
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 8907 Articles for Front End Technology

13K+ Views
In this tutorial, we will learn about the method of finding the length of an array in JavaScript. The length of an array is the number of elements or items contained by a JavaScript array. In JavaScript, we have only one way or method to find out the length of an array. Using the length property Using the length property In JavaScript, we are allowed to use the length property of JavaScript for finding the length of an array. As we know the length of an array is the number of elements contained by the array, the length ... Read More

65 Views
To create a zero-filled JavaScript array, use the Unit8Array typed array.ExampleYou can try to run the following code:Live Demo var arr1 = ["marketing", "technical", "finance", "sales"]; var arr2 = new Uint8Array(4); document.write(arr1); document.write("Zero filled array: "+arr2);

159 Views
To append an item to a JavaScript array, use the push() method.ExampleYou can try to run the following code to append an item:Live Demo var arr = ["marketing", "technical", "finance", "sales"]; arr.push("HR"); document.write(arr); Outputmarketing,technical,finance,sales,HR

858 Views
ECMAScript allows usage of const to define constants in JavaScript. To define integer constants in JavaScript, use the const,const MY_VAL = 5; // This will throw an error MY_VAL = 10;As shown above, MY_VAL is a constant and value 5 is assigned. On assigning, another value to a constant variable shows an error.Using const will not allow you to reassign any value to MY_VAL again. If you will assign a new value to a constant, then it would lead to an error.

8K+ Views
In JavaScript, we can check for particular class whether it is contained by an HTML element or not, in the HTML document. During the development of the web page, a developer uses many classes and sometimes assign similar classes to different elements which requires same styles in CSS. In this process, the developer may forget the class given to the particular element, then the developer may need check for the class inside the element using the JavaScript. In this tutorial, we are going to discuss how we can test if an element contains class in JavaScript. To check for the ... Read More
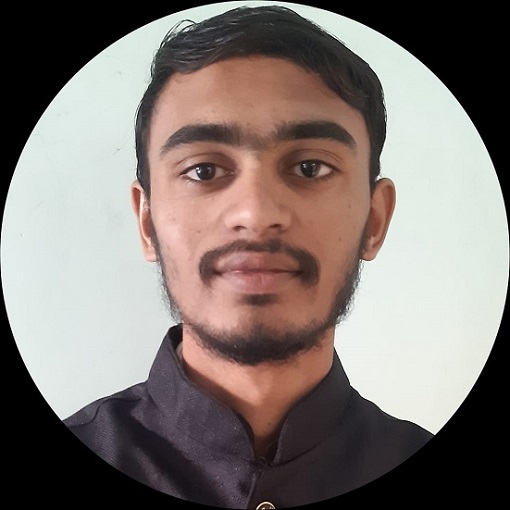
2K+ Views
In this tutorial, we will learn the methods to randomize or shuffle a JavaScript array. We can achieve this by using existing shuffle functions in some libraries or algorithms. Let’s move forward to discuss this. Using the Fisher-Yates Algorithm and Destructuring Assignment Here, the algorithm iterates through the array from the last index to the first index. In each looping, it swaps the array values and creates a random permutation of a finite sequence. We can follow the syntax below for using this algorithm. Syntax for (var i=arr.length – 1;i>0;i--) { var j = Math.floor(Math.random() * (i ... Read More
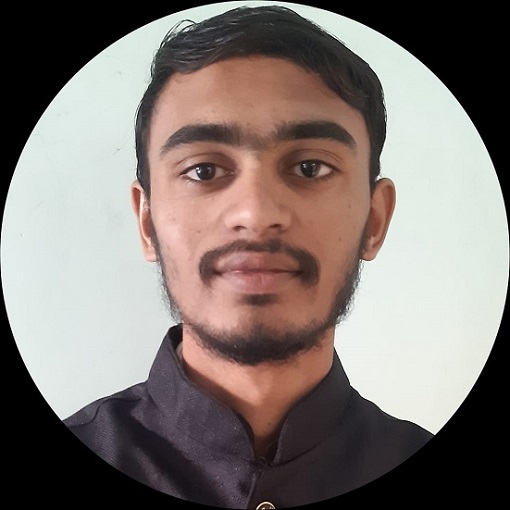
2K+ Views
In this tutorial, let’s find out how to extend an existing JavaScript array with another array. Before explaining the steps, we need to be aware that there is a requirement for several arrays at various times, and learning the right method can save our coding time. You will find more than four methods to extend an array with another. So, most likely, find an easy-to-remember method in the tutorial. Using Array push() Method and Spread Syntax The push() method is extremely useful in adding items to an existing JavaScript array. Moreover, you can pass a variety of different arguments based ... Read More
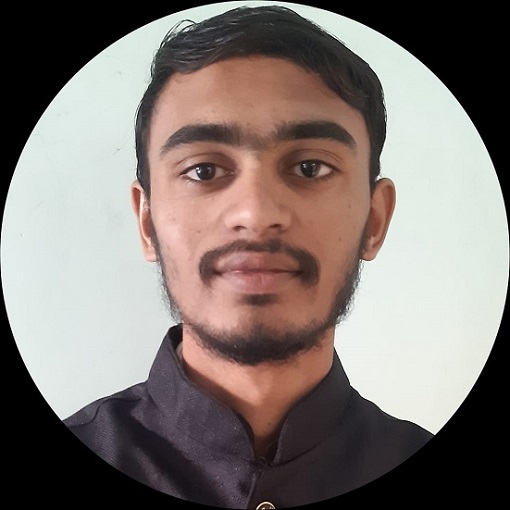
3K+ Views
In this tutorial, we will learn how to merge two arrays in JavaScript. In JavaScript, we can merge two arrays in different approaches, and in this tutorial, we will see some of the approaches for doing this − Using the Array concate() method Using the spread operator Using the loop iteration Using the Array concate() method The Array concate() method merges two or multiple arrays. It is one of the best method to merge arrays in JavaScript. This method operates on an array, takes another array in the parameters, and returns a new one after merging these ... Read More
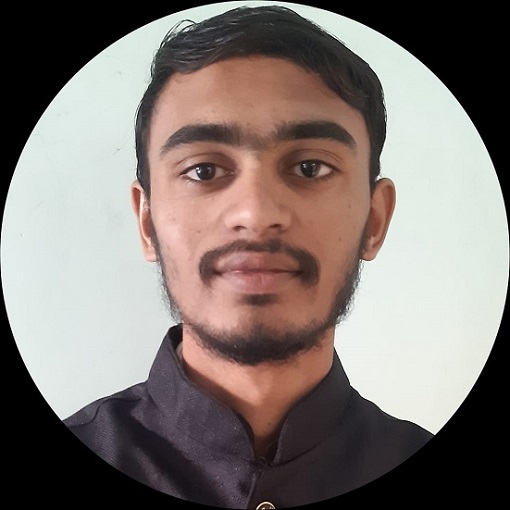
2K+ Views
In this tutorial, we will try to get the last element in an array using the following three approaches − Using Array length Property. Using the slice() Method. Using the pop() Method. Using Array length Property The length property of an array returns the number of elements in an array. The array index will start from 0, and the last index will total the length of array -1. For example, if the length of the array is 5 the indexing numbering will be 0 to 4. That means if we want to get the last element, the index ... Read More
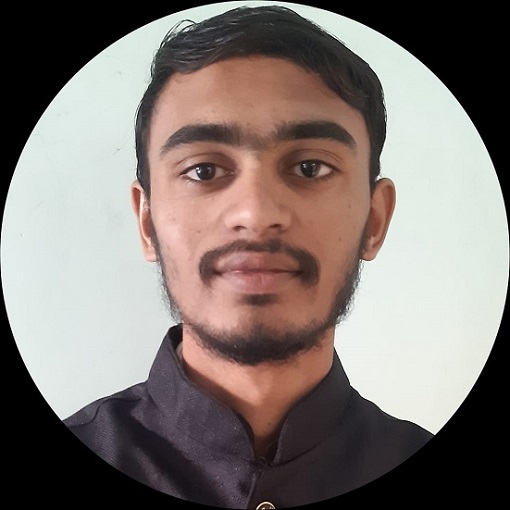
383 Views
In this tutorial, we will learn how to compare arrays in JavaScript using various methods. We will check whether each element present in one array is equal to the counterpart of the other array or not. If both the arrays are the same, then we will return true. Else, we will return false. When comparing two arrays, we cannot use the “==” or “===” operator because it will compare the addresses of the memory block to which both the arrays are pointing. Hence from this, we will get a false result. Now, we will see methods for comparing arrays in ... Read More
To Continue Learning Please Login