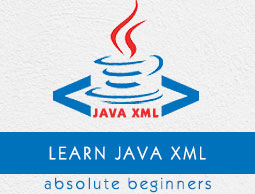
- Javax.xml.bind classes
- Home
- Binder
- DatatypeConverter
- JAXB
- JAXBContext
- JAXBElement
- JAXBElement.GlobalScope
- JAXBIntrospector
- Marshaller.Listener
- SchemaOutputResolver
- Unmarshaller.Listener
- Javax.xml.bind.util classes
- JAXBResult
- JAXBSource
- ValidationEventCollector
- Javax.xml.parsers classes
- DocumentBuilder
- DocumentBuilderFactory
- SAXParser
- SAXParserFactory
- Javax.xml.soap classes
- AttachmentPart
- MessageFactory
- MimeHeader
- MimeHeaders
- SAAJMetaFactory
- SOAPConnection
- SOAPConnectionFactory
- SOAPFactory
- SOAPMessage
- SOAPPart
- Javax.xml.validation classes
- Schema
- SchemaFactory
- TypeInfoProvider
- Validator
- ValidatorHandler
- Javax.xml.xpath classes
- XPathConstants
- XPathFactory
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
Javax.xml.parsers.SAXParser.parse() Method
Description
The Javax.xml.parsers.SAXParser.parse(InputStream is, DefaultHandler dh) method parses the content InputStream instance as XML using the specified DefaultHandler.
Declaration
Following is the declaration for Javax.xml.parsers.SAXParser.parse() method
public void parse(InputStream is, DefaultHandler dh)
Parameters
is − The InputStream containing the content to be parsed.
dh − The SAX DefaultHandler to use.
Return Value
This method does not return a value.
Exception
IllegalArgumentException − If the InputStream object is null
IOException − If any IO errors occur.
SAXException − If any SAX errors occur during processing.
Example
For our examples to work, a xml file named Student.xml is needed in our CLASSPATH. The contents of this XML are the following −
<?xml version = "1.0" encoding = "UTF-8" standalone = "yes"?> <student id = "10"> <age>12</age> <name>Malik</name> </student>
The following example shows the usage of Javax.xml.parsers.SAXParser.parse() method.
package com.tutorialspoint; import java.io.FileInputStream; import javax.xml.parsers.SAXParser; import javax.xml.parsers.SAXParserFactory; import org.xml.sax.helpers.DefaultHandler; public class SaxParserDemo { public static void main(String[] args) { // create a new SAXParserFactory SAXParserFactory factory = SAXParserFactory.newInstance(); // create a new DefaultHandler DefaultHandler handler = new DefaultHandler() { // define what the parser does when it finds a character public void characters(char[] ch, int start, int length) { // print the characters System.out.println("" + new String(ch, start, length)); } }; try { // create a new inputstream for our XML FileInputStream is = new FileInputStream("Student.xml"); // get a new SAXParser SAXParser parser = factory.newSAXParser(); // parse the file parser.parse(is, handler); } catch (Exception ex) { ex.printStackTrace(); } } }
If we compile the code and execute it, this will produce the following result −
12 Malik
To Continue Learning Please Login