
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 128 Articles for TypeScript

5K+ Views
The Symbol is introduced in the last main revision of JavaScript, ES6. The Symbol is a data type. As we use the number, string, or Boolean to create the variables of different data-type, we can use the symbol type to create the Symbol. Using the symbol types has many benefits as it provides more features than other data types. In this tutorial, we will learn about the basics of the Symbol and its different uses of the Symbol. Syntax Users can follow the syntax below to create a variable of symbol data type. let test_symbol = Symbol(); let key_symbol = ... Read More
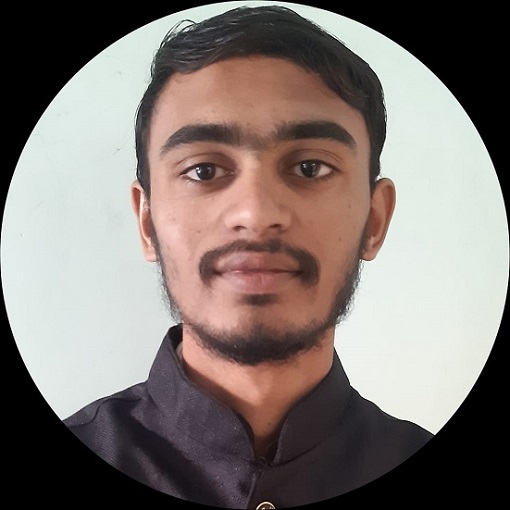
3K+ Views
In this TypeScript tutorial, we will learn to convert the string to uppercase. We need to convert every single alphabetic character to uppercase to convert the whole string uppercase by keeping the numbers and special characters as it is. As a beginner TypeScript programmer, maybe a question can arise in your mind what is the need to convert the string to uppercase? Here is the very straightforward answer. Have you ever used the search bar of any website, which shows different results when you search? If yes, search the uppercase and normal strings, which will return the search result. It ... Read More
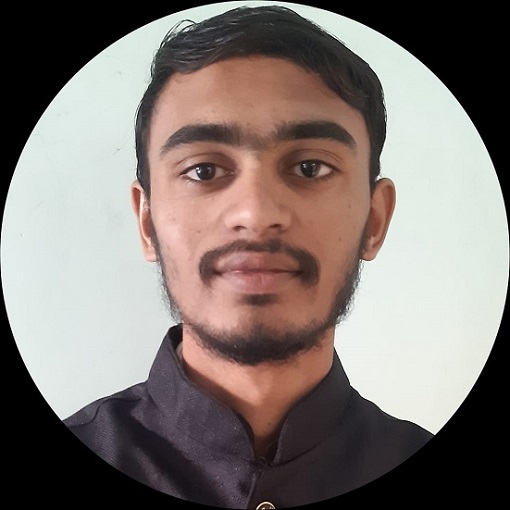
16K+ Views
Have you ever tried to delete the object properties in TypeScript? Then you definitely came across the ‘delete’ operator. In TypeScript, the delete operator only allows deleting the undefined, optional, or any object properties. Also, it returns the boolean values according to whether it deletes the property or not. Below, we will understand the different use cases of the delete operator in TypeScript via different examples. Using the Delete Operator to Delete the Object Properties The main use of the delete operator is to delete the optional properties of an object. We can use the delete keyword as an operator ... Read More
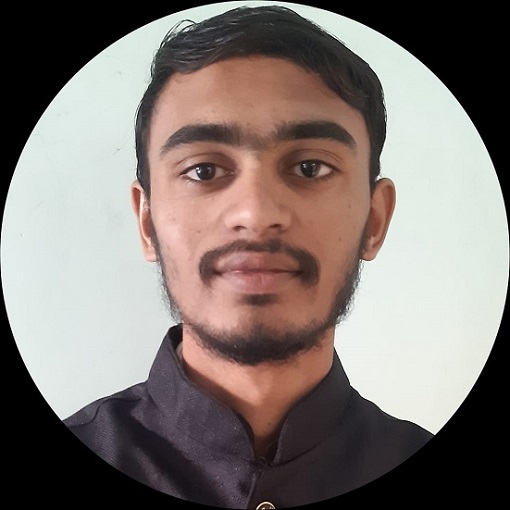
25K+ Views
In this tutorial, we will learn to round numbers in TypeScript. In TypeScript, the number data types can contain number values with decimal parts, and sometimes, we require to remove decimal part by rounding the number value. For example, if you want to show the progress of something in the application, and you are getting the decimal value of the progress, but you want to show the integer values only, then it is required to round the numbers. Here, we will learn 3 to 4 different methods to round the numbers. Using The Math.round() Method to Round a Number In ... Read More
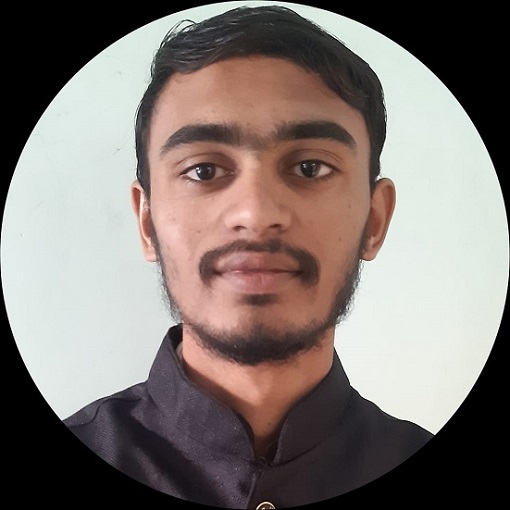
5K+ Views
The “this” keyword is one of the most used keywords in TypeScript. For beginner TypeScript programmers, it’s complex to understand how this keyword works, but they will learn by the end of this tutorial. In this tutorial, we will learn to use this keyword in TypeScript. Syntax Users can follow the syntax below to use this keyword generally. Users can observe how we can access the variable or invoke the method using this keyword. this.var_name; this.method_name(); console.log(this.var_name); In TypeScript, this keyword refers to the global object if it’s not used inside any class or method. Even if we use ... Read More
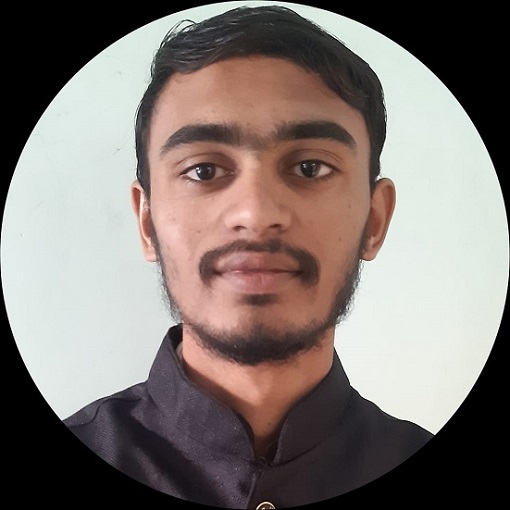
11K+ Views
The URI stands for the uniform resource identifier. The URL is one of the most common URIS. We use the URL (uniform resource locator) to find the web page located on the internet. The web pages also contain resources. In simple terms, URI is a string containing some characters, and we can identify the physical and logical resources on the web using the URI. The URL is a subset of the URI, which stores the document address on the web. Reasons to Encode URI The first question that arises in your mind after reading this tutorial's title is why we ... Read More
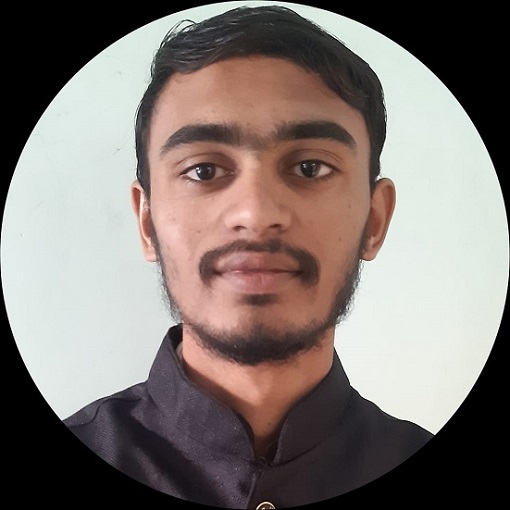
14K+ Views
The object contains the key-value pairs of its properties, or it can be an instance of the class in TypeScript. The class and its objects are the base of object-oriented programming. So, without an object, OOPS doesn’t exist. Mainly, objects are used to invoke the non-static methods of the class. There are multiple ways to define the objects in TypeScript. We will learn all ways to define objects one by one below. Use The Object Literal Notation to Create Objects The object-literal notation means we can create the object using the two curly braces. By comma separation, we need to ... Read More
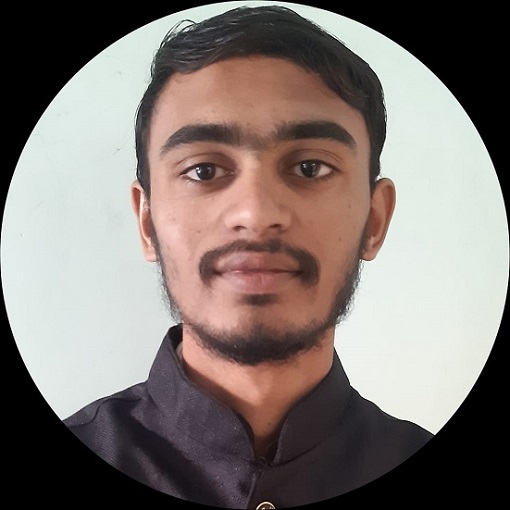
4K+ Views
In TypeScript, we can declare the function using the function keyword. It is a block of code that performs some operation, and we can reuse the function code by invoking the function. There are two types of functions. One is a named function, and another is an anonymous function. The name function means we can identify it using the unique identifier, and the identifier doesn’t exist for the anonymous functions. We will talk about anonymous functions in depth in this tutorial. As the word ‘anonymous function’ states, it means a function defined without any name or identifier. We can simply ... Read More
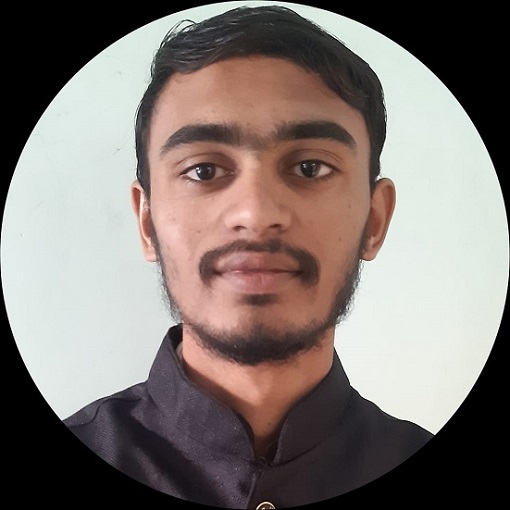
976 Views
Introduction to Abstraction We wanted readers to get familiar with abstract classes and the requirements of abstract classes before implementing them. The abstraction means hiding. It is used to hide the lower-level code implementation from users and some developers. Furthermore, it is used to show only the required information about the method rather than showing the whole complex implementation of methods. Create Abstract Classes We can use the abstract keyword to define the abstract classes or methods. The abstract classes can contain the normal and abstract types of methods. In the abstract class, we need to implement the functional or ... Read More
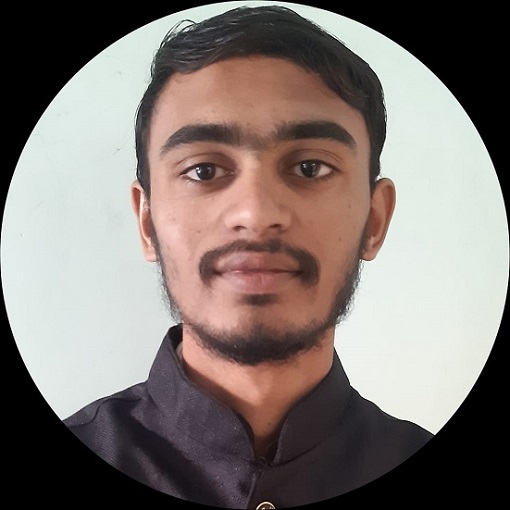
5K+ Views
In TypeScript, an object contains the properties and their values. We can use the for-in loop statement to iterate through every property of the objects and get its value. This tutorial will teach us to iterate through the object key-value pairs via different examples. Also, we will learn what kind of error we can get while iterating through the object properties and how to fix it quickly. Syntax Users can follow the syntax below to iterate through the iterable object properties using the for-in loop statement. For (var_name in object){ Statements or block to execute; } ... Read More
To Continue Learning Please Login