
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 1082 Articles for Go Programming
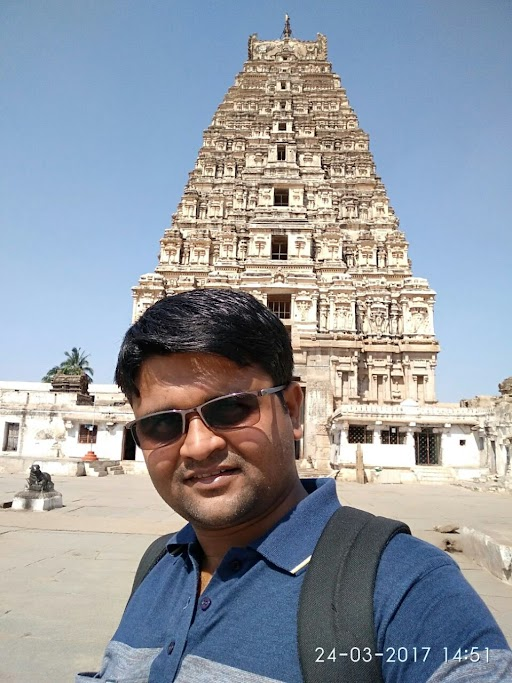
858 Views
Decorator function pattern is a pattern that is mainly found in Python and JavaScript, but we can also use it in Golang.It is a pattern in which we can add our own functionality over a current function by wrapping it. Since functions in Golang are considered firstclass objects, which in turn means that we can pass them as arguments as we would in the case of a variable.Example 1Let's start with a very simple example to understand the basic case of passing a function as an argument to an already existing function.Consider the code shown below.package main import ( ... Read More
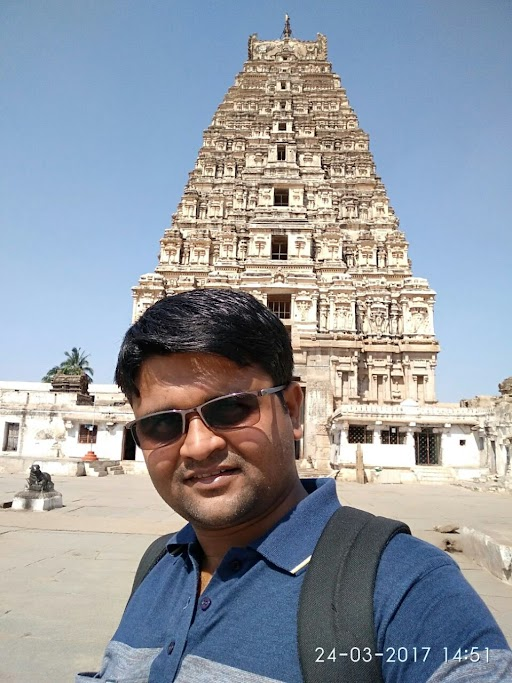
605 Views
Golang provides us with different approaches that we can use to print custom errors. We will explore two such approaches in this article.The first approach requires us to make use of the error.New() function which will create a new error, and we can even pass a string of our choice inside it as an argument.Example 1Consider the code shown below.package main import ( "errors" "fmt" "math" ) func areaOfCircle(radius float64) (float64, error) { if radius < 0 { return 0, errors.New("Area calculation wrong, the radius is < zero") } ... Read More
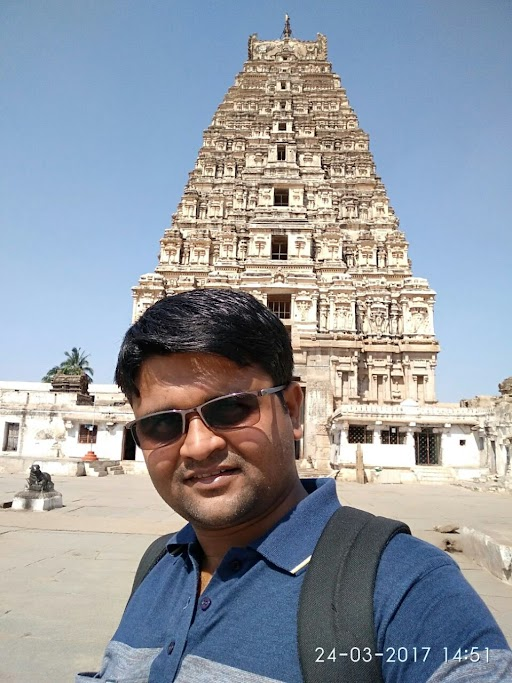
1K+ Views
We can make use of channels if we want to synchronize goroutines. By synchronizing, we want to make the goroutines work in a defined manner, for example, not starting the next goroutine until the previous one has finished its execution.The channels help in achieving that, as they can be used to block the process and then can also be used to notify the second goroutine that the previous goroutine has finished its job.Example 1Let's consider a very basic example of channel synchronization where we will see how we can achieve it with the help of a buffered channel.Consider the code ... Read More
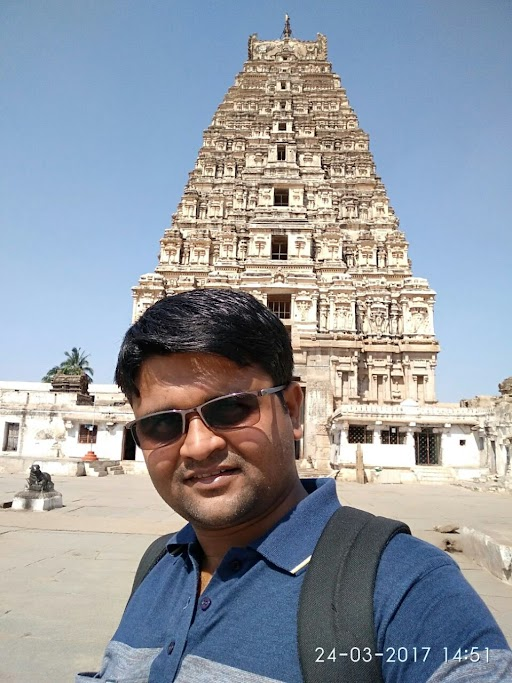
11K+ Views
Response status codes are the numbers that we get in response that signify what type of response we received from the server when we asked for something from it.There are different status codes that one gets from the response, and these are mainly divided into five categories.Generally, the status codes are divided into these five classes.1xx (Informational)2xx (Successful)3xx (Redirection)4xx (Client Error)5xx (Server Error)In this article, we will try to get two or more of these status codes.Example 1Let's start with a basic HTTP request to the google.com URL. Once we do that, we will get the response from the server ... Read More
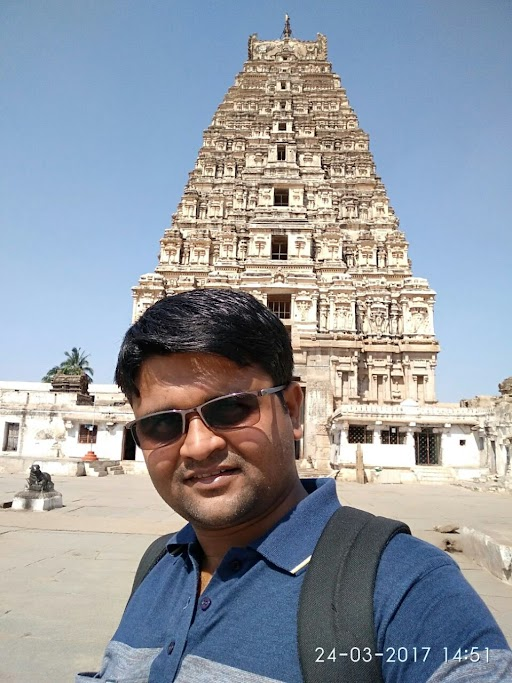
5K+ Views
Consider a case where we want to get the content type of a file in Golang for whatever reasons. In order to do that, we must first know how to open the file and read some of its bytes into a buffer slice which we will then pass to a function that will help us in detecting the type of the file.The first step is to open the file whose type we want to check.Open the FileConsider that we have a file named sample.pdf whose contentType is what we want to know. In order to open the file, we need ... Read More
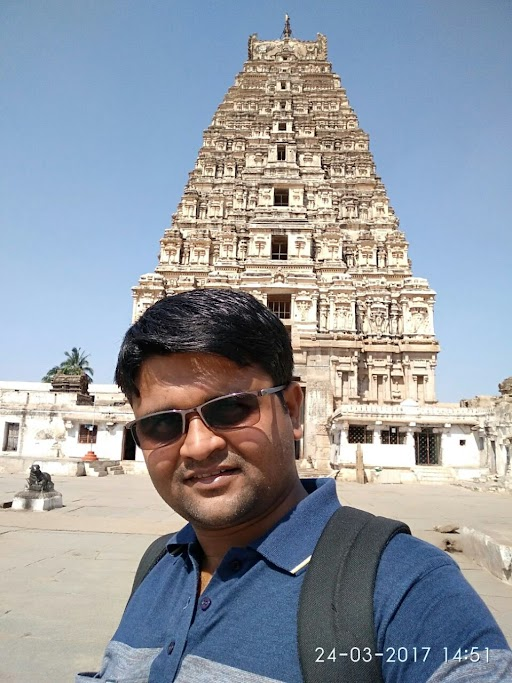
56K+ Views
To delete a key from a map, we can use Go's built-in delete() function. It should be noted that when we delete a key from a map, its value will also be deleted as the key-value pair is like a single entity when it comes to maps in Go.SyntaxThe syntax of the delete function is shown below.delete(map, key)Once we call the function in the above format, then the key from the map will be deleted.Now, let's use the above function in Go code and understand how it works.Example 1Consider the code shown belowpackage main import ( "fmt" ) ... Read More
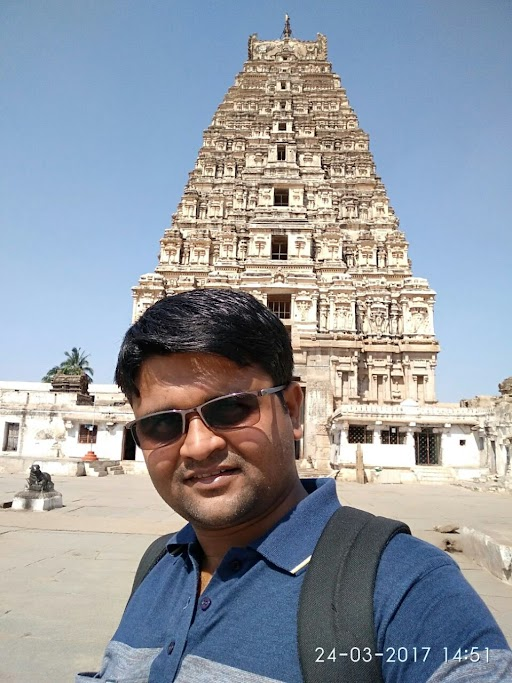
1K+ Views
Suppose we have a JSON that looks like this.{ "name":"Mukul Latiyan", "age":10, "sports":[ "football", "tennis", "cricket" ] }Now, we want to convert this JSON into struct fields which we can access later and maybe iterate over too.In order to do that, we need to first make a struct that will satisfy the fields of the above JSON.The struct shown below will work just fine for the above JSON.type Person struct ... Read More

269 Views
StepsTake a number from the user and store it in a variable.Pass the number as an argument to a recursive function.Define the base condition as the number to be lesser than 2.Otherwise, call the function recursively with the number minus 2.Then, return the result and check if the number is even or odd.Print the final result.Enter a number: 124Number is even!Enter a number: 567Number is odd!Example Live Demopackage main import ( "fmt" ) func check(n int) bool{ if n < 2 { return n % 2 == 0 } return check(n - 2) } ... Read More

168 Views
StepsDefine a recursive function.Define a base case for that function that the number should be greater than zero.If the number is greater than 0, call the function again with the argument as the number minus 1.Print the number.Enter the upper limit: 512345Enter the upper limit: 1512..15Example Live Demopackage main import ( "fmt" ) func printNo(number int){ if number >= 1{ printNo(number-1) fmt.Println(number) } } func main(){ var upper int fmt.Print("Enter the upper limit: ") fmt.Scanf("%d", &upper) printNo(upper) }OutputEnter the upper limit: 5 1 2 3 4 5

295 Views
StepsRead both the masses and the distance between the masses and store them in separate variables.Initialize one of the variables to the value of gravitational constant, G.Then, the formula f=(G*m1*m2)/(r**2) is used to determine the force acting between the masses.Rounding off up to two decimal places, print the value of the force.Enter the first mass: 1000000Enter the second mass: 500000Enter the distance between the centres of the masses: 20Hence, the gravitational force is: 0.08 NEnter the first mass: 90000000Enter the second mass: 7000000Enter the distance between the centres of the masses: 20Hence, the gravitational force is: 105.1 NExplanationUser must enter ... Read More
To Continue Learning Please Login