
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 1082 Articles for Go Programming

3K+ Views
Many languages do provide a method similar to indexOf() where one can find the existence of a particular element in an array-like data structure. However, in Golang, there's no such method and we can simply implement it with the help of a for-range loop.Let's suppose we have a slice of strings, and we want to find out whether a particular string exists in the slice or not.Example 1Consider the code shown below.package main import ( "fmt" ) func Contains(sl []string, name string) bool { for _, value := range sl { if value == name ... Read More

560 Views
Number parsing in Go is about converting the numbers that are present in string form to number form. By number form, we mean that these numbers can either be converted into integers, floats, etc.The most widely used package for number parsing is the "strconv" package that Go library provides us with. There are many cases that are present in the number parsing in Go, we will talk about all of these one by one in this article.The most basic approach is when we have a base 10 number that is actually present in a string form and we want to ... Read More

3K+ Views
In Go, we have a package named time that is present in Go's standard library. This time package contains different useful functions that we can use to calculate the time taken by a code block or even a function in Go.The most widely used functions are time.Sleep(), time.Since() and time.Now(). In this article, we will see how to use all these functions.Let's first consider a very basic example where we will use all these functions.Example 1Consider the code shown below.package main import ( "fmt" "time" ) func main() { fmt.Println("Measuring time in Go") start := time.Now() ... Read More

339 Views
In order to understand what closures are, we must know what anonymous functions are and how we can use them.Anonymous functionsIn Go, anonymous functions are those functions that don't have any name. Simply put, anonymous functions don't use any variables as a name when they are declared.We know that we declare a function with a similar syntax as shown below.func Sample(){ // some code }While we do have a name for the above function (Sample), in the case of anonymous functions, we don't have one.What is the closure function?Closure functions are the anonymous functions that have access to their ... Read More

3K+ Views
We know that every code in Golang is present inside a package, which can either be an executable one or a utility one. The executable package name is usually main and the name of the utility package can be anything, in most cases, it is the name of the folder.Suppose we have a directory structure that looks something like this.. |-- greet | `-- greet.go |-- sample | `-- sample.goWe have two directories, namely, greet and sample, and each of them contains a single .go file inside them. Now, we want to make use of a function that ... Read More

7K+ Views
To read a file into a string, we need to make use of the io/ioutil package that Go's standard library provides us with.Inside the io/ioutil package, there's a function called ReadFile() which is used to open a file and then convert its contents into a slice of bytes, and if for some reason, it isn't able to do so, then it will return an error too.Here is the syntax of the ReadLine() function.func ReadFile(filename string) ([]byte, error)It should be noted that if the above function has made a successful call, then it will return err == nil, not err == ... Read More

7K+ Views
Suppose we want to read a JSON file in Go. By reading, we mean that we want to convert the file data into a struct in Go.Consider the JSON file shown below that we will use to read and then convert the data into the structure.{ "users": [ { "name": "Mukul Latiyan", "social": { "facebook": "https://facebook.com/immukul", "twitter": "https://twitter.com/immukul", "linkedin": "https://linkedin.com/immukul" } }, ... Read More
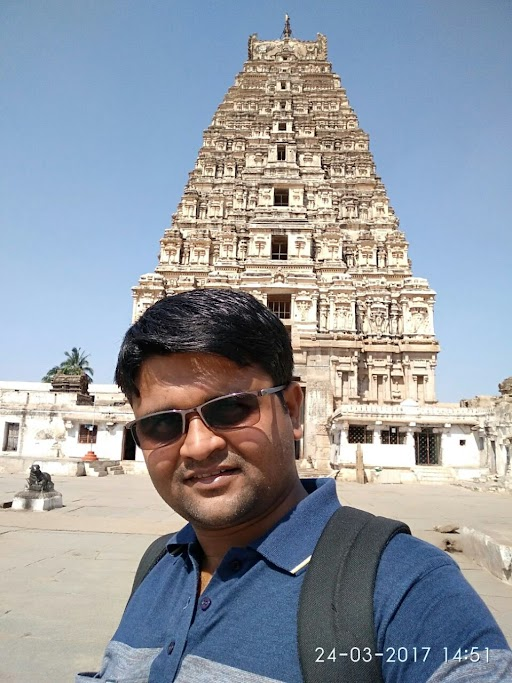
4K+ Views
Sometimes there are cases where we want to know which user is executing the current program and in which directory and we can get all these details with the help of the user package that is present inside the os package of Go's standard library.In this article, we will explore three such cases, where first, we will log out the username who is executing the current process and then we will log out the name along with the id and finally, we will log out from the directory in which the current program is located.Getting the UsernameTo get the username, ... Read More
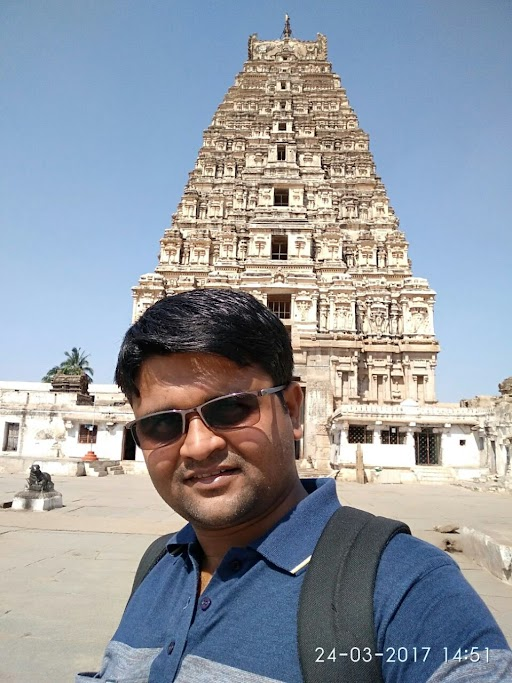
678 Views
In case we want to get the size of an array or a slice, we can make use of the built-in len() function that Go provides us with.Example 1Let's first explore how to use the len function with an array. Consider the code shown below.package main import ( "fmt" ) func main() { fmt.Println("Inside the main function") arr := [3]int{1, 2, 3} fmt.Println("The length of the array is:", len(arr)) }In the above code, we are using the len function on the array defined as arr.OutputIf we run the command go run main.go ... Read More
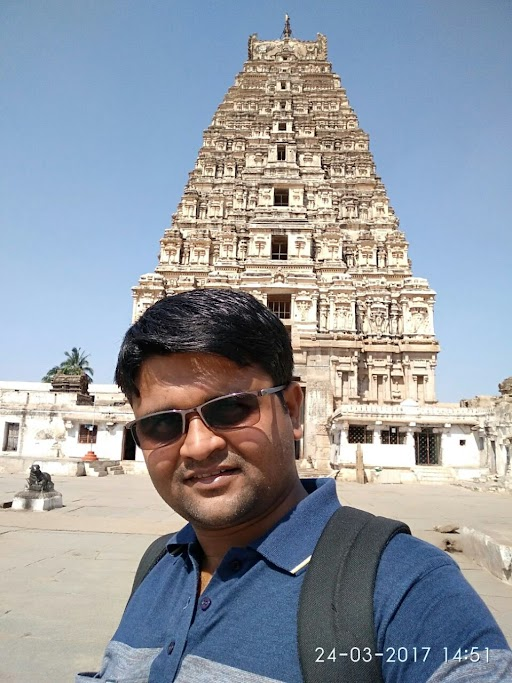
3K+ Views
We can close a channel in Golang with the help of the close() function. Once a channel is closed, we can't send data to it, though we can still read data from it. A closed channel denotes a case where we want to show that the work has been done on this channel, and there's no need for it to be open.We open the channel the moment we declare one using the make keyword.ch := make(chan int)Example 1Let's consider a very simple example, in which we will create a buffered channel and then pass data to it and then close ... Read More
To Continue Learning Please Login