HTML Canvas - bezierCurveTo() Method
The HTML Canvas bezierCurveTo() method of CanvasRenderingContext2D interface can be used to draw a cubic Bezier curve onto the current path.
It takes two control points and another end point as parameters to take as reference and draws the Bezier cubic curve onto the Canvas element.
Syntax
Following is the syntax of HTML Canvas bezierCurveTo() method −
CanvasRenderingContext2D.bezierCurveTo(p1x, p1y, p2x, p2y, x, y);
Parameters
Following is the list of parameters of this method −
S.No | Parameter & Description |
---|---|
1 | p1x
x co-ordinate of the first control point. |
2 | p1y
y co-ordinate of the first control point. |
3 | p2x
x co-ordinate of the second control point. |
4 | p2y
y co-ordinate of the second control point. |
5 | x
x co-ordinate of the end point. |
6 | y
y co-ordinate of the end point. |
Return value
A cubic Bezier curve is drawn when the method is called by the context object of CanvasRenderingContext2D interface of the Canvas element.
Example
The following example draws a simple cubic curve by using the HTML Canvas bezierCurveTo() method.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="250" height="100" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.lineWidth = 5; context.beginPath(); context.lineWidth = 10; context.moveTo(15, 15); context.bezierCurveTo(20, 110, 180, 90, 230, 20); context.stroke(); context.closePath(); </script> </body> </html>
Output
The output returned by the above code on the webpage as −
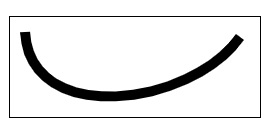
Example
The following example draws a Bezier curve by taking the control points and contact points passed as parameters to the bezierCurveTo() method.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="500" height="200" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.lineWidth = 5; context.beginPath(); context.moveTo(100, 100); context.bezierCurveTo(150, 15, 300, 150, 350, 75); context.stroke(); context.closePath(); </script> </body> </html>
Output
The output returned by the above code on the webpage as −
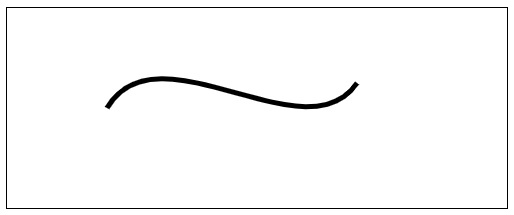
Example
The following example draws a Bezier cubic curve by taking the control points and contact points passed as parameters to the bezierCurveTo() method. The code to achieve this is given below.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="300" height="200" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.lineWidth = 5; context.beginPath(); context.strokeStyle = 'blue'; context.moveTo(25, 25); context.bezierCurveTo(100, 150, 150, 25, 250, 150); context.stroke(); context.closePath(); </script> </body> </html>
Output
The output returned by the above code on the webpage as −
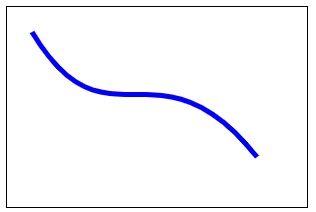
To Continue Learning Please Login