
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 766 Articles for JQuery

1K+ Views
We can access the HTML element using various methods in vanilla JavaScript or jQuery, a featured library of JavaScript. Sometimes, after accessing the DOM elements, developers may require to check if both accessed elements are the same or not. In this tutorial, we will learn to use the strict equality operator of JavaScript and a method of jQuery to check the equality of two HTML elements. Using the equality operator (==) in JavaScript We can access the HTML element by getElemenetById, querySelector, getElementByClassName, etc. methods. After that, we can store it in the JavaScript variable, and we can compare those ... Read More

3K+ Views
In JavaScript, an array is an object with an index as a key and array values as a value of a particular key of an array object. Sometimes, we require to check if two arrays are the same or not. The first solution that comes to mind is using the equality operator and comparing them like array1 == array2. Oops! It will not work as the array is an object, and we can’t compare two objects directly in JavaScript. So, we have to compare every element of the array. In this tutorial, we will learn to compare two JavaScript array ... Read More

5K+ Views
We will learn to check if users have clicked anywhere on the page except one element using JavaScript and jQuery. While working with the modals, we require to close the modal when the user clicks the outside of the modal. So, we can achieve that using various methods of JavaScript or jQuery. Also, there are other use cases where we need to check whether users have clicked on a particular element. For example, when users click outside the dropdown menu, close the dropdown menu; Otherwise, select the option from the dropdown menu. Here, we have different approaches to check if ... Read More
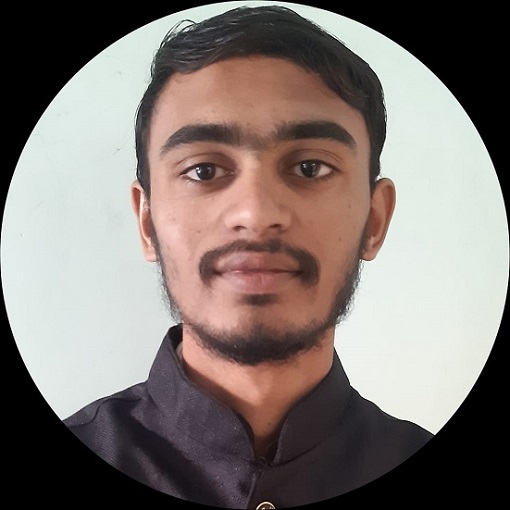
13K+ Views
In this tutorial, we will learn How to send FormData objects with Ajax requests in jQuery. The FormData object stores value in the form of key-value pair. It mainly sends form data to the server with HTTP requests. If the form’s encoding type had been set to multipart/form-data, the submitted data would have been sent similarly via the submit() function. The FormData object contains multiple operations methods such as append, delete, set, etc. Syntax const formData = new FormData() formData.append('key', 'value') Users can follow the above syntax to create a FormData object. Asynchronous JavaScript And XML are referred to ... Read More
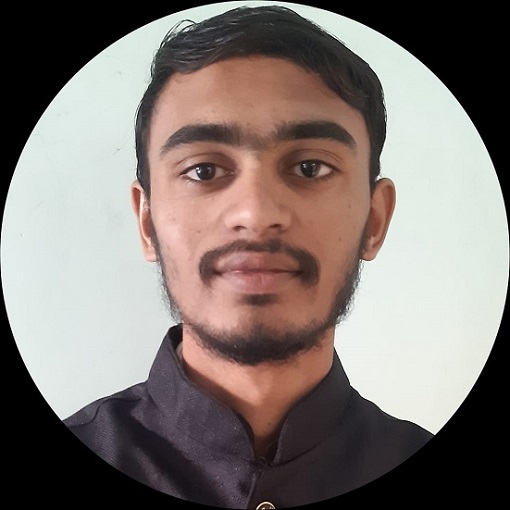
2K+ Views
In this tutorial, we will learn to fix broken images automatically in jQuery. Have you ever seen broken images while wondering on any website which shows only alternative text with the default icon of the broken image? Don’t you think it is a bad user experience? Obviously, it is. So, when you develop the application or website, you should write some JavaScript or jQuery code such that it can automatically replace broken images. Here, we will learn 2 to 3 different methods to fix broken images automatically using jQuery. Use the ‘onerror’ event inside the HTML ‘ ’ tag ... Read More

435 Views
jQuery is a feature-rich JavaScript library. We can perform a lot of actions with the help of jQuery which would otherwise require writing large pieces of code. It makes DOM manipulation, event handling, animation, ajax, etc. very easy.In this tutorial, we will learn how to register a handler to be called when the first Ajax request begins using jQuery. Ajax requests are typically HTTP requests that are called by the browser for different tasks such as GET, POST, etc. So when the tasks are performed, we can register a handler using the ajaxStart() function of jQuery. This function is always ... Read More

315 Views
In this tutorial, we will learn how to register a handler to be called when all Ajax requests are completed using jQuery. Ajax requests are typically HTTP requests that are called by the browser for different tasks such as GET, POST, etc. So when the tasks are performed, we can register a handler using the ,ajaxStop() function of jQuery.SyntaxUse the following syntax to register a handler after an ajax request −$(document).ajaxStop(function () { console.log('Registered handler.') })Explanation − Suppose we have a GET request on an API. When the API returns a result, jQuery checks if any requests are pending ... Read More

850 Views
In this tutorial, we will learn how to register a handler to be called when Ajax requests complete using jQuery. Ajax requests are typically HTTP requests that are called by the browser for different tasks such as GET, POST, etc. So when the tasks are performed, we can register a handler using the ajaxComplete() function of jQuery. This function is always triggered when the request is completed.SyntaxUse the following syntax to register a handler after every ajax request −$(document).ajaxComplete(function () { console.log('Registered handler.') })Explanation − Suppose we have a GET request on an API. When the API returns a ... Read More

115 Views
In this tutorial, we will learn how to check the lock-state of a callback list using jQuery. The lock is a callback list in jQuery in the current state. We can toggle the lock state so that additional changes cannot be made unless required.SyntaxThe callbacks list is locked and checked as follows −// Get callbacks list at current state var callbacks = $.Callbacks() // Lock the callbacks list callbacks.lock() // Check callbacks is locked or not console.log(callbacks.locked())AlgorithmFirst we receive the callbacks list at the current state using the Callbacks() function.Then we lock it using the lock() function and ... Read More

1K+ Views
In this tutorial, we will learn How to attach a function to be executed before an Ajax request is sent using jQuery. Ajax requests are typically HTTP requests that are called by the browser for different tasks such as GET, POST, etc. So when the tasks are performed, we can register a handler using the ajaxSend() function of jQuery. This event is called by jQuery whenever an ajax request is to be made.SyntaxUse the following syntax to register a handler before an ajax request −$(document).ajaxSend(function () { console.log('Triggered ajaxStart. Started Request') })We have an Ajax request. Before sending the ... Read More
To Continue Learning Please Login