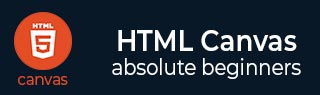
- HTML Canvas Tutorial
- HTML Canvas - Home
- HTML Canvas - Introduction
- Environmental Setup
- HTML Canvas - First Application
- HTML Canvas - Drawing 2D Shapes
- HTML Canvas - Path Elements
- 2D Shapes Using Path Elements
- HTML Canvas - Colors
- HTML Canvas - Adding Styles
- HTML Canvas - Adding Text
- HTML Canvas - Adding Images
- HTML Canvas - Canvas Clock
- HTML Canvas - Transformations
- Composting and Clipping
- HTML Canvas - Basic Animations
- Advanced Animations
- HTML Canvas API Functions
- HTML Canvas - Element
- HTML Canvas - Rectangles
- HTML Canvas - Lines
- HTML Canvas - Paths
- HTML Canvas - Text
- HTML Canvas - Colors and Styles
- HTML Canvas - Images
- HTML Canvas - Shadows and Transformations
- HTML Canvas Useful Resources
- HTML Canvas - Quick Guide
- HTML Canvas - Useful Resources
- HTML Canvas - Discussion
HTML Canvas - beginPath() Method
The HTML Canvas beginPath() method of CanvasRenderingContext2D interface creates an empty path in the Canvas element to draw the shapes.
This is only called when a new path is needed on the Canvas context. If the paths to be drawn are multiple, we have to use the same number of beginPath() methods.
Syntax
Following is the syntax of HTML Canvas beginPath() method −
CanvasRenderingContext2D.beginPath();
Parameters
It does not take any parameters as it is a return method only which performs the single task to create an empty path.
Return value
It creates an empty path which can be used to draw various shapes inside the Canvas element. It does not directly return anything rather than provides a path.
Example
In the following example, we use HTML Canvas beginPath() method to create an empty path and draw simple lines on it.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="250" height="200" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.beginPath(); context.moveTo(150, 50); context.lineTo(150, 120); context.lineTo(50, 190); context.stroke(); </script> </body> </html>
Output
The output returned by the following code on the webpage as −
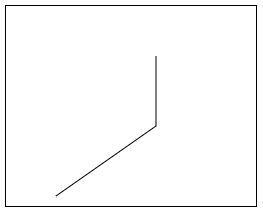
Example
The following example draws a right angled triangle using lines inside the Canvas element after creating the path by beginPath() method.
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body> <canvas id="canvas" width="250" height="200" style="border: 1px solid black;"></canvas> <script> var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.beginPath(); context.moveTo(50, 50); context.lineTo(50, 150); context.lineTo(150, 50); context.lineTo(50, 50); context.stroke(); </script> </body> </html>
Output
The output returned by the above code on the webpage as −
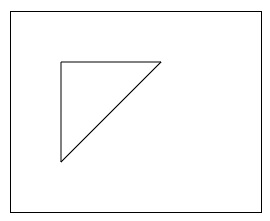
To Continue Learning Please Login