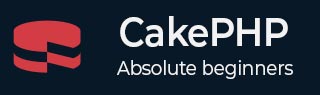
- CakePHP Tutorial
- CakePHP - Home
- CakePHP - Overview
- CakePHP - Installation
- CakePHP - Folder Structure
- CakePHP - Project Configuration
- CakePHP - Routing
- CakePHP - Controllers
- CakePHP - Views
- CakePHP - Extending Views
- CakePHP - View Elements
- CakePHP - View Events
- CakePHP - Working with Database
- CakePHP - View a Record
- CakePHP - Update a Record
- CakePHP - Delete a Record
- CakePHP - Services
- CakePHP - Errors & Exception Handling
- CakePHP - Logging
- CakePHP - Form Handling
- CakePHP - Internationalization
- CakePHP - Session Management
- CakePHP - Cookie Management
- CakePHP - Security
- CakePHP - Validation
- CakePHP - Creating Validators
- CakePHP - Pagination
- CakePHP - Date and Time
- CakePHP - File upload
- CakePHP Useful Resources
- CakePHP - Quick Guide
- CakePHP - Useful Resources
- CakePHP - Discussion
CakePHP - Security
Security is another important feature while building web applications. It assures the users of the website that, their data is secured. CakePHP provides some tools to secure your application.
Encryption and Decryption
Security library in CakePHP provides methods, by which we can encrypt and decrypt data. Following are the two methods, which are used for the same purpose.
static Cake\Utility\Security::encrypt($text, $key, $hmacSalt = null) static Cake\Utility\Security::decrypt($cipher, $key, $hmacSalt = null)
The encrypt method will take text and key as the argument to encrypt data and the return value will be the encrypted value with HMAC checksum.
To hash a data, hash() method is used. Following is the syntax of the hash() method.
static Cake\Utility\Security::hash($string, $type = NULL, $salt = false)
CSRF
CSRF stands for Cross Site Request Forgery. By enabling the CSRF Component, you get protection against attacks. CSRF is a common vulnerability in web applications.
It allows an attacker to capture and replay a previous request, and sometimes submit data requests using image tags or resources on other domains. The CSRF can be enabled by simply adding the CsrfComponent to your components array as shown below −
public function initialize(): void { parent::initialize(); $this->loadComponent('Csrf'); }
The CsrfComponent integrates seamlessly with FormHelper. Each time you create a form with FormHelper, it will insert a hidden field containing the CSRF token.
While this is not recommended, you may want to disable the CsrfComponent on certain requests. You can do so by using the controller’s event dispatcher, during the beforeFilter() method.
public function beforeFilter(Event $event) { $this->eventManager()->off($this->Csrf); }
Security Component
Security Component applies tighter security to your application. It provides methods for various tasks like −
Restricting which HTTP methods your application accepts − You should always verify the HTTP method, being used before executing side-effects. You should check the HTTP method or use Cake\Network\Request::allowMethod() to ensure the correct HTTP method is used.
Form tampering protection − By default, the SecurityComponent prevents users from tampering with forms in specific ways. The SecurityComponent will prevent the following things −
Unknown fields cannot be added to the form.
Fields cannot be removed from the form.
Values in hidden inputs cannot be modified.
Requiring that SSL be used − All actions to require a SSL- secured
Limiting cross controller communication − We can restrict which controller can send request to this controller. We can also restrict which actions can send request to this controller’s action.
Example
Make changes in the config/routes.php file as shown in the following program.
config/routes.php
<?php use Cake\Http\Middleware\CsrfProtectionMiddleware; use Cake\Routing\Route\DashedRoute; use Cake\Routing\RouteBuilder; $routes->setRouteClass(DashedRoute::class); $routes->scope('/', function (RouteBuilder $builder) { $builder->registerMiddleware('csrf', new CsrfProtectionMiddleware([ 'httpOnly' => true, ])); $builder->applyMiddleware('csrf'); //$builder->connect('/pages', ['controller'=>'Pages','action'=>'display', 'home']); $builder->connect('login',['controller'=>'Logins','action'=>'index']); $builder->fallbacks(); });
Create a LoginsController.php file at src/Controller/LoginsController.php. Copy the following code in the controller file.
src/Controller/LoginsController.php
<?php namespace App\Controller; use App\Controller\AppController; class LoginsController extends AppController { public function initialize() : void { parent::initialize(); $this->loadComponent('Security'); } public function index(){ } } ?>
Create a directory Logins at src/Template and under that directory create a View file called index.php. Copy the following code in that file.
src/Template/Logins/index.php
<?php echo $this->Form->create(NULL,array('url'=>'/login')); echo $this->Form->control('username'); echo $this->Form->control('password'); echo $this->Form->button('Submit'); echo $this->Form->end(); ?>
Execute the above example by visiting the following URL − http://localhost/cakephp4/login
Output
Upon execution, you will receive the following output.
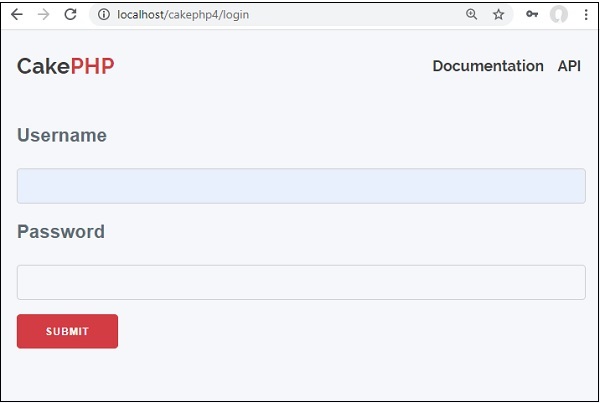