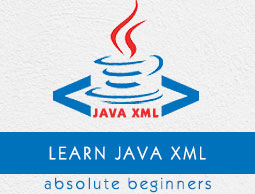
- Javax.xml.bind classes
- Home
- Binder
- DatatypeConverter
- JAXB
- JAXBContext
- JAXBElement
- JAXBElement.GlobalScope
- JAXBIntrospector
- Marshaller.Listener
- SchemaOutputResolver
- Unmarshaller.Listener
- Javax.xml.bind.util classes
- JAXBResult
- JAXBSource
- ValidationEventCollector
- Javax.xml.parsers classes
- DocumentBuilder
- DocumentBuilderFactory
- SAXParser
- SAXParserFactory
- Javax.xml.soap classes
- AttachmentPart
- MessageFactory
- MimeHeader
- MimeHeaders
- SAAJMetaFactory
- SOAPConnection
- SOAPConnectionFactory
- SOAPFactory
- SOAPMessage
- SOAPPart
- Javax.xml.validation classes
- Schema
- SchemaFactory
- TypeInfoProvider
- Validator
- ValidatorHandler
- Javax.xml.xpath classes
- XPathConstants
- XPathFactory
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
Java Xml Bind DatatypeConverter.parseInt() Method
Description
The Java.xml.bind.DatatypeConverter.parseInt(String lexicalXSDInt) method converts the string argument into an int value.
Declaration
Following is the declaration for java.xml.bind.DatatypeConverter.parseInt(String lexicalXSDInt) method −
public static int parseInt(String lexicalXSDInt)
Parameters
lexicalXSDInt − A string containing a lexical representation of xsd:int.
Return Value
A int value represented by the string argument.
Exception
NumberFormatException − lexicalXSDInt is not a valid string representation of an int value.
Example
The following example shows the usage of java.xml.bind.DatatypeConverter.parseInt(String lexicalXSDInt) method. To proceed, consider the following student.xsd file which contains the student xml definations.
<xsd:schema xmlns:xsd = "http://www.w3.org/2001/XMLSchema" xmlns:jxb = "http://java.sun.com/xml/ns/jaxb" jxb:version = "1.0"> <xsd:annotation> <xsd:documentation></xsd:documentation> <xsd:appinfo> <jxb:globalBindings fixedAttributeAsConstantProperty="false" collectionType = "java.util.Vector" typesafeEnumBase = "xsd:NCName" choiceContentProperty = "false" typesafeEnumMemberName = "generateError" enableFailFastCheck = "false" generateIsSetMethod = "false" underscoreBinding = "asCharInWord"> </jxb:globalBindings> <jxb:schemaBindings> <jxb:package name = "com.tutorialspoint"> <jxb:javadoc> <![CDATA[ <body>Package level documentation for generated package com.tutorialspoint.</body> ]]> </jxb:javadoc> </jxb:package> <jxb:nameXmlTransform> <jxb:elementName suffix = "Element"/> </jxb:nameXmlTransform> </jxb:schemaBindings> </xsd:appinfo> </xsd:annotation> <xsd:element name = "student" type = "StudentType"/> <xsd:complexType name = "StudentType"> <xsd:annotation> <xsd:appinfo> <jxb:class name = "Student"> <jxb:javadoc>A <b>Student</b> consists of details of a student.</jxb:javadoc> </jxb:class> </xsd:appinfo> </xsd:annotation> <xsd:sequence> <xsd:element name = "name" type = "xsd:string" /> <xsd:element name = "age" minOccurs = "0" type = "xsd:positiveInteger"> <xsd:annotation> <xsd:appinfo> <jxb:property> <jxb:baseType> <jxb:javaType name = "int" parseMethod = "javax.xml.bind.DatatypeConverter.parseInt" printMethod = "javax.xml.bind.DatatypeConverter.printInt"/> </jxb:baseType> </jxb:property> </xsd:appinfo> </xsd:annotation> </xsd:element> <xsd:element name = "birthdate" minOccurs = "0" type = "xsd:date"/> </xsd:sequence> <xsd:attribute name = "id" type = "xsd:positiveInteger"/> </xsd:complexType> </xsd:schema>
Now let us create main class which will be used to unmarshal ie. convert Student XML file to JAXB object. Here we will use DatatypeConverter parseInt method which will overrides the default JAXB binding of type java.math.BigInteger to int.This example unmarshals the Student object and prints.
package datatypeconverter; import com.tutorialspoint.Student; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.util.logging.Level; import java.util.logging.Logger; import javax.xml.bind.JAXBContext; import javax.xml.bind.JAXBElement; import javax.xml.bind.JAXBException; import javax.xml.bind.Unmarshaller; public class Main { public static void main(String[] args) throws FileNotFoundException{ // create a JAXBContext capable of handling classes generated into // the com.tutorialspoint package JAXBContext jc; try { jc = JAXBContext.newInstance("com.tutorialspoint"); // create an Unmarshaller Unmarshaller u = jc.createUnmarshaller(); // unmarshal a student instance document into a tree of Java content // objects composed of classes from the com.tutorialspoint package. JAXBElement studentElement = (JAXBElement) u.unmarshal( new FileInputStream("student.xml")); Student student = (Student)studentElement.getValue(); int age = student.getAge(); System.out.println("Age: " + age); } catch (JAXBException ex) { Logger.getLogger(Main.class.getName()).log(Level.SEVERE, null, ex); } } }
To create document, an XML file is needed as input. The XML file is named as student.xml −
<student id = "1"> <name>Robert</name> <age>20</age> <birthdate>1992-01-19</birthdate> </student>
Let us compile and run the above program, this will produce the following result −
Age: 20
To Continue Learning Please Login