
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 8907 Articles for Front End Technology

301 Views
We will learn how to add new array elements at the beginning of an array in JavaScript. To achieve this in JavaScript we have multiple ways, a few of them are following. Using the Array unshift() Method Using the Array splice() Method Using ES6 Spread Operator Using the Array unshift() Method The array unshift() method in JavaScript is used to add new elements at the start of the array. This method changes the original array by overwriting the elements inside it. Syntax arr.unshift(element1, element2, . . . . . . , elementN) Parameter element1, element2, …, ... Read More
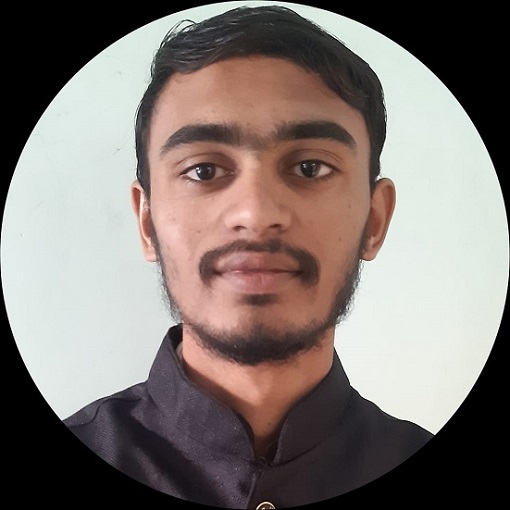
2K+ Views
In this tutorial, we shall learn to access the methods of an array of objects in JavaScript. What is an array of objects? An array of objects can store several values in a single variable. Every value has an array index. The values can be objects, booleans, functions, numbers, strings, or another array. Arrays are extremely flexible because they are listed as objects. Therefore, we can access it easily. An array of objects has a lot of built-in methods. Some are concat, forEach, join, indexOf, splice, sort, slice, lastIndexOf, filter, map, pop, shift, push, unshift, reverse, find, reduce, isArray, length, ... Read More

137 Views
The Array object lets you store multiple values in a single variable. It stores a fixed-size sequential collection of elements of the same type.Here is a list of some of the methods of the Array object:S.NoMethod & Description 1concat()Returns a new array comprised of this array joined with other array(s) and/or value(s). 2every()Returns true if every element in this array satisfies the provided testing function. 3filter()Creates a new array with all of the elements of this array for which the provided filtering function returns true. 4forEach()Calls a function for ... Read More
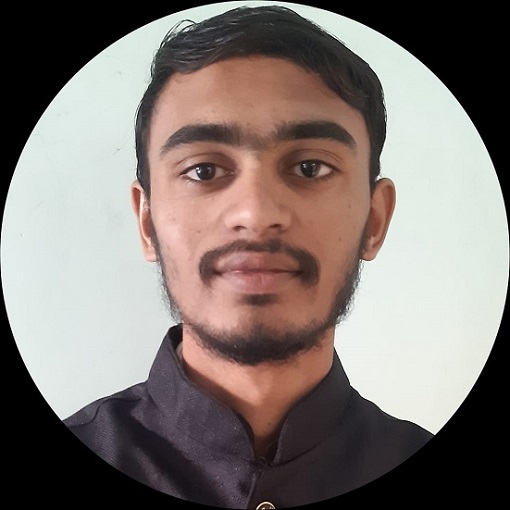
5K+ Views
In this tutorial, we will learn how to access the properties of an array of objects in JavaScript. JavaScript is built on a basic object-oriented paradigm. A collection of properties is an object, and property is a relationship between a name (or key) and a value. The value of a property can be a function, in which case the property is known as a method. A JavaScript object is associated with properties. An object's property may be thought of as a variable that is associated with the object. Except for the attachment to objects, object attributes are the same as ... Read More

9K+ Views
To create an array of strings in JavaScript, simply assign values −var animals = ["Time", "Money", "Work"];You can also use the new keyword to create an array of strings in JavaScript −var animals = new Array("Time", "Money", "Work");The Array parameter is a list of strings or integers. When you specify a single numeric parameter with the Array constructor, you specify the initial length of the array. The maximum length allowed for an array is 4, 294, 967, 295.ExampleLet’s see an example to create arrays in JavaScript −Live Demo JavaScript Arrays ... Read More

4K+ Views
The Array object lets you store multiple values in a single variable. It stores a fixed-size sequential collection of elements of the same type.The following is the list of the properties of the Array object −Sr.NoProperty & Description1constructorReturns a reference to the array function that created the object.2indexThe property represents the zero-based index of the match in the string.3inputThis property is only present in arrays created by regular expression matches.4lengthReflects the number of elements in an array.5prototypeThe prototype property allows you to add properties and methods to an object.ExampleLet’s see an example of the prototype propertyLive Demo ... Read More

14K+ Views
To create an array of integers in JavaScript, try the following −var rank = [1, 2, 3, 4];You can also use the new keyword to create array of integers in JavaScript −var rank = new Array(1, 2, 3, 4);The Array parameter is a list of strings or integers. When you specify a single numeric parameter with the Array constructor, you specify the initial length of the array. The maximum length allowed for an array is 4, 294, 967, 295.ExampleLet’s see an example to create an array of integers in JavaScript −Live Demo ... Read More

277 Views
To create an array in JavaScript, simply assign values −var animals = ["Dog", "Cat", "Tiger"];You can also use the new keyword to create arrays in JavaScript −var animals = new Array("Dog", "Cat", "Tiger");The Array parameter is a list of strings or integers. When you specify a single numeric parameter with the Array constructor, you specify the initial length of the array. The maximum length allowed for an array is 4, 294, 967, 295.ExampleLet’s see an example to create arrays in JavaScriptLive Demo JavaScript Arrays ... Read More

816 Views
The best and fastest way to concatenate strings in JavaScript is to use the + operator. You can also use the concat() method.ExampleYou can try to run the following code to concatenate strings in JavaScriptLive Demo JavaScript Concatenation var str1 = "Hello"; var str2 = "World"; var res = str1 + str2; document.write(res);
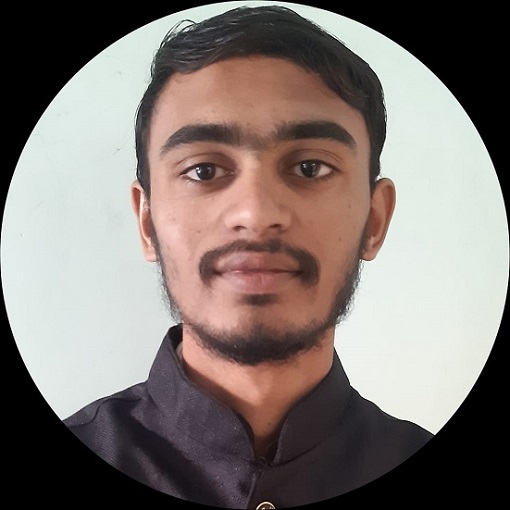
1K+ Views
It is often required to update the string and replace the single character at a particular index while programming with JavaScript. JavaScript doesn’t contain the built-in method to replace the single character at the particular index in the string as the Java and C++, but we can use some other built-in methods and create custom logic to solve our problem.In this tutorial, we have two methods to replace the character at a particular index in the string and update the string. One is by converting string to array, and another is using the substr() built-in method of JavaScript.Replace Character by ... Read More
To Continue Learning Please Login