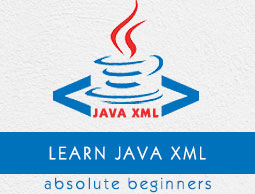
- Javax.xml.bind classes
- Home
- Binder
- DatatypeConverter
- JAXB
- JAXBContext
- JAXBElement
- JAXBElement.GlobalScope
- JAXBIntrospector
- Marshaller.Listener
- SchemaOutputResolver
- Unmarshaller.Listener
- Javax.xml.bind.util classes
- JAXBResult
- JAXBSource
- ValidationEventCollector
- Javax.xml.parsers classes
- DocumentBuilder
- DocumentBuilderFactory
- SAXParser
- SAXParserFactory
- Javax.xml.soap classes
- AttachmentPart
- MessageFactory
- MimeHeader
- MimeHeaders
- SAAJMetaFactory
- SOAPConnection
- SOAPConnectionFactory
- SOAPFactory
- SOAPMessage
- SOAPPart
- Javax.xml.validation classes
- Schema
- SchemaFactory
- TypeInfoProvider
- Validator
- ValidatorHandler
- Javax.xml.xpath classes
- XPathConstants
- XPathFactory
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
String[] getHeader(String name) Method
Description
The javax.xml.soap.MimeHeaders.getHeader(String name) method returns all of the values for the specified header as an array of String objects.
Declaration
Following is the declaration for javax.xml.soap.MimeHeaders.getHeader(String name) method
String[] getHeader(String name)
Parameters
name − a String with the name of the header to be retrieved.
Return Value
a String array with all of the values for the specified header.
Example
The following example shows the usage of javax.xml.soap.MimeHeaders.getHeader(String name) method.
package com.tutorialspoint; import java.io.ByteArrayInputStream; import javax.xml.soap.MessageFactory; import javax.xml.soap.MimeHeaders; import javax.xml.soap.SOAPConstants; import javax.xml.soap.SOAPMessage; public class MimeHeadersDemo { public static void main(String[] args) { try { //create a default message factory MessageFactory messageFactory = MessageFactory.newInstance( SOAPConstants.SOAP_1_2_PROTOCOL); String contents = "Hello World!"; // add raw content ByteArrayInputStream bis = new ByteArrayInputStream( contents.getBytes()); MimeHeaders headers = new MimeHeaders(); // add a MIME header headers.addHeader("Content-Type", "text/xml"); // create a new SOAPMessage SOAPMessage message = MessageFactory .newInstance() .createMessage(headers,bis); String[] headerContent = message.getMimeHeaders() .getHeader("Content-Type"); System.out.println("Mime Header Value: " + headerContent[0]); } catch (Exception ex) { ex.printStackTrace(); } } }
If we compile the code and execute it, this will produce the following result −
Mime Header Value: text/xml
javax_xml_soap_mimeheaders.htm
Advertisements
To Continue Learning Please Login