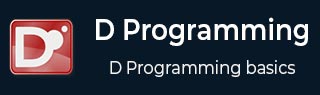
- D Programming Basics
- D Programming - Home
- D Programming - Overview
- D Programming - Environment
- D Programming - Basic Syntax
- D Programming - Variables
- D Programming - Data Types
- D Programming - Enums
- D Programming - Literals
- D Programming - Operators
- D Programming - Loops
- D Programming - Decisions
- D Programming - Functions
- D Programming - Characters
- D Programming - Strings
- D Programming - Arrays
- D Programming - Associative Arrays
- D Programming - Pointers
- D Programming - Tuples
- D Programming - Structs
- D Programming - Unions
- D Programming - Ranges
- D Programming - Aliases
- D Programming - Mixins
- D Programming - Modules
- D Programming - Templates
- D Programming - Immutables
- D Programming - File I/O
- D Programming - Concurrency
- D Programming - Exception Handling
- D Programming - Contract
- D - Conditional Compilation
- D Programming - Object Oriented
- D Programming - Classes & Objects
- D Programming - Inheritance
- D Programming - Overloading
- D Programming - Encapsulation
- D Programming - Interfaces
- D Programming - Abstract Classes
- D Programming - Useful Resources
- D Programming - Quick Guide
- D Programming - Useful Resources
- D Programming - Discussion
D Programming - Mixins
Mixins are structs that allow mixing of the generated code into the source code. Mixins can be of the following types −
- String Mixins
- Template Mixins
- Mixin name spaces
String Mixins
D has the capability to insert code as string as long as that string is known at compile time. The syntax of string mixins is shown below −
mixin (compile_time_generated_string)
Example
A simple example for string mixins is shown below.
import std.stdio; void main() { mixin(`writeln("Hello World!");`); }
When the above code is compiled and executed, it produces the following result −
Hello World!
Here is another example where we can pass the string in compile time so that mixins can use the functions to reuse code. It is shown below.
import std.stdio; string print(string s) { return `writeln("` ~ s ~ `");`; } void main() { mixin (print("str1")); mixin (print("str2")); }
When the above code is compiled and executed, it produces the following result −
str1 str2
Template Mixins
D templates define common code patterns, for the compiler to generate actual instances from that pattern. The templates can generate functions, structs, unions, classes, interfaces, and any other legal D code. The syntax of template mixins is as shown below.
mixin a_template!(template_parameters)
A simple example for string mixins is shown below where we create a template with class Department and a mixin instantiating a template and hence making the the functions setName and printNames available to the structure college.
Example
import std.stdio; template Department(T, size_t count) { T[count] names; void setName(size_t index, T name) { names[index] = name; } void printNames() { writeln("The names"); foreach (i, name; names) { writeln(i," : ", name); } } } struct College { mixin Department!(string, 2); } void main() { auto college = College(); college.setName(0, "name1"); college.setName(1, "name2"); college.printNames(); }
When the above code is compiled and executed, it produces the following result −
The names 0 : name1 1 : name2
Mixin Name Spaces
Mixin name spaces are used to avoid ambiguities in template mixins. For example, there can be two variables, one defined explicitly in main and the other is mixed in. When a mixed-in name is the same as a name that is in the surrounding scope, then the name that is in the surrounding scope gets used. This example is shown below.
Example
import std.stdio; template Person() { string name; void print() { writeln(name); } } void main() { string name; mixin Person a; name = "name 1"; writeln(name); a.name = "name 2"; print(); }
When the above code is compiled and executed, it produces the following result −
name 1 name 2