
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 128 Articles for TypeScript
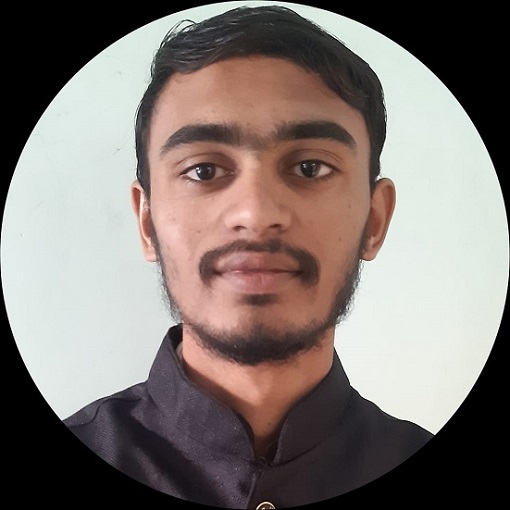
6K+ Views
In TypeScript, parameter destructuring is unpacking the argument object into separate parameter variables. For example, suppose we have passed the object with multiple properties as an argument of any function. In that case, we can destructure the object in the parameter and access all required properties of the object in a separate variable. However, we can destructure the object properties or array passed as an argument of the function. Also, we have to define the type of every parameter using type annotation in TypeScript while destructuring the parameters. So it might not be very clear for beginners. In this tutorial, ... Read More
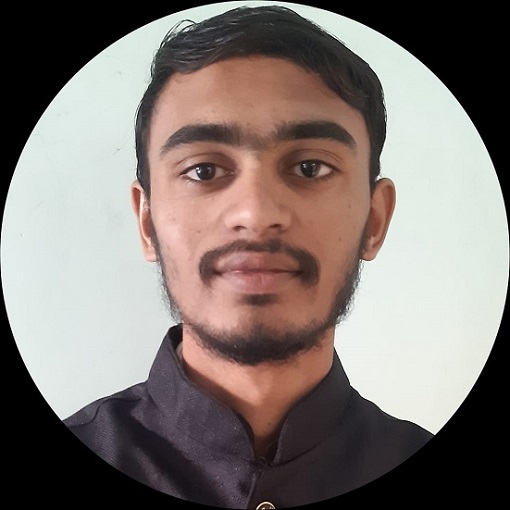
3K+ Views
Recursion is a fundamental programming concept that refers to a function calling itself. It can be a powerful tool for solving problems, but it can also be a source of confusion and frustration, especially for beginners. In this tutorial, we'll explore how to use recursion effectively in TypeScript, a popular superset of JavaScript that adds optional static typing and other features. One important thing to keep in mind when working with recursion is to define a base case, which is a condition that stops the function from calling itself again. Without a base case, the function will keep calling itself ... Read More
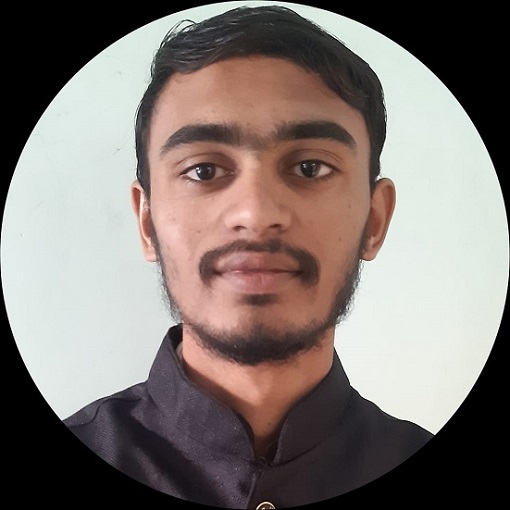
10K+ Views
An object with one or more elements is known as an array. Each of these components can be an object or a simple data type. For example, you can put dates, strings, and numbers in the same array. Information can also be stored using associative arrays. An array that employs strings as indexes is known as an associative array. You can create a mixed array that uses numeric and string indexes within a single array. The length of an array will only reflect the number of entries with numeric indexes if it has both numeric and string indexes. In terms ... Read More
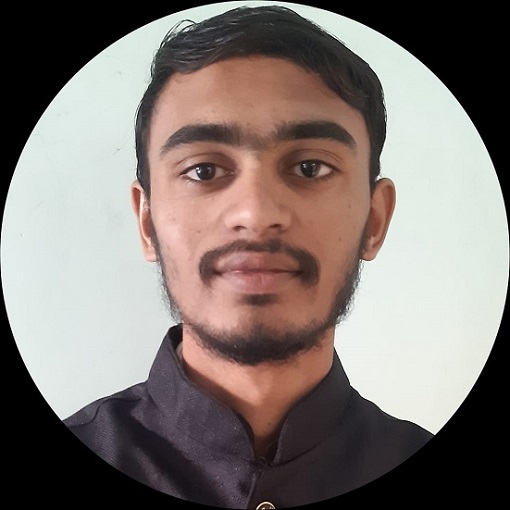
7K+ Views
A two-dimensional array in TypeScript is an array of arrays, or a matrix, which can be used to represent a table of data, a chess board, or any other type of grid. Two-dimensional arrays are useful when working with a data grid, such as a table or a chessboard. They allow you to store and manipulate data in a structured way and access elements using a pair of indices. Create a two-dimensional array To create a two-dimensional array in TypeScript, users can use an array literal with the desired dimensions, like this − Syntax Users can follow the syntax below ... Read More
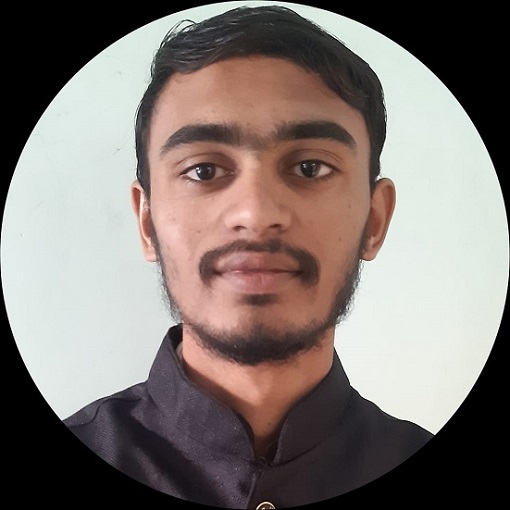
887 Views
The function or method overloading allows developers to create multiple functions with the same name. Every function contains the same number of parameters but different data types. Also, the return type of the overloaded function can vary. Function overloading is the concept of object-oriented programming. Also, TypeScript supports OOPS, so we can easily implement function overloading in TypeScript. Furthermore, function overloading provides code reusability and helps developers to increase code readability. Let’s understand the use of function overloading via real-life examples. For example, you have created a function that takes a string as a parameter and returns the length of ... Read More
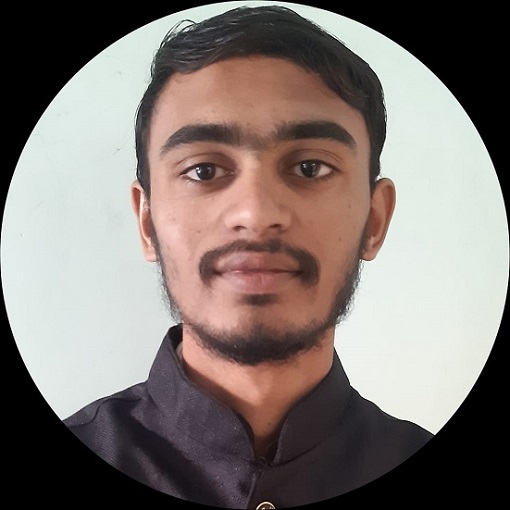
1K+ Views
TypeScript is a strongly typed, object-oriented programming language that enables developers to write code that is cleaner and easier to understand. The dynamic date population theory in TypeScript is that a JavaScript application can automatically populate a calendar, list, or other types of display with the current Date, time, or other dynamic information. This allows developers to create user interfaces that automatically display the current Date, time, or other dynamic information without manually entering the data. This can be especially useful for applications requiring frequent updates or user interaction. To dynamically populate a date in TypeScript, developers can use the ... Read More
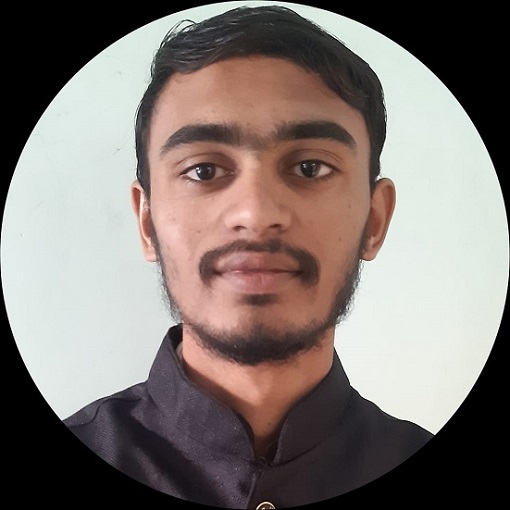
1K+ Views
The idea of boxing and unboxing is crucial to TypeScript. A value type in TypeScript is automatically converted into a reference type using a process known as boxing. In other words, boxing refers to transforming a value type into a reference type, and unboxing refers to transforming a reference type into a value type. These are two techniques used in TypeScript to convert a value type to an object type. Boxing is the process of wrapping a value type in an object type. In contrast, unboxing is the process of unwrapping an object type back to a value type. The ... Read More

2K+ Views
TypeScript is a type-strict language in which we need to define the type for every variable. Also, we need to define the return type of the function and the types of parameters of the function. The never is also one of type in TypeScript like other data types such as string, number, boolean, symbol, etc. We can use the ‘never’ keyword to make a variable of never type. Users can use the never type when they are sure about any situation that will never occur. For example, we can use the never as a return type when we are sure ... Read More

213 Views
In any programming language, we use the loops to execute the same code block repeatedly or multiple times. The loops allow us to write less code to execute the same code block many times. The for loop is one of the loops in TypeScript. As given below, there are also three different sub-types of the for loop in TypeScript. Normal for loop for-of loop for-in loop We will learn about all variants of the for loop in this TypeScript tutorial. Also, we will learn how each type of for loop is different from others. Introduction to normal for ... Read More

208 Views
We will learn about the tuple types in TypeScript. In JavaScript, an array can contain elements of different data types. Still, as TypeScript is a superset of JavaScript and type-strict language, a TypeScript array can contain only single types of elements. So, the tuple allows us to store elements of the different data types in the TypeScript array. Also, when we store elements inside the tuple, the order of elements is important; Otherwise, the TypeScript compiler can generate an error while compiling the code. Syntax You can follow the syntax below to define the tuples in TypeScript. let sample_tuple: [string, ... Read More