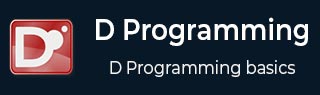
- D Programming Basics
- D Programming - Home
- D Programming - Overview
- D Programming - Environment
- D Programming - Basic Syntax
- D Programming - Variables
- D Programming - Data Types
- D Programming - Enums
- D Programming - Literals
- D Programming - Operators
- D Programming - Loops
- D Programming - Decisions
- D Programming - Functions
- D Programming - Characters
- D Programming - Strings
- D Programming - Arrays
- D Programming - Associative Arrays
- D Programming - Pointers
- D Programming - Tuples
- D Programming - Structs
- D Programming - Unions
- D Programming - Ranges
- D Programming - Aliases
- D Programming - Mixins
- D Programming - Modules
- D Programming - Templates
- D Programming - Immutables
- D Programming - File I/O
- D Programming - Concurrency
- D Programming - Exception Handling
- D Programming - Contract
- D - Conditional Compilation
- D Programming - Object Oriented
- D Programming - Classes & Objects
- D Programming - Inheritance
- D Programming - Overloading
- D Programming - Encapsulation
- D Programming - Interfaces
- D Programming - Abstract Classes
- D Programming - Useful Resources
- D Programming - Quick Guide
- D Programming - Useful Resources
- D Programming - Discussion
D Programming - Tuples
Tuples are used for combining multiple values as a single object. Tuples contains a sequence of elements. The elements can be types, expressions, or aliases. The number and elements of a tuple are fixed at compile time and they cannot be changed at run time.
Tuples have characteristics of both structs and arrays. The tuple elements can be of different types like structs. The elements can be accessed via indexing like arrays. They are implemented as a library feature by the Tuple template from the std.typecons module. Tuple makes use of TypeTuple from the std.typetuple module for some of its operations.
Tuple Using tuple()
Tuples can be constructed by the function tuple(). The members of a tuple are accessed by index values. An example is shown below.
Example
import std.stdio; import std.typecons; void main() { auto myTuple = tuple(1, "Tuts"); writeln(myTuple); writeln(myTuple[0]); writeln(myTuple[1]); }
When the above code is compiled and executed, it produces the following result −
Tuple!(int, string)(1, "Tuts") 1 Tuts
Tuple using Tuple Template
Tuple can also be constructed directly by the Tuple template instead of the tuple() function. The type and the name of each member are specified as two consecutive template parameters. It is possible to access the members by properties when created using templates.
import std.stdio; import std.typecons; void main() { auto myTuple = Tuple!(int, "id",string, "value")(1, "Tuts"); writeln(myTuple); writeln("by index 0 : ", myTuple[0]); writeln("by .id : ", myTuple.id); writeln("by index 1 : ", myTuple[1]); writeln("by .value ", myTuple.value); }
When the above code is compiled and executed, it produces the following result
Tuple!(int, "id", string, "value")(1, "Tuts") by index 0 : 1 by .id : 1 by index 1 : Tuts by .value Tuts
Expanding Property and Function Params
The members of Tuple can be expanded either by the .expand property or by slicing. This expanded/sliced value can be passed as function argument list. An example is shown below.
Example
import std.stdio; import std.typecons; void method1(int a, string b, float c, char d) { writeln("method 1 ",a,"\t",b,"\t",c,"\t",d); } void method2(int a, float b, char c) { writeln("method 2 ",a,"\t",b,"\t",c); } void main() { auto myTuple = tuple(5, "my string", 3.3, 'r'); writeln("method1 call 1"); method1(myTuple[]); writeln("method1 call 2"); method1(myTuple.expand); writeln("method2 call 1"); method2(myTuple[0], myTuple[$-2..$]); }
When the above code is compiled and executed, it produces the following result −
method1 call 1 method 1 5 my string 3.3 r method1 call 2 method 1 5 my string 3.3 r method2 call 1 method 2 5 3.3 r
TypeTuple
TypeTuple is defined in the std.typetuple module. A comma-separated list of values and types. A simple example using TypeTuple is given below. TypeTuple is used to create argument list, template list, and array literal list.
import std.stdio; import std.typecons; import std.typetuple; alias TypeTuple!(int, long) TL; void method1(int a, string b, float c, char d) { writeln("method 1 ",a,"\t",b,"\t",c,"\t",d); } void method2(TL tl) { writeln(tl[0],"\t", tl[1] ); } void main() { auto arguments = TypeTuple!(5, "my string", 3.3,'r'); method1(arguments); method2(5, 6L); }
When the above code is compiled and executed, it produces the following result −
method 1 5 my string 3.3 r 5 6