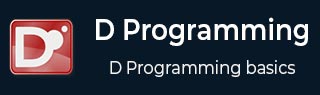
- D Programming Basics
- D Programming - Home
- D Programming - Overview
- D Programming - Environment
- D Programming - Basic Syntax
- D Programming - Variables
- D Programming - Data Types
- D Programming - Enums
- D Programming - Literals
- D Programming - Operators
- D Programming - Loops
- D Programming - Decisions
- D Programming - Functions
- D Programming - Characters
- D Programming - Strings
- D Programming - Arrays
- D Programming - Associative Arrays
- D Programming - Pointers
- D Programming - Tuples
- D Programming - Structs
- D Programming - Unions
- D Programming - Ranges
- D Programming - Aliases
- D Programming - Mixins
- D Programming - Modules
- D Programming - Templates
- D Programming - Immutables
- D Programming - File I/O
- D Programming - Concurrency
- D Programming - Exception Handling
- D Programming - Contract
- D - Conditional Compilation
- D Programming - Object Oriented
- D Programming - Classes & Objects
- D Programming - Inheritance
- D Programming - Overloading
- D Programming - Encapsulation
- D Programming - Interfaces
- D Programming - Abstract Classes
- D Programming - Useful Resources
- D Programming - Quick Guide
- D Programming - Useful Resources
- D Programming - Discussion
D Programming - Classes & Objects
Classes are the central feature of D programming that supports object-oriented programming and are often called user-defined types.
A class is used to specify the form of an object and it combines data representation and methods for manipulating that data into one neat package. The data and functions within a class are called members of the class.
D Class Definitions
When you define a class, you define a blueprint for a data type. This does not actually define any data, but it defines what the class name means, that is, what an object of the class will consist of and what operations can be performed on such an object.
A class definition starts with the keyword class followed by the class name; and the class body, enclosed by a pair of curly braces. A class definition must be followed either by a semicolon or a list of declarations. For example, we defined the Box data type using the keyword class as follows −
class Box { public: double length; // Length of a box double breadth; // Breadth of a box double height; // Height of a box }
The keyword public determines the access attributes of the members of the class that follow it. A public member can be accessed from outside the class anywhere within the scope of the class object. You can also specify the members of a class as private or protected which we will discuss in a sub-section.
Defining D Objects
A class provides the blueprints for objects, so basically an object is created from a class. You declare objects of a class with exactly the same sort of declaration that you declare variables of basic types. The following statements declare two objects of class Box −
Box Box1; // Declare Box1 of type Box Box Box2; // Declare Box2 of type Box
Both of the objects Box1 and Box2 have their own copy of data members.
Accessing the Data Members
The public data members of objects of a class can be accessed using the direct member access operator (.). Let us try the following example to make the things clear −
import std.stdio; class Box { public: double length; // Length of a box double breadth; // Breadth of a box double height; // Height of a box } void main() { Box box1 = new Box(); // Declare Box1 of type Box Box box2 = new Box(); // Declare Box2 of type Box double volume = 0.0; // Store the volume of a box here // box 1 specification box1.height = 5.0; box1.length = 6.0; box1.breadth = 7.0; // box 2 specification box2.height = 10.0; box2.length = 12.0; box2.breadth = 13.0; // volume of box 1 volume = box1.height * box1.length * box1.breadth; writeln("Volume of Box1 : ",volume); // volume of box 2 volume = box2.height * box2.length * box2.breadth; writeln("Volume of Box2 : ", volume); }
When the above code is compiled and executed, it produces the following result −
Volume of Box1 : 210 Volume of Box2 : 1560
It is important to note that private and protected members can not be accessed directly using direct member access operator (.). Shortly you will learn how private and protected members can be accessed.
Classes and Objects in D
So far, you have got very basic idea about D Classes and Objects. There are further interesting concepts related to D Classes and Objects which we will discuss in various sub-sections listed below −
Sr.No. | Concept & Description |
---|---|
1 | Class member functions
A member function of a class is a function that has its definition or its prototype within the class definition like any other variable. |
2 | Class access modifiers
A class member can be defined as public, private or protected. By default members would be assumed as private. |
3 | Constructor & destructor
A class constructor is a special function in a class that is called when a new object of the class is created. A destructor is also a special function which is called when created object is deleted. |
4 | The this pointer in D
Every object has a special pointer this which points to the object itself. |
5 | Pointer to D classes
A pointer to a class is done exactly the same way a pointer to a structure is. In fact a class is really just a structure with functions in it. |
6 | Static members of a class
Both data members and function members of a class can be declared as static. |