
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 128 Articles for TypeScript
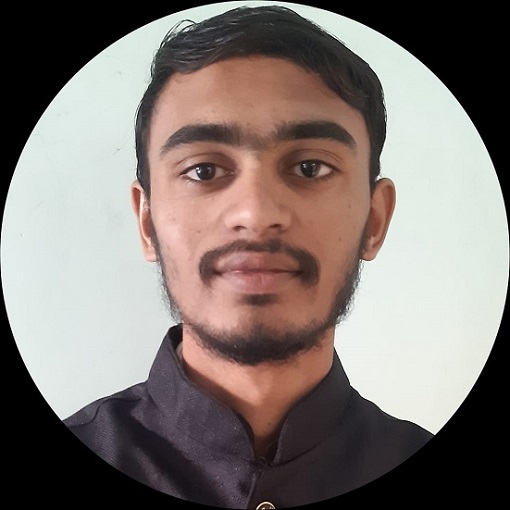
713 Views
What is Duck Typing? First, we will know what Duck Typing is. According to programmers, the circumstance where an object's type is decided by its behaviour, like methods and attributes, rather than its class is known as "duck typing". Duck Typing in TypeScript The usage of interfaces in TypeScript makes duck typing possible. Where interface means the set of methods and characteristics an object must have to fall under that type are described. For example, if an interface defines the function, any object with the method called "myFunc()" may be treated as belonging to a specific kind, regardless of ... Read More
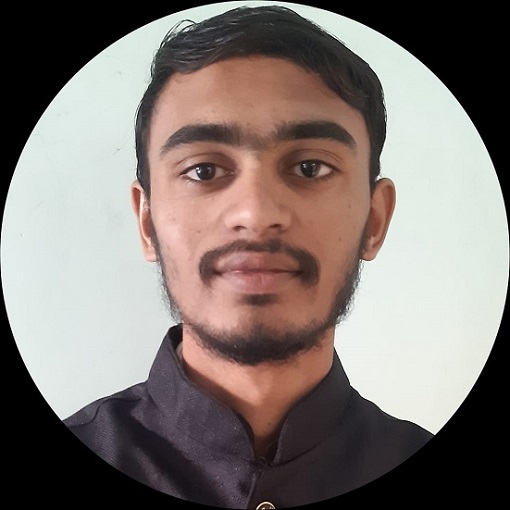
4K+ Views
In React with TypeScript, you can use conditional rendering to choose what to render based on a condition. This is useful when displaying different content or components based on specific criteria. There are a few different ways to implement conditional rendering in React with TypeScript. One way is to use the conditional operator (also known as the ternary operator). This operator takes a condition and returns one value if the condition is true and another if the condition is false. You can also use the && operator to render a component based on a condition conditionally. This operator evaluates to ... Read More
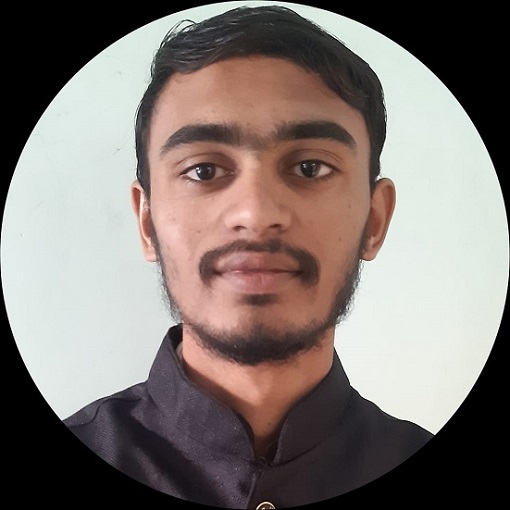
10K+ Views
In TypeScript, to access an element, or we can say HTML components, we use the Document Object Model (DOM). The DOM defines an HTML and XML programming interface that visualizes a document's structure as a tree of nodes. A paragraph, a button, a div, a heading, etc., are just a few examples of the document elements each node in the tree represents. The document object in TypeScript serves as the doorway to the DOM. This means we can easily access the elements of the DOM using TypeScript. There are several ways to access elements, those are − Using the ... Read More

276 Views
In this article, you will understand how optional chaining works in TypeScript. Optional chaining operator (?.) accesses an object’s property. If the objects property is null or not defined, it returns ‘undefined’. Let us first understand what TypeScript is. Strongly typed programming language TypeScript, which is based on JavaScript, gives you better tools at any scale. Code written in TypeScript can be converted to execute in any JavaScript-compatible environment. JavaScript is understood by TypeScript, and type inference is used to provide excellent tooling without the need for additional code. Example 1 In this example, we use the optional chaining operator ... Read More
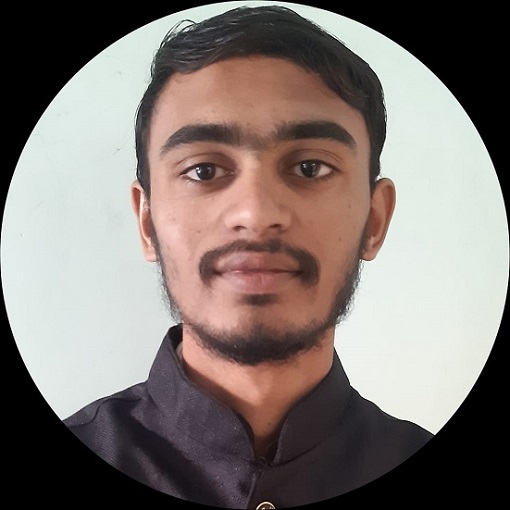
367 Views
TypeScript allows us to create a new type from the existing types, and we can use the utility types for such transformation. There are various utility types that exist in TypeScript, and we can use any utility type according to our requirements of the type transformation. In this tutorial, we will learn about the different utility types with examples. Partial Type in TypeScript The Partial utility type transforms all the properties of the current type to optional. The meaning of the partial is either all, some, or none. So, it makes all properties optional, and users can use it while ... Read More
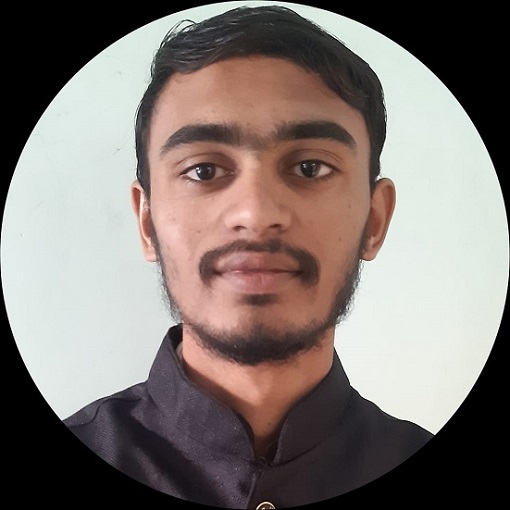
1K+ Views
In TypeScript, a tuple is an object which contains the values of different data types. The length of a tuple is always pre-defined. It is similar to an array, but the array contains the values of only one data type, and the tuple contains values of multiple data types. Destructuring the tuple means getting the values from the tuple in separate variables. For example, we need to use tuple values multiple times in the code block. We can get all values in separate variables and use variables whenever we require tuple values, rather than every time accessing values from tuple ... Read More
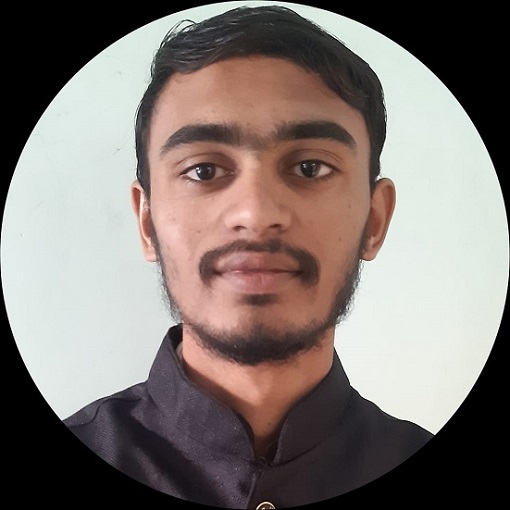
269 Views
We will learn to use the readonly keyword in TypeScript. The readonly keyword allows developers to make class properties and members read-only, and we can’t edit the value of the read-only properties. It works the same as the const keyword, but the const keyword is used for the variables, and the readonly keyword is used with the class member properties. Also, we can’t assign the values to the const variables after initializing them. Still, we can assign the values to the read-only properties inside the class constructor, and we can’t modify them after assigning them once. Syntax Users can follow ... Read More
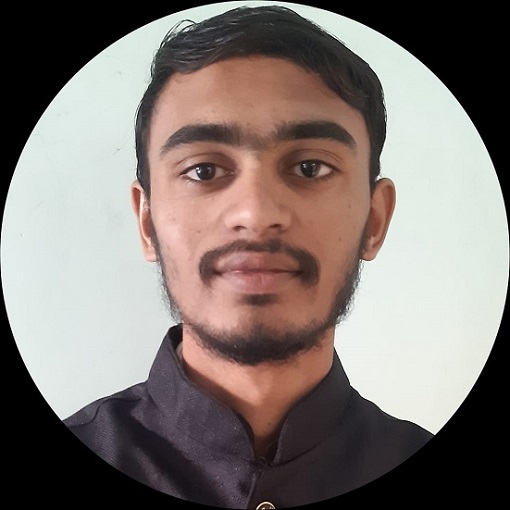
2K+ Views
The simple definition of the immutable object property is the properties we can’t modify once we define and initialize the object property. We can use the const keyword, but we have to initialize the property while creating the property. So, we have to use the readonly keyword to make the property immutable, allowing it to be read-only. So, once we initialize the property, we can’t modify the value of the property. Syntax Users can follow the syntax below to use the readonly keyword to make object properties immutable. interface test { readonly property1: boolean; } var object: ... Read More
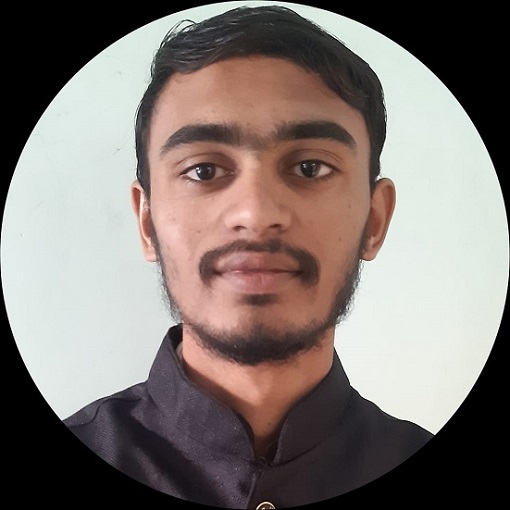
2K+ Views
The stack is a data structure based on the LIFO, which means last in, first out. In brief, it says that whatever element you add at last in the stack comes out first from the stack. There are some basic operations that users can perform on the stack. For example, we can push an element to the stack, pop an element from the stack, or peek an element from the stack. Here, users can see the basic methods of the stack, which we will also implement while creating the stack in this tutorial. Stack Methods Push() − It allows ... Read More
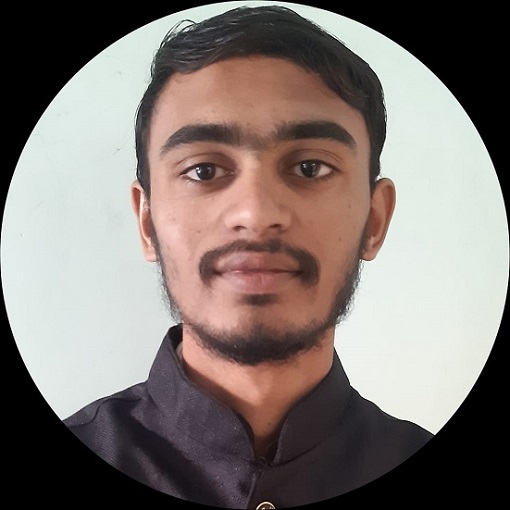
1K+ Views
We will learn to create a queue from scratch using the array in TypeScript in this tutorial. The Queue is a data structure allowing users to add elements from the end and remove them from the start. It means it works based on the FIFO concept, meaning first in, first out. Also, we can’t remove the element randomly from the queue like an array. We can only remove elements from the first index and add them to the last empty index. Here, we will use some concepts of object-oriented programming language to create a queue using an array. Methods of ... Read More