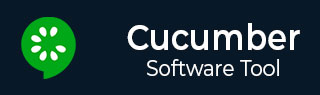
- Cucumber Tutorial
- Cucumber - Home
- Cucumber - Overview
- Cucumber - Environment
- Cucumber - Gherkins
- Cucumber - Features
- Cucumber - Scenarios
- Cucumber - Annotations
- Cucumber - Scenario Outline
- Cucumber - Tags
- Cucumber - Data Tables
- Cucumber - Comments
- Cucumber - Hooks
- Cucumber - Command Line Options
- Cucumber - JUnit Runner
- Cucumber - Reports
- Cucumber - Debugging
- Cucumber - Java Testing
- Cucumber - Ruby Testing
- Cucumber Useful Resources
- Cucumber - Quick Guide
- Cucumber - Useful Resources
- Cucumber - Discussion
Cucumber - Features
A Feature can be defined as a standalone unit or functionality of a project. Let’s take a very common example of a social networking site. How does the feature of this product/project look like? Few basic features can be determined as −
Create and remove the user from the social networking site.
User login functionality for the social networking site.
Sharing photos or videos on the social networking site.
Sending a friend request.
Logout.
By now, it is clear that, each independent functionality of the product under test can be termed as a feature when we talk about Cucumber. It is a best practice later when you start testing, that before deriving the test scripts, we should determine the features to be tested.
A feature usually contains a list of scenarios to be tested for that feature. A file in which we store features, description about the features and scenarios to be tested is known as Feature File. We will see more about feature files in the following chapter.
The keyword to represent a feature under test in Gherkins is “Feature”. The suggested best practice is, to write a small description of the feature beneath the feature title in the feature file. This will fulfill the need of a good documentation as well.
Example
Feature − Login functionality for a social networking site.
The user should be able to login into the social networking site if the username and the password are correct.
The user should be shown the error message if the username and the password are incorrect.
The user should be navigated to home page, if the username and password are correct.
Feature Files
The file, in which Cucumber tests are written, is known as feature files. It is advisable that there should be a separate feature file, for each feature under test. The extension of the feature file needs to be “.feature”.
One can create as many feature files as needed. To have an organized structure, each feature should have one feature file.
For Example −
Sr.No | Feature | Feature File name |
---|---|---|
1 | User Login | userLogin.feature |
2 | Share the Post | sharePost.feature |
3 | Create Account | createAccount.feature |
4 | Delete Account | deleteAccount.feature |
The naming convention to be used for feature name, feature file name depends on the individual’s choice. There is no ground rule in Cucumber about names.
A simple feature file consists of the following keywords/parts −
Feature − Name of the feature under test.
Description (optional) − Describe about feature under test.
Scenario − What is the test scenario.
Given − Prerequisite before the test steps get executed.
When − Specific condition which should match in order to execute the next step.
Then − What should happen if the condition mentioned in WHEN is satisfied.
Example
Feature − User login on social networking site.
The user should be able to login into the social networking site when the username and the password are correct.
The user should be shown an error message when the username and the password are incorrect.
The user should be navigated to the home page if the username and the password are correct.
Outline − Login functionality for a social networking site.
The given user navigates to Facebook. When I enter Username as "<username>" and Password as "<password>". Then, login should be unsuccessful.
| username | password | | username1 | password1 |
* AND keyword is used to show conjunction between two conditions. AND can be used with any other keywords like GIVEN, WHEN and THEN.
There are no logic details written in the feature file.
Steps Definitions
We have got our feature file ready with the test scenarios defined. However, this is not the complete job done. Cucumber doesn’t really know which piece of code is to be executed for any specific scenario outlined in a feature file.
This calls the need of an intermediate – Step Definition file. Steps definition file stores the mapping between each step of the scenario defined in the feature file with a code of function to be executed.
So, now when Cucumber executes a step of the scenario mentioned in the feature file, it scans the step definition file and figures out which function is to be called.
Example of Step Definition File
public void goToFacebook() { driver = new FirefoxDriver(); driver.navigate().to("https://www.facebook.com/"); } @When "^user logs in using Username as \"([^\"]*)\" and Password as \"([^\"]*)\"$" public void I_enter_Username_as_and_Password_as(String arg1, String arg2) { driver.findElement(By.id("email")).sendKeys(arg1); driver.findElement(By.id("pass")).sendKeys(arg2); driver.findElement(By.id("u_0_v")).click(); } @Then"^login should be unsuccessful$" public void validateRelogin() { if(driver.getCurrentUrl().equalsIgnoreCase( "https://www.facebook.com/login.php?login_attempt=1&lwv=110")){ System.out.println("Test Pass"); } else { System.out.println("Test Failed"); } driver.close(); }
So with each function, whatever code you want to execute with each test step (i.e. GIVEN/THEN/WHEN), you can write it within Step Definition file. Make sure that code/function has been defined for each of the steps.
This function can be Java functions, where we can use both Java and Selenium commands in order to automate our test steps.