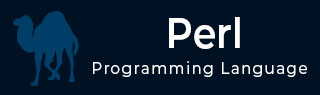
- Perl Basics
- Perl - Home
- Perl - Introduction
- Perl - Environment
- Perl - Syntax Overview
- Perl - Data Types
- Perl - Variables
- Perl - Scalars
- Perl - Arrays
- Perl - Hashes
- Perl - IF...ELSE
- Perl - Loops
- Perl - Operators
- Perl - Date & Time
- Perl - Subroutines
- Perl - References
- Perl - Formats
- Perl - File I/O
- Perl - Directories
- Perl - Error Handling
- Perl - Special Variables
- Perl - Coding Standard
- Perl - Regular Expressions
- Perl - Sending Email
- Perl Advanced
- Perl - Socket Programming
- Perl - Object Oriented
- Perl - Database Access
- Perl - CGI Programming
- Perl - Packages & Modules
- Perl - Process Management
- Perl - Embedded Documentation
- Perl - Functions References
- Perl Useful Resources
- Perl - Questions and Answers
- Perl - Quick Guide
- Perl - Useful Resources
- Perl - Discussion
Perl open Function
Description
This function opens a file using the specified file handle. The file handle may be an expression, the resulting value is used as the handle. If no filename is specified a variable with the same name as the file handle used (this should be a scalar variable with a string value referring to the file name). The special file name '-' refers to STDIN and '>-' refers to STDOUT.
Syntax
Following is the simple syntax for this function −
open FILEHANDLE, EXPR, LIST open FILEHANDLE, EXPR open FILEHANDLE
Return Value
This function returns 0 on failure and 1 on success.
Example
Following is the syntax to open file.txt in read-only mode. Here less than < sign indicates that file has to be opend in read-only mode.
open(DATA, "<file.txt");
Here DATA is the file handle which will be used to read the file. Here is the example which will open a file and will print its content over the screen.
#!/usr/bin/perl open(DATA, "<file.txt"); while(<DATA>) { print "$_"; }
Following is the syntax to open file.txt in writing mode. Here less than > sign indicates that file has to be opend in writing mode −
open(DATA, ">file.txt");
This example actually truncates (empties) the file before opening it for writing, which may not be the desired effect. If you want to open a file for reading and writing, you can put a plus sign before the > or < characters.
For example, to open a file for updating without truncating it −
open(DATA, "+<file.txt");
To truncate the file first −
open DATA, "+>file.txt" or die "Couldn't open file file.txt, $!";
You can open a file in append mode. In this mode writing point will be set to the end of the file.
open(DATA,">>file.txt") || die "Couldn't open file file.txt, $!";
A double >> opens the file for appending, placing the file pointer at the end, so that you can immediately start appending information. However, you can.t read from it unless you also place a plus sign in front of it −
open(DATA,"+>>file.txt") || die "Couldn't open file file.txt, $!";
Following is the table which gives the possible values of different modes.
Entities Definition < or r Read Only Access > or w Creates, Writes, and Truncates >> or a Writes, Appends, and Creates +< or r+ Reads and Writes +> or w+ Reads, Writes, Creates, and Truncates +>> or a+ Reads, Writes, Appends, and Creates
To Continue Learning Please Login