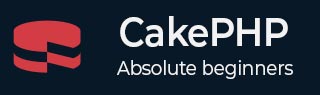
- CakePHP Tutorial
- CakePHP - Home
- CakePHP - Overview
- CakePHP - Installation
- CakePHP - Folder Structure
- CakePHP - Project Configuration
- CakePHP - Routing
- CakePHP - Controllers
- CakePHP - Views
- CakePHP - Extending Views
- CakePHP - View Elements
- CakePHP - View Events
- CakePHP - Working with Database
- CakePHP - View a Record
- CakePHP - Update a Record
- CakePHP - Delete a Record
- CakePHP - Services
- CakePHP - Errors & Exception Handling
- CakePHP - Logging
- CakePHP - Form Handling
- CakePHP - Internationalization
- CakePHP - Session Management
- CakePHP - Cookie Management
- CakePHP - Security
- CakePHP - Validation
- CakePHP - Creating Validators
- CakePHP - Pagination
- CakePHP - Date and Time
- CakePHP - File upload
- CakePHP Useful Resources
- CakePHP - Quick Guide
- CakePHP - Useful Resources
- CakePHP - Discussion
CakePHP - Pagination
If we want to show a set of data that is huge, we can use pagination and this feature is available with cake php 4 which is very easy to use.
We have a table titled “articles” with following data −
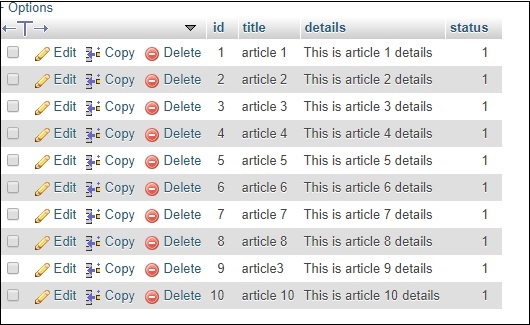
Let us use pagination to display the data in the form of pages, instead of showing them all together.
Example
Make Changes in the config/routes.php file as shown in the following program.
config/routes.php
<?php use Cake\Http\Middleware\CsrfProtectionMiddleware; use Cake\Routing\Route\DashedRoute; use Cake\Routing\RouteBuilder; $routes->setRouteClass(DashedRoute::class); $routes->scope('/', function (RouteBuilder $builder) { $builder->registerMiddleware('csrf', new CsrfProtectionMiddleware([ 'httpOnly' => true, ])); $builder->applyMiddleware('csrf'); //$builder->connect('/pages',['controller'=>'Pages','action'=>'display', 'home']); $builder->connect('posts',['controller'=>'Posts','action'=>'index']); $builder->fallbacks(); });
Create a PostsController.php file at src/Controller/PostsController.php. Copy the following code in the controller file. Ignore, if already created.
src/Controller/PostsController.php
<?php namespace App\Controller; use App\Controller\AppController; class PostsController extends AppController { public function index(){ $this->loadModel('articles'); $articles = $this->articles->find('all')->order(['articles.id ASC']); $this->set('articles', $this->paginate($articles, ['limit'=> '3'])); } } ?>
The data from articles table is fetched using −
$this->loadModel('articles'); $articles = $this->articles->find('all')->order(['articles.id ASC']);
To apply pagination and we would show the data with 3 per records and the same is done as follows −
$this->set('articles', $this->paginate($articles, ['limit'=> '3']));
This is enough to activate pagination on the articles tables.
Create a directory Posts at src/Template and under that directory create a Viewfile called index.php. Copy the following code in that file.
src/Template/Posts/index.php
<div> <?php foreach ($articles as $key=>$article) {?> <a href="#"> <div> <p><?= $article->title ?> </p> <p><?= $article->details ?></p> </div> </a> <br/> <?php } ?> <ul class="pagination"> <?= $this->Paginator->prev("<<") ?> <?= $this->Paginator->numbers() ?> <?= $this->Paginator->next(">>") ?> </ul> </div>
The pagination for the list of pages is done as follows −
<ul class="pagination"> <?= $this->Paginator->prev("<<") ?> <?= $this->Paginator->numbers() ?> <?= $this->Paginator->next(">>") ?> </ul>
Execute the above example by visiting the following URL −
http://localhost/cakephp4/posts
Output
When you run the code, you will see the following output −
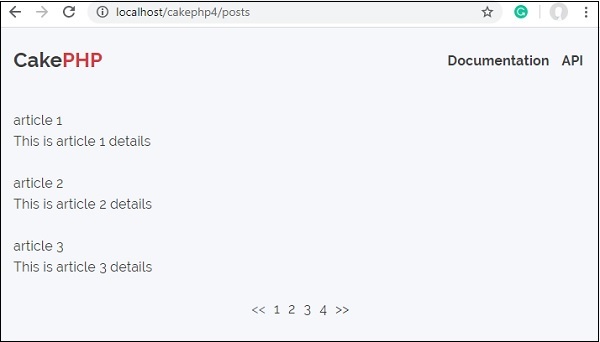
Click on the numbers below, to switch to next page, or use the next or previous button.
For example
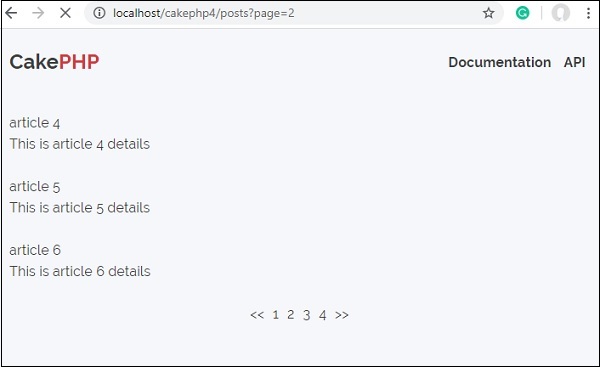
You will see that page=2 is appended to the page url in the browser.