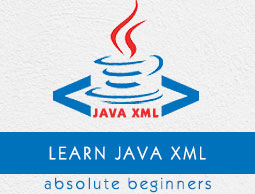
- Javax.xml.bind classes
- Home
- Binder
- DatatypeConverter
- JAXB
- JAXBContext
- JAXBElement
- JAXBElement.GlobalScope
- JAXBIntrospector
- Marshaller.Listener
- SchemaOutputResolver
- Unmarshaller.Listener
- Javax.xml.bind.util classes
- JAXBResult
- JAXBSource
- ValidationEventCollector
- Javax.xml.parsers classes
- DocumentBuilder
- DocumentBuilderFactory
- SAXParser
- SAXParserFactory
- Javax.xml.soap classes
- AttachmentPart
- MessageFactory
- MimeHeader
- MimeHeaders
- SAAJMetaFactory
- SOAPConnection
- SOAPConnectionFactory
- SOAPFactory
- SOAPMessage
- SOAPPart
- Javax.xml.validation classes
- Schema
- SchemaFactory
- TypeInfoProvider
- Validator
- ValidatorHandler
- Javax.xml.xpath classes
- XPathConstants
- XPathFactory
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
Javax.xml.parsers.SAXParser.getSchema() Method
Description
The Javax.xml.parsers.SAXParser.getSchema() method gets a reference to the the Schema being used by the XML processor. If no schema is being used, null is returned.
Declaration
Following is the declaration for Javax.xml.parsers.SAXParser.getSchema() method
public Schema getSchema()
Parameters
NA
Return Value
This method returns the Schema being used or null if none in use
Exception
UnsupportedOperationException − When implementation does not override this method
Example
The schema set in this example is a file called Student.xsd and is in our CLASSPATH. The contents of this file are the following −
<?xml version = "1.0" encoding = "utf-8"?> <xs:schema attributeFormDefault = "unqualified" elementFormDefault = "qualified" xmlns:xs = "http://www.w3.org/2001/XMLSchema"> <xs:element name = "student"> <xs:complexType> <xs:sequence> <xs:element name = "age" type = "xs:unsignedByte" /> <xs:element name = "name" type = "xs:string" /> </xs:sequence> <xs:attribute name="id" type="xs:unsignedByte" use = "required" /> </xs:complexType> </xs:element> </xs:schema>
The following example shows the usage of Javax.xml.parsers.SAXParser.getSchema() method.
package com.tutorialspoint; import java.io.File; import javax.xml.parsers.SAXParser; import javax.xml.parsers.SAXParserFactory; import javax.xml.validation.Schema; import javax.xml.validation.SchemaFactory; import static javax.xml.XMLConstants.*; public class SaxParserDemo { public static void main(String[] args) { // create a new SAXParserFactory SAXParserFactory factory = SAXParserFactory.newInstance(); // create a new schema factory SchemaFactory schemaFactory = SchemaFactory.newInstance(W3C_XML_SCHEMA_NS_URI); // a file that points to our schema File file = new File("Student.xsd"); try { // create a new schema Schema schema = schemaFactory.newSchema(file); // set our schema for our parser factory.setSchema(schema); // get a new SAXParser SAXParser parser = factory.newSAXParser(); // get the schema System.out.println("" + parser.getSchema()); } catch (Exception ex) { ex.printStackTrace(); } } }
If we compile the code and execute it, this will produce the following result −
com.sun.org.apache.xerces.internal.jaxp.validation.SimpleXMLSchema@4447393f
javax_xml_parsers_saxparser.htm
Advertisements
To Continue Learning Please Login