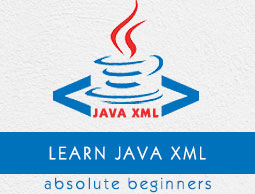
- Javax.xml.bind classes
- Home
- Binder
- DatatypeConverter
- JAXB
- JAXBContext
- JAXBElement
- JAXBElement.GlobalScope
- JAXBIntrospector
- Marshaller.Listener
- SchemaOutputResolver
- Unmarshaller.Listener
- Javax.xml.bind.util classes
- JAXBResult
- JAXBSource
- ValidationEventCollector
- Javax.xml.parsers classes
- DocumentBuilder
- DocumentBuilderFactory
- SAXParser
- SAXParserFactory
- Javax.xml.soap classes
- AttachmentPart
- MessageFactory
- MimeHeader
- MimeHeaders
- SAAJMetaFactory
- SOAPConnection
- SOAPConnectionFactory
- SOAPFactory
- SOAPMessage
- SOAPPart
- Javax.xml.validation classes
- Schema
- SchemaFactory
- TypeInfoProvider
- Validator
- ValidatorHandler
- Javax.xml.xpath classes
- XPathConstants
- XPathFactory
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
Iterator getAllHeaders() Method
Description
The javax.xml.soap.MimeHeaders.getAllHeaders() method adds a MimeHeader object with the specified name and value to this MimeHeaders object's list of headers.
Declaration
Following is the declaration for javax.xml.soap.MimeHeaders.getAllHeaders() method
void addHeader(String name, String value)
Parameters
name − a String with the name of the header to be added.
value − a String with the value of the header to be added.
Return Value
No return value
Exception
IllegalArgumentException − if there was a problem in the mime header name or value being added.
Example
The following example shows the usage of javax.xml.soap.MimeHeaders.addHeader(String name, String value) method.
package com.tutorialspoint; import java.io.ByteArrayInputStream; import java.util.Iterator; import javax.xml.soap.MessageFactory; import javax.xml.soap.MimeHeader; import javax.xml.soap.MimeHeaders; import javax.xml.soap.SOAPConstants; import javax.xml.soap.SOAPMessage; public class MimeHeadersDemo { public static void main(String[] args) { try { //create a default message factory MessageFactory messageFactory = MessageFactory.newInstance( SOAPConstants.SOAP_1_2_PROTOCOL); String contents = "Hello World!"; // add raw content ByteArrayInputStream bis = new ByteArrayInputStream( contents.getBytes()); MimeHeaders headers = new MimeHeaders(); // add a MIME header headers.addHeader("Content-Type", "text/xml"); // create a new SOAPMessage SOAPMessage message = MessageFactory .newInstance() .createMessage(headers,bis); Iterator<MimeHeader> iterator = message.getMimeHeaders().getAllHeaders(); while (iterator.hasNext()) { MimeHeader header = iterator.next(); System.out.println("Mime Header Name: " + header.getName()); System.out.println("Mime Header value: " + header.getValue()); } } catch (Exception ex) { ex.printStackTrace(); } } }
If we compile the code and execute it, this will produce the following result −
Mime Header Name: Content-Type Mime Header value: text/xml
javax_xml_soap_mimeheaders.htm
Advertisements
To Continue Learning Please Login