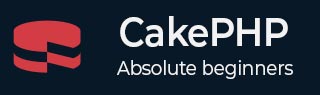
- CakePHP Tutorial
- CakePHP - Home
- CakePHP - Overview
- CakePHP - Installation
- CakePHP - Folder Structure
- CakePHP - Project Configuration
- CakePHP - Routing
- CakePHP - Controllers
- CakePHP - Views
- CakePHP - Extending Views
- CakePHP - View Elements
- CakePHP - View Events
- CakePHP - Working with Database
- CakePHP - View a Record
- CakePHP - Update a Record
- CakePHP - Delete a Record
- CakePHP - Services
- CakePHP - Errors & Exception Handling
- CakePHP - Logging
- CakePHP - Form Handling
- CakePHP - Internationalization
- CakePHP - Session Management
- CakePHP - Cookie Management
- CakePHP - Security
- CakePHP - Validation
- CakePHP - Creating Validators
- CakePHP - Pagination
- CakePHP - Date and Time
- CakePHP - File upload
- CakePHP Useful Resources
- CakePHP - Quick Guide
- CakePHP - Useful Resources
- CakePHP - Discussion
CakePHP - Routing
In this chapter, we are going to learn the following topics related to routing −
- Introduction to Routing
- Connecting Routes
- Passing Arguments to Routes
- Generating urls
- Redirect urls
Introduction to Routing
In this section, we will see how you can implement routes, how you can pass arguments from URL to controller’s action, how you can generate URLs, and how you can redirect to a specific URL. Normally, routes are implemented in file config/routes.php. Routing can be implemented in two ways −
- static method
- scoped route builder
Here, is an example presenting both the types.
// Using the scoped route builder. Router::scope('/', function ($routes) { $routes->connect('/', ['controller' => 'Articles', 'action' => 'index']); }); // Using the static method. Router::connect('/', ['controller' => 'Articles', 'action' => 'index']);
Both the methods will execute the index method of ArticlesController. Out of the two methods, scoped route builder gives better performance.
Connecting Routes
Router::connect() method is used to connect routes. The following is the syntax of the method −
static Cake\Routing\Router::connect($route, $defaults =[], $options =[])
There are three arguments to the Router::connect() method −
The first argument is for the URL template you wish to match.
The second argument contains default values for your route elements.
The third argument contains options for the route, which generally contains regular expression rules.
Here, is the basic format of a route −
$routes->connect( 'URL template', ['default' => 'defaultValue'], ['option' => 'matchingRegex'] );
Example
Make changes in the config/routes.php file as shown below.
config/routes.php
<?php use Cake\Http\Middleware\CsrfProtectionMiddleware; use Cake\Routing\Route\DashedRoute; use Cake\Routing\RouteBuilder; $routes->setRouteClass(DashedRoute::class); $routes->scope('/', function (RouteBuilder $builder) { // Register scoped middleware for in scopes. $builder->registerMiddleware('csrf', new CsrfProtectionMiddleware([ 'httpOnly' => true, ])); $builder->applyMiddleware('csrf'); $builder->connect('/', ['controller' => 'Tests', 'action' => 'show']); $builder->connect('/pages/*', ['controller' => 'Pages', 'action' => 'display']); $builder->fallbacks(); });
Create a TestsController.php file at src/Controller/TestsController.php. Copy the following code in the controller file.
src/Controller/TestsController.php
<?php declare(strict_types=1); namespace App\Controller; use Cake\Core\Configure; use Cake\Http\Exception\ForbiddenException; use Cake\Http\Exception\NotFoundException; use Cake\Http\Response; use Cake\View\Exception\MissingTemplateException; class TestsController extends AppController { public function show() { } }
Create a folder Tests under src/Template and under that folder, create a View file called show.php. Copy the following code in that file.
src/Template/Tests/show.php
<h1>This is CakePHP tutorial and this is an example of connecting routes.</h1>
Execute the above example by visiting the following URL which is available at http://localhost/cakephp4/
Output
The above URL will yield the following output.
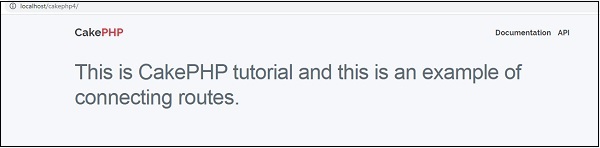
Passed Arguments
Passed arguments are the arguments which are passed in the URL. These arguments can be passed to controller’s action. These passed arguments are given to your controller in three ways.
As arguments to the action method
Following example shows, how we can pass arguments to the action of the controller. Visit the following URL at http://localhost/cakephp4/tests/value1/value2
This will match the following route line.
$builder->connect('tests/:arg1/:arg2', ['controller' => 'Tests', 'action' => 'show'],['pass' => ['arg1', 'arg2']]);
Here, the value1 from URL will be assigned to arg1 and value2 will be assigned to arg2.
As numericallyindexed array
Once the argument is passed to the controller’s action, you can get the argument with the following statement.
$args = $this->request->params[‘pass’]
The arguments passed to controller’s action will be stored in $args variable.
Using routing array
The argument can also be passed to action by the following statement −
$routes->connect('/', ['controller' => 'Tests', 'action' => 'show',5,6]);
The above statement will pass two arguments 5, and 6 to TestController’s show() method.
Example
Make Changes in the config/routes.php file as shown in the following program.
config/routes.php
<?php use Cake\Http\Middleware\CsrfProtectionMiddleware; use Cake\Routing\Route\DashedRoute; use Cake\Routing\RouteBuilder; $routes->setRouteClass(DashedRoute::class); $routes->scope('/', function (RouteBuilder $builder) { // Register scoped middleware for in scopes. $builder->registerMiddleware('csrf', new CsrfProtectionMiddleware([ 'httpOnly' => true, ])); $builder->applyMiddleware('csrf'); $builder->connect('tests/:arg1/:arg2', ['controller' => 'Tests', 'action' => 'show'],['pass' => ['arg1', 'arg2']]); $builder->connect('/pages/*', ['controller' => 'Pages', 'action' => 'display']); $builder->fallbacks(); });
Create a TestsController.php file at src/Controller/TestsController.php. Copy the following code in the controller file.
src/Controller/TestsController.php
<?php declare(strict_types=1); namespace App\Controller; use Cake\Core\Configure; use Cake\Http\Exception\ForbiddenException; use Cake\Http\Exception\NotFoundException; use Cake\Http\Response; use Cake\View\Exception\MissingTemplateException; class TestsController extends AppController { public function show($arg1, $arg2) { $this->set('argument1',$arg1); $this->set('argument2',$arg2); } }
Create a folder Tests at src/Template and under that folder create a View file called show.php. Copy the following code in that file.
src/Template/Tests/show.php.
<h1>This is CakePHP tutorial and this is an example of Passed arguments.</h1> <?php echo "Argument-1:".$argument1."<br/>"; echo "Argument-2:".$argument2."<br/>"; ?>
Execute the above example by visiting the following URL http://localhost/cakephp4/tests/Virat/Kunal
Output
Upon execution, the above URL will produce the following output.
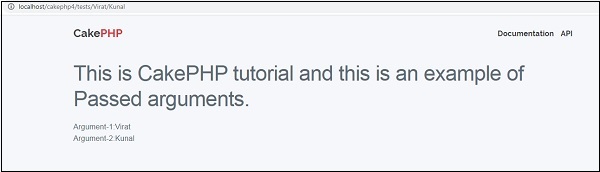
Generating URLs
This is a cool feature of CakePHP. Using the generated URLs, we can easily change the structure of URL in the application without modifying the whole code.
url( string|array|null $url null , boolean $full false )
The above function will take two arguments −
The first argument is an array specifying any of the following - 'controller', 'action', 'plugin'. Additionally, you can provide routed elements or query string parameters. If string, it can be given the name of any valid url string.
If true, the full base URL will be prepended to the result. Default is false.
Example
Make Changes in the config/routes.php file as shown in the following program.
config/routes.php
<?php use Cake\Http\Middleware\CsrfProtectionMiddleware; use Cake\Routing\Route\DashedRoute; use Cake\Routing\RouteBuilder; $routes->setRouteClass(DashedRoute::class); $routes->scope('/', function (RouteBuilder $builder) { // Register scoped middleware for in scopes. $builder->registerMiddleware('csrf', new CsrfProtectionMiddleware([ 'httpOnly' => true, ])); $builder->applyMiddleware('csrf'); $builder->connect('/generate',['controller'=>'Generates','action'=>'show']); $builder->fallbacks(); });
Create a GeneratesController.php file at src/Controller/GeneratesController.php. Copy the following code in the controller file.
src/Controller/GeneratesController.php
<?php declare(strict_types=1); namespace App\Controller; 21 use Cake\Core\Configure; use Cake\Http\Exception\ForbiddenException; use Cake\Http\Exception\NotFoundException; use Cake\Http\Response; use Cake\View\Exception\MissingTemplateException; class GeneratesController extends AppController { public function show() { } }
Create a folder Generates at src/Template and under that folder, create a View file called show.php. Copy the following code in that file.
src/Template/Generates/show.php
<h1>This is CakePHP tutorial and this is an example of Generating URLs<h1>
Execute the above example by visiting the following URL −
http://localhost/cakephp4/generate
Output
The above URL will produce the following output −
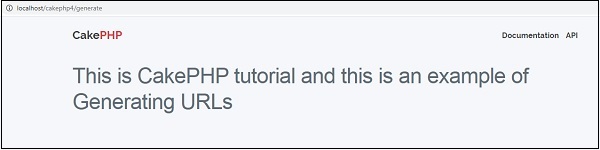
Redirect Routing
Redirect routing is useful, when we want to inform client applications that, this URL has been moved. The URL can be redirected using the following function −
static Cake\Routing\Router::redirect($route, $url, $options =[])
There are three arguments to the above function as follows −
A string describing the template of the route.
A URL to redirect to.
An array matching the named elements in the route to regular expressions which that element should match.
Example
Make Changes in the config/routes.php file as shown below. Here, we have used controllers that were created previously.
config/routes.php
<?php use Cake\Http\Middleware\CsrfProtectionMiddleware; use Cake\Routing\Route\DashedRoute; use Cake\Routing\RouteBuilder; $routes->setRouteClass(DashedRoute::class); $routes->scope('/', function (RouteBuilder $builder) { // Register scoped middleware for in scopes. $builder->registerMiddleware('csrf', new CsrfProtectionMiddleware([ 'httpOnly' => true, ])); $builder->applyMiddleware('csrf'); $builder->connect('/generate',['controller'=>'Generates','action'=>'show']); $builder->redirect('/redirect','https://tutorialspoint.com/'); $builder->fallbacks(); });
Execute the above example by visiting the following URLs.
URL 1 − http://localhost/cakephp4/generate
Output for URL 1
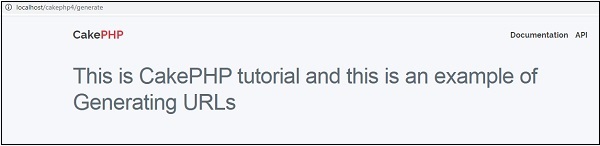
URL 2 − http://localhost/cakephp4/redirect
Output for URL 2
You will be redirected to https://tutorialspoint.com