
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 35 Articles for ASP.Net

289 Views
Entity Framework is an ORM (object-relational mapper) framework that makes it easy to create, retrieve, update, or delete data from relational databases. Using Entity Framework, you can work with C# objects that abstract the database related code, so you rarely have to deal with raw SQL.The following figure illustrates where the Entity Framework fits into a layered architecture.Entity Framework Core (EF Core) is the new version of Entity Framework 6. Similar to .NET Core, EF Core is a lightweight, open-source, and cross-platform version of Entity Framework. It is developed to be used with .NET Core applications.To integrate EF Core into ... Read More

242 Views
The iterator pattern is used to loop over the elements in a collection and is implemented using the IEnumerator interface. It defines the basic low-level protocol through which elements in a collection are traversed—or enumerated. This is done in a forward-only manner.Here's the IEnumerator interface in C#.public interface IEnumerator{ bool MoveNext(); object Current { get; } void Reset(); }MoveNext advances the current element or "cursor" to the next position, returning false if there are no more elements in the collection.Current returns the element at the current position (usually cast from object to a more specific type). MoveNext ... Read More

809 Views
Working with JSONJSON is a data format that has become a popular alternative to XML. It is simple and uncluttered with a syntax similar to a JavaScript object. In fact, the term JSON stands for JavaScript Object Notation. The recent versions of .NET provide built-in support for working with JSON data.The System.Text.Json namespace provides high-performance, low-allocating features to process the JSON data. These features include serializing objects to JSON and deserializing JSON back into objects. It also provides types to create an in-memory document object model (DOM) for accessing any element within the JSON document, providing a structured view of ... Read More

471 Views
Assembly contains all the compiled types in your application along with their Intermediate Language (IL) code. It is also the basic unit of deployment in .NET. In the latest versions of .NET, i.e. .NET Core, an assembly is a file with a .dll extension, which stands for Dynamic Link Library.There are primarily four items in an assembly.Compiled TypesThe compiled IL code for all the types in your application.Assembly ManifestContains the metadata needed by the Common Language Runtime, such as the dependencies and versions that this DLL references.Its purpose is to describe the assembly to the runtime via the assembly's data, ... Read More

329 Views
The .NET streams architecture provides a consistent programming interface for reading and writing across different I/O types. It includes classes for manipulating files and directories on disk, as well as specialized streams for compression, named pipes, and memory-mapped files.The streaming architecture in .NET relies on a backing store and adapters.Backing StoreIt represents a store of data, such as a file or a network connection. It can act as either a source from which bytes can be read sequentially or a destination to which bytes can be written sequentially.The Stream class in .NET exposes the backing store to programmers. It exposes ... Read More

863 Views
Variables of value types directly contain the values. When you assign one value type variable to another, each variable associates with a different storage location in memory. Hence, Changing the value of one value type variable doesn't affect the value in the second variable.Similarly, when you pass an instance of a value type to a method, the compiler copies the memory associated with the argument to a new location associated with the parameter. Any changes to the parameter won't affect the original value. Since memory is copied for value types, they should be small (typically, less than 16 bytes).All the ... Read More

256 Views
A C# program compiles to a DLL assembly that contains the compiled C# code along with the metadata for the runtime, and other resources. C# provides a reflection API that lets us inspect the metadata and compiled code at runtime.Using reflection, it's possible to −Access the assembly metadata for all types inside the assemblyObtain a list of types and their members (methods, properties, etc.)Dynamically invoke the type members at runtime.Instantiate objects by only providing their nameBuild assembliesIn a traditional program, when you compile the source code to the machine code, the compiler removes all the metadata about the code. However, ... Read More

399 Views
Events are a simplified pattern that uses delegates. In C#, all delegates have the multicast capability, i.e., an instance of a delegate can represent not just a single method but a list of methods. For example −Exampledelegate int Transformer(int x); static void Main(){ Transformer perform = x =>{ int result = x * x; Console.WriteLine(result); return result; }; perform += x =>{ int result = x * x * x; Console.WriteLine(result); return result; }; perform(2); // prints ... Read More

4K+ Views
Both ASP and ASP.NET are the widely used languages for application and mainly the frontEnd development. Both the languages used for dynamic generation of web pages. The content generated through server-side scripting is then sent to the client’s web browser.Following are the important differences between ASP and ASP.NET.Sr. No.KeyASPASP.NET1DefinitionASP or also popularly known as Classic ASP developed by Microsoft is first Server-side scripting engine which is used for dynamic generation of web pages.ASP.NET, on the other hand, is a server-side web framework, open-source, which is designed for the generation of dynamic web pages.2Language typeASP is interpreted language that means the ... Read More
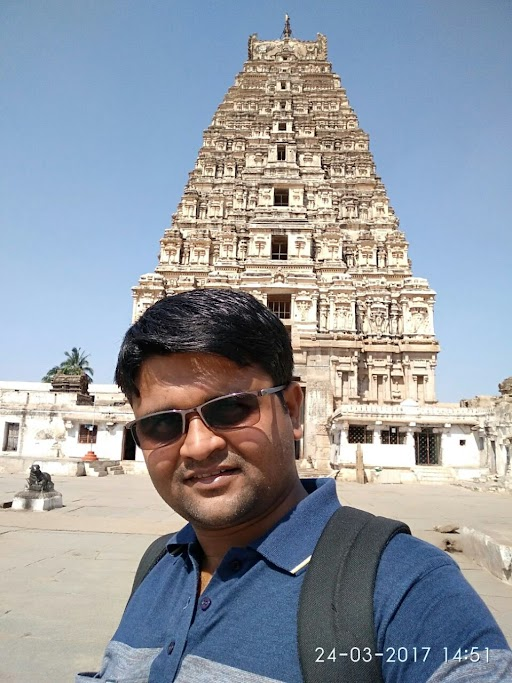
3K+ Views
JSP and ASP are both server-side scripting languages. JSP is Java based and is developed by Sun Microsystems, whereas ASP is developed by Microsoft and is also referred as Classic ASP. Whenever a browser requests a JSP or ASP page, the server engine reads the file, executes the code in file and returns the HTML output to the browser.JSP is compiled, whereas ASP is interpreted. ASP.NET is a .NET based variant of ASP where the codes are compiled to improve the performance.What is ASP?ASP is a server-side scripting engine, which means the code that is written gets sent to the ... Read More